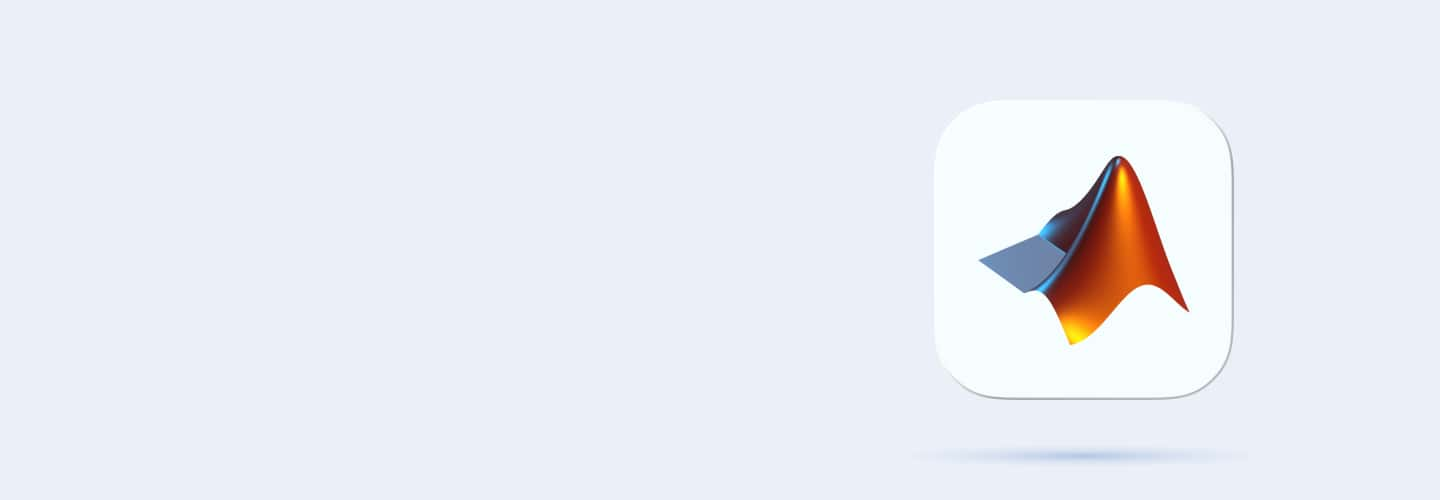
Q61
Q61 How can you pass multiple arguments to a function?
function result = myFunc(arg1, arg2)
function myFunc(arg1, arg2)
function result = myFunc[arg1, arg2]
function myFunc(arg1 arg2)
Q62
Q62 Create a function to calculate the factorial of a number.
function f = fact(n), if n == 0, f = 1; else, f = n * fact(n-1); end; end
function f = fact(n), f = n * fact(n+1); end
function f = fact(n), f = n^2; end
function fact(n), disp(factorial(n)); end
Q63
Q63 What happens if a function name and a variable name conflict?
Error
Variable is ignored
Function is ignored
Unexpected behavior
Q64
Q64 Why does "Too many input arguments" occur?
Passing fewer arguments than required
Passing more arguments than defined
Using undefined variables
Function name conflict
Q65
Q65 Why does "Not enough input arguments" occur?
Missing input values in the function call
Incorrect function definition
Conflicting variable names
Undefined function
Q66
Q66 Which function is used to create a basic 2D plot?
graph()
plot()
plot2D()
chart()
Q67
Q67 What does the "title()" function do in MATLAB?
Adds a title to a plot
Adds a legend to a plot
Adds a label to the axes
Creates a subplot
Q68
Q68 How do you label the x-axis of a plot?
xlabel("label")
ylabel("label")
axislabel("label")
xaxis("label")
Q69
Q69 What is the purpose of the "legend()" function?
Adds a legend to a plot
Adds a title to a plot
Creates a new figure
Sets the axis limits
Q70
Q70 Which function is used for creating a 3D surface plot?
meshgrid()
surf()
surface3D()
plot3D()
Q71
Q71 Write a code snippet to plot a sine wave.
x = 0:0.1:10; y = sin(x); plot(x, y);
x = linspace(0, 10, 100); y = cos(x); plot(y, x);
x = 0:0.1:10; y = cos(x); plot(x, y);
x = 1:0.1:10; y = sin(x); plot(y, x);
Q72
Q72 How do you plot multiple lines on the same graph?
plot(x1, y1, x2, y2)
multiplot(x1, y1, x2, y2)
lines(x1, y1, x2, y2)
plotlines(x1, y1, x2, y2)
Q73
Q73 Write a MATLAB code to create a bar chart.
bar(x, y)
barchart(x, y)
plotbar(x, y)
chartbar(x, y)
Q74
Q74 Write a MATLAB code snippet to create a 3D plot of z = x^2 + y^2.
[x, y] = meshgrid(-10:1:10); z = x.^2 + y.^2; surf(x, y, z);
[x, y] = meshgrid(-10:1:10); z = x^2 + y^2; plot(x, y, z);
[x, y] = meshgrid(-10:1:10); z = x + y; surf(x, y, z);
[x, y] = meshgrid(-10:1:10); z = x.^2 + y.^2; plot(x, y, z);
Q75
Q75 Why does "Index exceeds matrix dimensions" occur when plotting?
Incorrect axis limits
Matrix size mismatch
Undefined variable
Incorrect syntax
Q76
Q76 What causes "Invalid color specification" in MATLAB?
Undefined variable
Unsupported color format
Missing semicolon
Incorrect axis label
Q77
Q77 Why does "Array dimensions do not match" occur in a plot?
Missing function argument
Array sizes are incompatible
Undefined function
Matrix is empty
Q78
Q78 What does the "zeros" function do in MATLAB?
Creates an array of zeros
Initializes variables to zero
Generates random zeros
None of the above
Q79
Q79 How do you create a 3x3 identity matrix in MATLAB?
identity(3)
eye(3)
ones(3)
diag(3)
Q80
Q80 Which function returns the size of a matrix?
length()
size()
dim()
rows()
Q81
Q81 What does "diag" function do in MATLAB?
Creates a diagonal matrix
Finds the determinant
Returns the trace
Transposes a matrix
Q82
Q82 How do you access the last element of a matrix "A"?
A(end)
A(last)
A(size(A))
A(-1)
Q83
Q83 Write a MATLAB command to create a row vector from 1 to 10.
1:10
[1,10]
linspace(1,10,10)
ones(1,10)
Q84
Q84 How do you transpose a matrix "A" in MATLAB?
transpose(A)
A'
flip(A)
inv(A)
Q85
Q85 Write a MATLAB command to create a 2x2 matrix with random values.
rand(2)
random(2)
randmatrix(2)
rand2(2)
Q86
Q86 Write a MATLAB code snippet to calculate the inverse of a matrix "A".
inv(A)
inverse(A)
A^-1
1/A
Q87
Q87 Why does "Index exceeds matrix dimensions" occur in MATLAB?
Accessing an element outside the matrix size
Matrix is not initialized
Matrix contains invalid data
Incorrect syntax
Q88
Q88 Why does "Singular matrix" error occur when inverting a matrix?
Matrix is not square
Matrix determinant is zero
Matrix dimensions do not match
Matrix contains NaN
Q89
Q89 What causes "Matrix dimensions must agree" in element-wise operations?
Mismatched dimensions
Unsupported operator
Empty matrix
NaN values
Q90
Q90 Which function is used to save variables to a file in MATLAB?
save()
write()
store()
export()