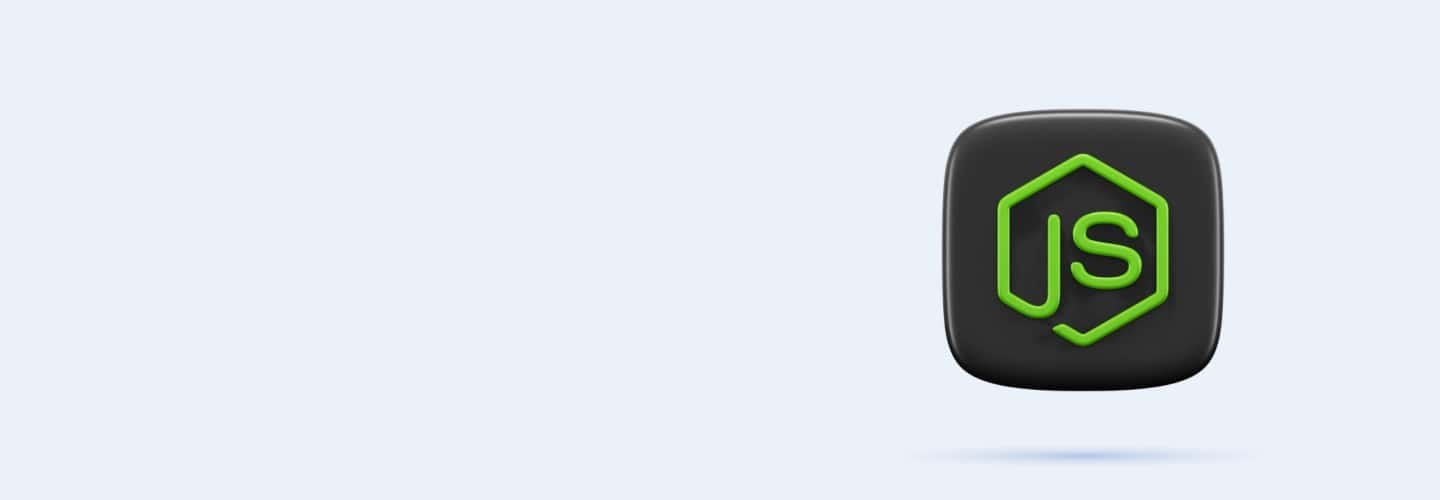
Q31
Q31 An application crashes with "Error: ENOENT, no such file or directory".
What should you check?
Disk space
File path
Node.js version
Network connection
Q32
Q32 What is the role of the event loop in Node.js?
To execute synchronous code
To manage I/O operations
To enhance CSS
To handle database queries
Q33
Q33 Which phase of the event loop executes timers scheduled by setTimeout and setInterval?
Poll phase
Check phase
Timers phase
Close callbacks phase
Q34
Q34 What happens during the 'poll' phase of the event loop?
Execution of callbacks
Processing of events
Execution of expired timers
Execution of I/O callbacks
Q35
Q35 Which phase of the event loop is responsible for executing callbacks from setImmediate()?
Poll phase
Check phase
Timers phase
Immediate phase
Q36
Q36 What is the primary benefit of Node.js's event-driven architecture?
Improved security
Ease of use
Scalability
Compatibility with other languages
Q37
Q37 Which method is used to defer the execution of a function until the next iteration of the event loop?
setTimeout
setInterval
setImmediate
nextTick
Q38
Q38 What is the output of the following code:
console.log('1'); process.nextTick(() => console.log('2')); console.log('3');
1 2 3
1 3 2
2 1 3
3 2 1
Q39
Q39 What is the output of the following code:
setTimeout(() => console.log('1'), 0); setImmediate(() => console.log('2'));
1 2
2 1
1
2
Q40
Q40 How can you monitor the phases of the event loop in a Node.js application?
Using console.log()
Using a debugger
Using process.nextTick()
Using event listeners
Q41
Q41 An application experiences high CPU usage and slow performance.
Which phase of the event loop might be causing this issue?
Poll phase
Timers phase
Close callbacks phase
Check phase
Q42
Q42 What is a stream in Node.js?
A way to handle I/O operations
A method for handling HTTP requests
A library for file manipulation
A type of database
Q43
Q43 Which of the following is a type of stream in Node.js?
Readable
Writable
Duplex
All of these
Q44
Q44 What is a buffer in Node.js?
A temporary storage for data
A method to read files
A HTTP handler
A type of stream
Q45
Q45 How do you create a buffer of 10 bytes in Node.js?
Buffer.alloc(10)
Buffer.create(10)
new Buffer(10)
Buffer.init(10)
Q46
Q46 What is the difference between Buffer.alloc and Buffer.allocUnsafe?
Buffer.alloc initializes the buffer with zeros
Buffer.allocUnsafe initializes the buffer with zeros
Buffer.alloc is deprecated
Buffer.allocUnsafe is deprecated
Q47
Q47 How do you create a readable stream in Node.js?
new stream.Readable()
fs.createReadStream()
stream.createRead()
new stream.Read()
Q48
Q48 How can you handle data events in a readable stream?
stream.on('data', callback)
stream.read(callback)
stream.write('data', callback)
stream.handle('data', callback)
Q49
Q49 What is the output of the following code:
const buf = Buffer.from('hello'); console.log(buf.toString('hex'));
68656c6c6f
hello
68656C6C6F
5a5a5a5a5a
Q50
Q50 What should you check if a readable stream is not emitting data?
Stream state
File permissions
Network connection
Buffer size
Q51
Q51 How can you handle errors in a stream?
Using a try-catch block
Using the error event
Restarting the stream
Ignoring the errors
Q52
Q52 What is the primary purpose of the HTTP module in Node.js?
To handle HTTP requests and responses
To handle file operations
To manage streams
To manage buffers
Q53
Q53 Which method is used to create a server in Node.js using the HTTP module?
http.createServer()
http.initServer()
http.newServer()
http.startServer()
Q54
Q54 Which event is emitted when the HTTP server receives a request?
request
data
connection
receive
Q55
Q55 What does the response.end() method do in the HTTP module?
Sends headers
Ends the response
Sends data
Closes the server
Q56
Q56 How can you set the response HTTP status code in Node.js?
response.setStatus()
response.statusCode()
response.writeHead()
response.sendStatus()
Q57
Q57 How do you write "Hello, World!" to the response in Node.js?
response.send('Hello, World!')
response.write('Hello, World!')
response.end('Hello, World!')
response.print('Hello, World!')
Q58
Q58 How do you read the request headers in Node.js?
request.headers
request.getHeaders()
request.readHeaders()
request.httpHeaders()
Q59
Q59 What is the output of the following code snippet?
const server = http.createServer((req, res) => { res.writeHead(200, {'Content-Type': 'text/plain'}); res.end('OK'); });
Content-Type
200 OK
plain text
OK
Q60
Q60 What should you check if an HTTP server in Node.js is not responding?
Server status
Network connection
Code syntax
HTTP version