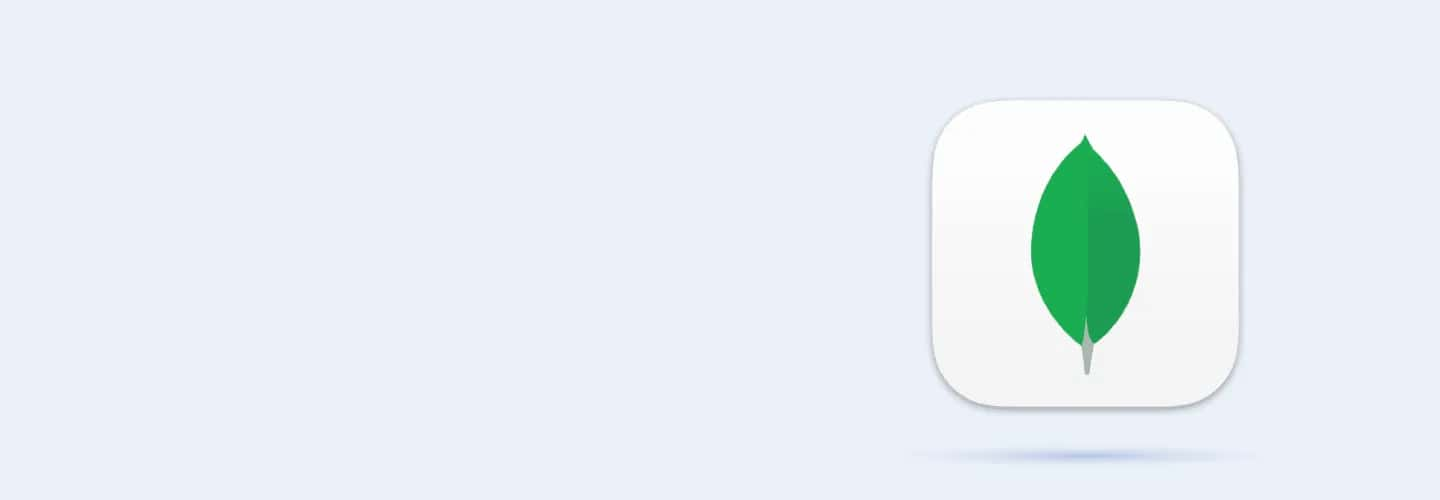
Q61
Q61 How does the $not operator function in MongoDB?
It negates the query condition specified
It selects documents that do not contain the field
It replaces the value of a field with null if the condition is true
It checks for values not equal to the specified value
Q62
Q62 What is the role of the $elemMatch operator in MongoDB queries?
To match documents where at least one element in an array matches the specified query conditions
To find the first element in an array that matches a condition
To apply a match condition to each element of an array
To update elements in an array based on a condition
Q63
Q63 In a MongoDB query, what does using the $expr operator allow you to do?
Compare fields from the same document
Perform aggregation functions in a query
Use regular expressions in queries
None of the above
Q64
Q64 Which MongoDB operator would you use to find documents where the 'age' field is greater than 30?
$gt
$gte
$lt
$lte
Q65
Q65 How do you write a query to find documents where 'status' is either 'A' or 'D'?
db.collection.find({status: {$in: ["A", "D"]}})
db.collection.find({status: ["A", "D"]})
db.collection.find({status: {$or: ["A", "D"]}})
db.collection.find({$or: [{status: "A"}, {status: "D"}]})
Q66
Q66 How would you find documents where the 'age' field exists and is not equal to 25?
db.collection.find({age: {$ne: 25, $exists: true}})
db.collection.find({age: {$exists: true, $ne: 25}})
db.collection.find({age: {$not: 25}})
db.collection.find({age: {$exists: true}, age: {$ne: 25}})
Q67
Q67 What query would you use to find documents where the 'age' field is less than 30 or the 'status' is 'A'?
db.collection.find({age: {$lt: 30}, status: "A"})
db.collection.find({$or: [{age: {$lt: 30}}, {status: "A"}]})
db.collection.find({age: {$lt: 30} || {status: "A"}})
db.collection.find({age: {$lt: 30}, status: {$eq: "A"}})
Q68
Q68 Identify the issue with this query:
db.collection.find({age: {$gt: "30"}})
Incorrect operator
Incorrect field
Type mismatch in value
Missing field
Q69
Q69 Why might the query db.collection.find({status: {$all: ["A", "D"]}}) return no results?
