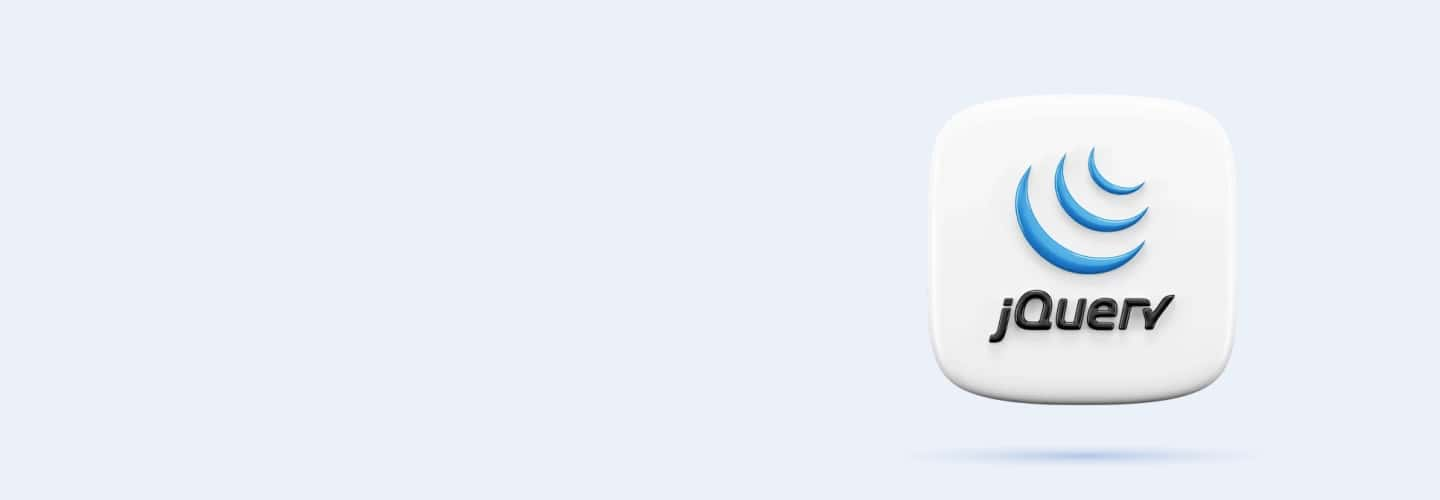
Q121
Q121 How can you safely use jQuery in noConflict mode while using another library that also uses $?
var jq = jQuery.noConflict();
jQuery.noConflict(true);
var $j = $.noConflict();
var $j = jQuery.noConflict();
Q122
Q122 How do you extend jQuery's capabilities in a way that minimizes conflict with other loaded libraries?
By modifying jQuery's prototype directly
By adding new methods using jQuery.fn.extend()
By rewriting existing jQuery methods
By isolating jQuery in an IIFE
Q123
Q123 What diagnostic approach should be used if jQuery scripts conflict with another library after an update?
Review the jQuery migration guide
Check for deprecated methods
Examine the console for errors
All of the above
Q124
Q124 How can you identify and resolve script execution order issues when using jQuery with other libraries?
Use script loaders
Adjust the order of script tags manually
Both
Implement callbacks for dependent scripts
Q125
Q125 What is the first step in debugging jQuery code?
Checking the jQuery version compatibility
Reviewing the console for errors
Ensuring jQuery is properly loaded
Verifying HTML structure
Q126
Q126 How can "linting" tools be beneficial for jQuery code debugging?
They automatically correct syntax errors
They optimize code performance
They help identify syntax and other errors
They encrypt the code
Q127
Q127 What is an effective strategy for debugging complex jQuery selectors?
Break down selectors into simpler parts
Use only ID selectors
Avoid using CSS classes
Use inline styles instead of selectors
Q128
Q128 Which jQuery function is essential for checking whether the DOM is fully loaded before running script?
$(document).ready()
$(window).load()
$(document).start()
$(document).init()
Q129
Q129 How can you use the .error() method in jQuery for debugging?
