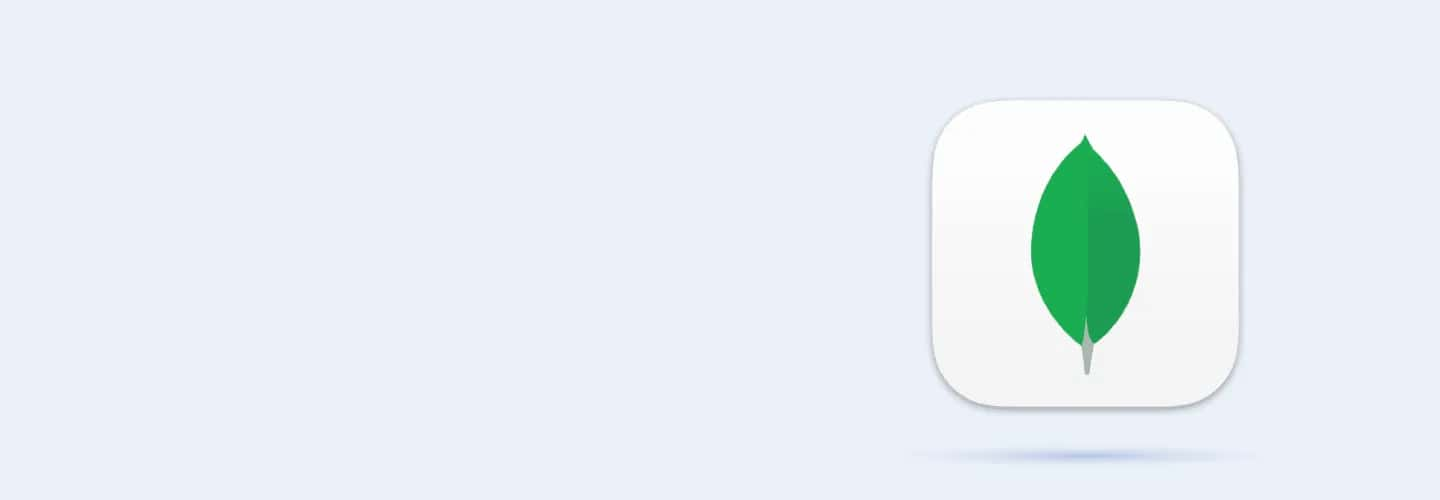
Q31
Q31 What is the primary benefit of embedding documents in MongoDB?
To speed up queries
To enforce data consistency
To reduce data duplication
To simplify query syntax
Q32
Q32 In MongoDB, when should you use references instead of embedding?
When data size is small
When data is frequently updated
When data is rarely accessed
When data duplication is a concern
Q33
Q33 How does MongoDB handle schema validation?
Automatically upon every insert and update
Only during collection creation
Through user-defined validation rules
It does not support schema validation
Q34
Q34 What is the advantage of using a normalized data model in MongoDB?
Improved write performance
Increased data redundancy
Reduced data redundancy
Simplified queries
Q35
Q35 In MongoDB, what is a potential drawback of deeply nested documents?
Increased query performance
Simplified data retrieval
Limitations in depth and size
Improved data consistency
Q36
Q36 Which command can be used to create a schema validation rule in MongoDB?
db.createCollection("users", {validator: {...}})
db.users.setSchema({...})
db.users.validateSchema({...})
db.schema.create({...})
Q37
Q37 How can you enforce that all documents in the 'books' collection have a 'title' field in MongoDB?
db.books.update({}, {$set: {title: {$exists: true}}})
db.books.createIndex({title: 1}, {unique: true})
db.books.setValidator({title: {$exists: true}})
db.books.modifySchema({title: {$exists: true}})
Q38
Q38 What does this MongoDB command do:
db.runCommand({collMod: "mycollection", validator: {...}})?