The $all operator is used incorrectly
The 'status' field doesn't contain arrays
The 'status' field doesn't exist
There are no documents with both 'A' and 'D' in the 'status' field
Q70
Q70 What is the most common cause for a MongoDB $regex query to perform poorly?
Searching in a large collection
Not using an index
Complex regular expression
Case-insensitive search
Q71
Q71 What is the primary role of authentication in MongoDB?
To encrypt data
To create backups
To verify the identity of users
To log transactions
Q72
Q72 Which feature in MongoDB provides the ability to control user access to database resources and operations?
Indexing
Sharding
Role-based access control
Data validation
Q73
Q73 What is the purpose of enabling TLS/SSL in MongoDB?
To speed up data retrieval
To compress data
To secure data communication
To manage data replication
Q74
Q74 In MongoDB, what is "Field Level Redaction"?
Encrypting sensitive fields in a document
Preventing users from deleting fields
Controlling visibility of specific fields based on user privileges
Automatically deleting fields after a certain period
Q75
Q75 How does MongoDB's Auditing feature contribute to database security?
By encrypting audit logs
By tracking database activity and changes
By periodically scanning for vulnerabilities
By updating security patches automatically
Q76
Q76 Which command in MongoDB is used to create a new user?
db.createUser()
db.newUser()
db.addUser()
db.registerUser()
Q77
Q77 How do you enable authorization in MongoDB using a configuration file?
Set authorization: enabled in mongod.conf
Set security.authorization: enabled in mongod.conf
Set userAuthentication: true in mongod.conf
Set dbAccess: granted in mongod.conf
Q78
Q78 How can you restrict a user's read access to only specific fields in a collection in MongoDB?
Use role-based access control with field-level restrictions
Implement a custom authentication mechanism
Modify the collection schema
Encrypt specific fields in the collection
Q79
Q79 Identify the issue if a MongoDB user cannot authenticate with their username and password.
Incorrect username or password
The MongoDB server is down
Lack of network connectivity
Database corruption
Q80
Q80 What could be the reason if a user with read-only access is able to write to the database in MongoDB?
The user has been granted additional privileges inadvertently
The read-only role is misconfigured
The database is in read-write mode
There is a replication lag
Q81
Q81 What is the main purpose of replication in MongoDB?
To distribute data across multiple machines for load balancing
To back up data
To ensure data availability and redundancy
To compress data
Q82
Q82 How does sharding in MongoDB enhance performance?
By reducing the load on a single server
By compressing data
By encrypting data
By automatically deleting old data
Q83
Q83 In MongoDB, what is a shard key and what is its significance?
A key to encrypt shard data
A key used for connecting to a shard
A field used to distribute documents across shards
A key used for backing up shards
Q84
Q84 What is the role of an arbiter in MongoDB replication?
To store a copy of the data for backup purposes
To vote in elections for the primary node
To balance load between replicas
To compress data in transit
Q85
Q85 How do you add a new shard to an existing sharded cluster in MongoDB?
Use the sh.addShard() method
Use the db.addShard() command
Modify the sharding configuration file
Restart the cluster with the new shard configuration
Q86
Q86 How can you change the shard key of a collection in MongoDB?
By using the sh.moveChunk() command
By dropping and recreating the collection
By updating the shard key in the collection settings
Shard keys cannot be changed
Q87
Q87 What is the most likely cause of a delay in replication in a MongoDB replica set?
Network issues
Disk space limitations on the secondary nodes
High read/write operations on the primary
Hardware inefficiencies
Q88
Q88 If a MongoDB shard appears to be unresponsive, what could be a primary reason?
The shard server is down
The configuration server is not accessible
Network partitioning
Overloaded data requests
Q89
Q89 What is the primary function of MongoDB drivers?
To increase database performance
To encrypt data
To facilitate interaction between different programming languages and MongoDB
To manage user authentication
Q90
Q90 In the context of MongoDB, what is connection pooling?
A method of encrypting database connections
A mechanism to maintain and manage a cache of database connections
A tool for monitoring database performance
A strategy for replicating database connections