Attach it to load events to handle errors
Apply it to any jQuery object
Use it to log errors in AJAX requests
It's deprecated
Q130
Q130 What is a simple check to ensure that a jQuery selector is working as expected?
Verify the selector in the console
Change the selector until it works
Use a default selector
Ask a colleague for help
Q131
Q131 What is a significant benefit of using .on() for event delegation in jQuery for dynamic content?
It reduces memory usage by managing fewer event handlers
It allows easier management of events
It isolates event handlers
It simplifies event handler updates
Q132
Q132 What debugging technique can provide insights into the sequence of jQuery event firing?
Adding console.log() statements within event handlers
Using performance profiling tools
Monitoring network requests
Setting conditional breakpoints
Q133
Q133 How can you identify memory leaks in jQuery applications?
Monitoring memory usage over time in browser tools
Checking for detached DOM elements
Both
None of the above
Q134
Q134 What is the primary purpose of unit testing in jQuery code?
To check for syntax errors
To ensure that each function works as intended
To enhance performance
To simplify code
Q135
Q135 How does integration testing differ from unit testing for jQuery code?
Integration testing combines all modules and tests them as a group
Integration testing tests a single function at a time
Integration testing is only for client-side scripts
Integration testing is quicker
Q136
Q136 What role does automated testing play in maintaining jQuery code?
It helps prevent regressions by automatically testing code changes
It replaces manual testing completely
It only tests new code
It simplifies the debugging process
Q137
Q137 Which tool is commonly used for writing automated tests for jQuery functions?
Selenium
Jasmine
QUnit
Mocha
Q138
Q138 How can you simulate user interactions in jQuery code testing?
Using $.trigger() to mimic events
Using .click() to force actions
Using setTimeout()
Using manual testing
Q139
Q139 What is a quick method to verify that jQuery is triggering the correct events during testing?
Checking the event log in the browser's developer console
Using alert boxes
Using console.log in event handlers
Rewriting the event handlers
Q140
Q140 How can breakpoints be useful in testing jQuery code?
They can pause execution at critical points
They can modify code on the fly
They can bypass errors
They can simulate server responses
Q141
Q141 What should you do when a jQuery test unexpectedly fails after recent changes to the code?
Revert all changes
Run the test in a different environment
Check the change log for clues
All of the above
Q142
Q142 How do you address intermittent test failures in jQuery, especially those caused by asynchronous operations?
Increasing timeout settings
Refactoring the tests to handle asynchronous results
Running the tests multiple times
Ignoring the failures
Q143
Q143 Why is it recommended to load jQuery from a CDN?
Faster load times due to cached versions
It is more secure
It provides automatic updates
It requires less configuration
Q144
Q144 What is the advantage of using method chaining in jQuery?
It reduces the readability of code
It increases execution speed by minimizing DOM access
It simplifies the syntax
It increases memory usage
Q145
Q145 How can minimizing the use of global selectors improve jQuery performance?
It reduces the chance of selecting unintended elements
It limits the scope of each search, reducing processing time
It enhances security
It simplifies debugging
Q146
Q146 What is the best practice for attaching event handlers to elements that are dynamically added to the DOM?
Using .click() directly on the elements
Using .on() delegated from a static parent
Using .bind() on each element individually
Using .live() on document
Q147
Q147 How should you handle jQuery AJAX requests to ensure they are maintainable and scalable?
By using the global .ajaxSetup() for all configurations
By customizing each request with specific parameters
By always using synchronous requests
By limiting AJAX calls to client-side operations only
Q148
Q148 What strategy is effective for debugging jQuery code that interacts with a lot of dynamic content?
Setting breakpoints in all scripts
Logging all actions in the console
Using the MutationObserver API
Inspecting elements in real time
Q149
Q149 What should be verified to ensure a jQuery script that works in development also functions correctly in production?
Checking for any CORS issues
Ensuring all external resources are correctly loaded
Verifying that the jQuery version matches
Confirming script load order
Q150
Q150 How do you ensure that jQuery code is future-proof, especially concerning deprecations and changes in the library?
Regularly updating the code according to the jQuery migration guide
Ignoring deprecation warnings
Using older versions of jQuery indefinitely
Using only core jQuery features