Modifies an existing collection's schema
Deletes and recreates the collection with a new schema
Creates a new collection
Runs a database maintenance operation
Q39
Q39 How would you add a field with a default value to an existing MongoDB collection?
db.collection.updateMany({}, {$set: {newField: "defaultValue"}})
db.collection.setDefault({newField: "defaultValue"})
db.collection.addField("newField", "defaultValue")
db.collection.modify({}, {$setDefault: {newField: "defaultValue"}})
Q40
Q40 Identify the issue:
db.createCollection("users", {validation: {age: {$type: "int"}}})
Incorrect syntax for schema validation
The 'age' field type should be 'number'
The 'users' collection already exists
No issue
Q41
Q41 What's wrong with this schema validation rule:
{validator: {email: {$regex: /@mongodb.com$/}}}?
The regex is incorrect
The validator should be set on the collection level
The field 'email' doesn't exist
No issue
Q42
Q42 Why might db.users.insert({name: "John", age: "30"}) fail in a collection with schema validation?
The 'age' field is not an integer
The 'name' field is duplicated
There's no 'age' field in the schema
There's no issue
Q43
Q43 Spot the error in nested schema validation:
{validator: {address: {city: {$type: "string"}}}}
'address' must be an embedded document
'city' is not a valid field type
Nested validation is not supported
No error
Q44
Q44 What is the primary purpose of indexing in MongoDB?
To increase data storage efficiency
To enhance security
To speed up query performance
To simplify data aggregation
Q45
Q45 What is a compound index in MongoDB?
An index on a single field
An index combining multiple fields
A unique index
A text index
Q46
Q46 What happens when you create an index on a field that has duplicate values in MongoDB?
The index creation fails
The duplicate values are removed
Only the first occurrence is indexed
The index is created with no issues
Q47
Q47 In MongoDB, what is a covered query?
A query that only needs indexes to return results
A query that retrieves all fields of a document
A query that updates data
A query that requires sorting
Q48
Q48 How does the $group stage in the aggregation pipeline operate?
It sorts the documents
It filters the documents
It groups documents by specified criteria
It merges documents into a single document
Q49
Q49 What is the impact of indexing on write performance in MongoDB?
It improves write performance
It has no impact
It can slow down write performance
It varies depending on the document size
Q50
Q50 Which command creates a simple index on the 'name' field in MongoDB?
db.collection.createIndex({name: 1})
db.collection.index({name: 1})
db.collection.addIndex({name: 1})
db.collection.setIndex({name: 1})
Q51
Q51 How do you create a descending index on the 'age' field in MongoDB?
db.collection.createIndex({age: -1})
db.collection.createIndex({age: "desc"})
db.collection.createIndex({age: 0})
db.collection.createIndex({age: "descending"})
Q52
Q52 What is the purpose of the $match stage in MongoDB's aggregation pipeline?
To sort documents
To group documents
To filter documents based on criteria
To limit the number of documents
Q53
Q53 How can you create a text index on two fields, 'title' and 'description', in MongoDB?
db.collection.createIndex({title: "text", description: "text"})
db.collection.createTextIndex({title, description})
db.collection.addTextIndex({title, description})
db.collection.createIndex({title: 1, description: 1}, {type: "text"})
Q54
Q54 Identify the error in this command:
db.collection.createIndex({name: "1"})
Incorrect index type
Incorrect field name
Syntax error in the index specification
No error
Q55
Q55 Why might an aggregation pipeline return incorrect results after adding a new index?
The index is not being used
The index is corrupt
The index has caused a change in the data order
The pipeline does not support indexing
Q56
Q56 What could be wrong if db.collection.createIndex({email: 1}, {unique: true}) fails to enforce uniqueness?
The collection already has duplicate emails
The index is not properly configured
The 'email' field is not indexed
MongoDB does not support unique indexes
Q57
Q57 Spot the error:
An aggregation pipeline with $sort, $match, and $group stages returns no results.
The $sort stage is misplaced
The $match stage is using incorrect criteria
The $group stage has an error in the grouping key
No error
Q58
Q58 Which operator is used in MongoDB to select documents where a field equals a specified value?
$eq
$set
$match
$get
Q59
Q59 What does the $in operator do in a MongoDB query?
Selects documents where a field's value is any of the specified array values
Changes the value of a field to one within a specified array
Counts how many times a field's value appears in a specified array
None of the above
Q60
Q60 What is the purpose of the $and operator in MongoDB queries?
To perform a logical AND operation on an array of two or more expressions
To compare two fields in the same document
To add new fields to documents
To update data in a document conditionally