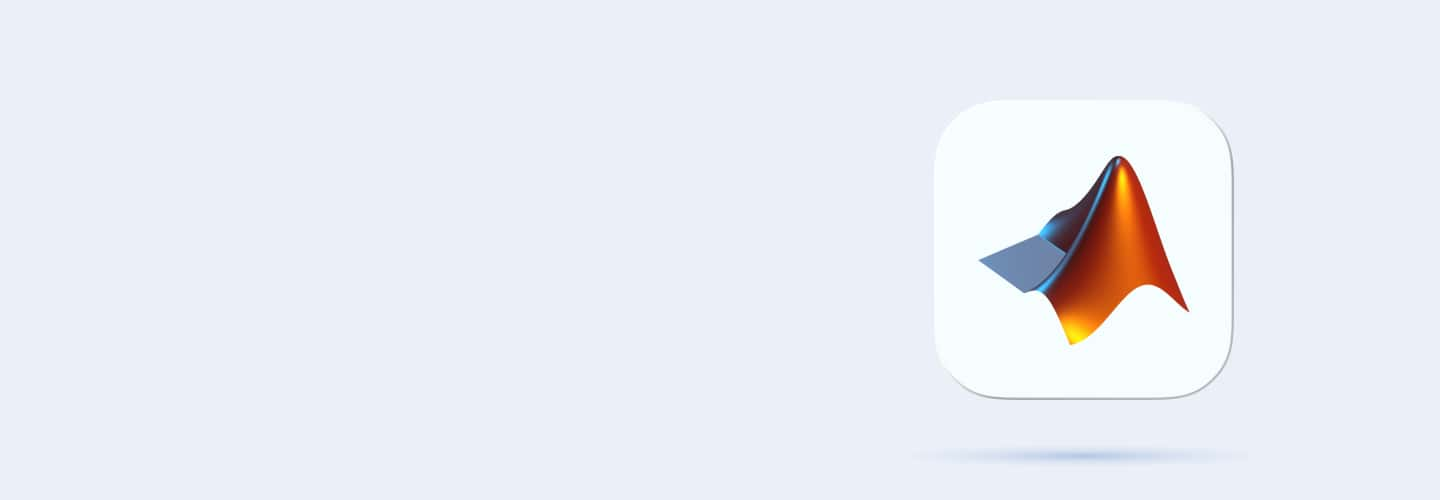
Q91
Q91 How can you load variables from a .mat file?
read()
import()
load()
retrieve()
Q92
Q92 What is the purpose of the "fopen" function?
Opens a file for reading or writing
Saves a file
Creates a directory
Reads data into a matrix
Q93
Q93 Which MATLAB function reads data from a text file?
fscanf()
textread()
fread()
csvread()
Q94
Q94 What is the difference between "fprintf" and "fwrite"?
"fprintf" writes formatted text, "fwrite" writes binary data
Both write binary data
Both write formatted text
"fprintf" writes binary data, "fwrite" writes formatted text
Q95
Q95 Write a MATLAB command to save the variables x and y to a file "data.mat".
save('data.mat', 'x', 'y')
store('data.mat', x, y)
export('data.mat', x, y)
savefile('data.mat', x, y)
Q96
Q96 How can you write data to a CSV file in MATLAB?
csvwrite('file.csv', data)
writecsv('file.csv', data)
exportcsv('file.csv', data)
savecsv('file.csv', data)
Q97
Q97 Write a MATLAB code snippet to read a matrix from a text file.
fileID = fopen('file.txt', 'r'); data = fscanf(fileID, '%f'); fclose(fileID);
data = load('file.txt');
fileID = open('file.txt', 'r'); data = read(fileID);
fileID = fopen('file.txt', 'r'); data = fscanf(fileID, '%d'); fclose(fileID);
Q98
Q98 Why does "Permission denied" occur when accessing a file?
File is open in another program
File does not exist
Incorrect file permissions
All of the above
Q99
Q99 Why does "Invalid file identifier" occur in MATLAB?
File is already closed
File was not successfully opened
Incorrect file name
All of the above
Q100
Q100 Why does "End of file reached" occur unexpectedly in MATLAB?
File reading completed
File contains empty lines
Incorrect read format
File is not opened
Q101
Q101 What is the purpose of the Signal Processing Toolbox?
Analyzing and visualizing signals
Performing data visualization
Creating neural networks
Solving linear equations
Q102
Q102 Which toolbox is used for processing images?
Optimization Toolbox
Image Processing Toolbox
Signal Processing Toolbox
Statistics Toolbox
Q103
Q103 What is the role of the Optimization Toolbox?
Solving optimization problems
Creating graphs
Analyzing matrices
Generating random data
Q104
Q104 Which toolbox is useful for deep learning tasks?
Neural Network Toolbox
Optimization Toolbox
Signal Processing Toolbox
Control System Toolbox
Q105
Q105 What is the purpose of the Statistics Toolbox?
Performing statistical analysis and modeling
Creating 3D plots
Solving differential equations
Processing audio signals
Q106
Q106 Write a MATLAB command to perform edge detection in an image.
edge(img, 'Canny')
detectedge(img)
filteredge(img, 'Canny')
edge(img)
Q107
Q107 Write a MATLAB code snippet to calculate the Fast Fourier Transform (FFT) of a signal.
fft(signal)
fft2(signal)
transform(signal)
fourier(signal)
Q108
Q108 Write a MATLAB command to perform a 2D convolution of two matrices A and B.
conv2(A, B)
conv(A, B)
convolve(A, B)
filter2(A, B)
Q109
Q109 Why does "Undefined function or variable" occur when using a toolbox function?
Toolbox is not installed
Incorrect syntax
File is missing
All of the above
Q110
Q110 Why does "License Manager Error -5" occur in MATLAB?
Toolbox license is unavailable
Function is undefined
File is missing
Incorrect MATLAB version
Q111
Q111 What is the primary purpose of debugging in MATLAB?
To optimize code
To fix errors and bugs
To run scripts
To visualize data
Q112
Q112 Which MATLAB command is used to set breakpoints in code?
stop
pause
break
dbstop
Q113
Q113 What does the "try-catch" block do in MATLAB?
Handles errors during runtime
Runs code in parallel
Loops through errors
Suppresses warnings
Q114
Q114 What is the difference between "error" and "warning" functions in MATLAB?
"error" halts execution; "warning" does not
"warning" halts execution; "error" does not
Both halt execution
Both suppress warnings
Q115
Q115 Write a MATLAB command to display an error message "Invalid input".
error('Invalid input')
msg('Invalid input')
throw('Invalid input')
disp('Invalid input')
Q116
Q116 Write a MATLAB code snippet to handle an error using "try-catch".
try x = 1/0; catch disp('Error occurred'); end
try x = 1; catch disp('Error occurred'); end
try x = 1/0; catch disp('No errors'); end
try x = 1; catch disp('No errors'); end
Q117
Q117 Write a MATLAB function that throws an error if input is not positive.
function checkInput(x), if x <= 0, error('Input must be positive'); end; end
function checkInput(x), if x >= 0, error('Input must be positive'); end; end
function checkInput(x), if x < 0, error('Input must be positive'); end; end
function checkInput(x), if x > 0, error('Input must be positive'); end; end
Q118
Q118 What does the "dbclear" command do?
Clears breakpoints
Stops execution
Pauses debugging
Clears variables
Q119
Q119 Why does MATLAB throw "Out of memory" errors?
Large data size
Incorrect syntax
Undefined variable
Missing semicolon
Q120
Q120 What is Simulink used for in MATLAB?
System simulation and modeling
Creating plots
Statistical analysis
Data visualization