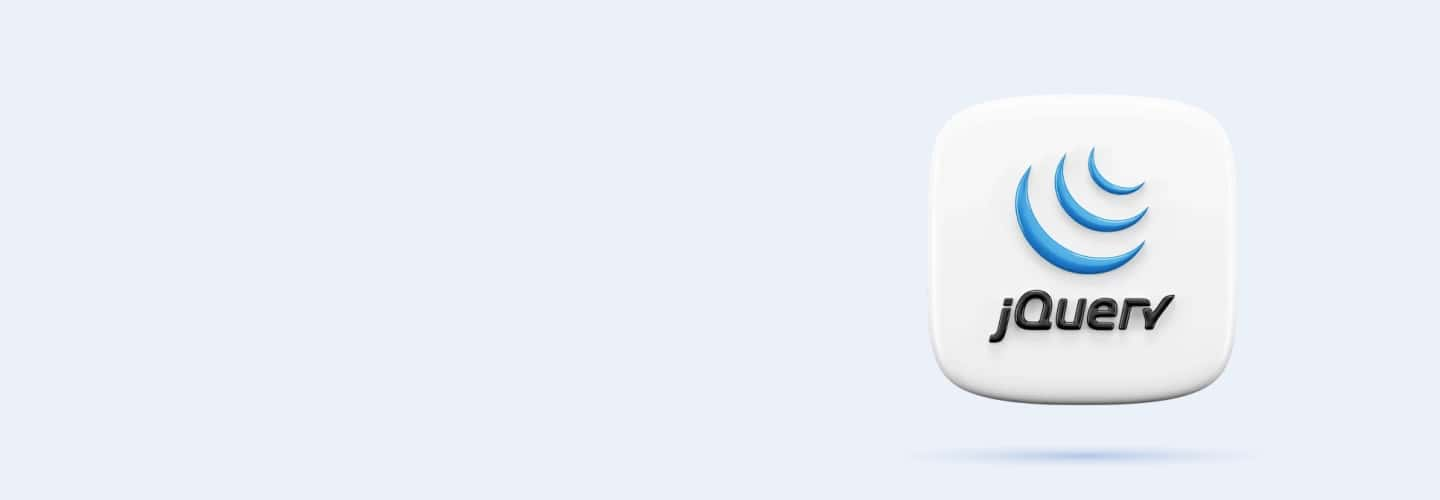
Q91
Q91 What is the purpose of the .dialog() widget in jQuery UI?
To display messages
To create a popup window
To confirm user actions
To display forms
Q92
Q92 Which method is used to disable a jQuery UI button?
.disableButton()
.button("disable")
.disable()
.buttonDisable()
Q93
Q93 How can you prevent a dialog from closing on jQuery UI?
Using the .preventClose() method
Modifying the closeText option
Setting the modal option to true
Overriding the close event
Q94
Q94 How do you initialize a datepicker on a text input field in jQuery UI?
$("#date").datepicker()
$("#date").initDatepicker()
$("#date").setDatepicker()
$("#date").createDatepicker()
Q95
Q95 What jQuery UI method is used to make an element resizable?
.resizable()
.makeResizable()
.enableResize()
.setResizable()
Q96
Q96 How do you create a custom animation effect on a dialog box using jQuery UI?
.dialog({effect: "bounce"})
.dialog({show: {effect: "bounce", duration: 1000}})
.animateDialog("bounce")
.dialogAnimate("bounce")
Q97
Q97 What should you check if a jQuery UI widget is not responding to user interactions?
jQuery UI is not loaded
The widget's script is corrupted
The browser is incompatible
JavaScript is disabled in the browser
Q98
Q98 How can you solve an issue where a jQuery UI dialog does not open?
Check if the dialog div is hidden
Ensure the .dialog() is correctly called
Verify there are no JavaScript errors in the console
All of the above
Q99
Q99 How does the .delegate() method function in jQuery?
It triggers a delegated event
It attaches a handler to the parent for any event that occurs on matching children
It directly binds events to elements
It replaces all previous events
Q100
Q100 What is "chaining" in jQuery?
Linking several methods on different jQuery selectors
Connecting similar events into a single handler
Applying multiple methods in a single, continuous line of code on the same element set
Combining multiple CSS files into one request
Q101
Q101 How can you use jQuery to dynamically load a script?
Using the .loadScript() method
Using the .getScript() method
Using the .ajax() method with a script parameter
Using the .script() method
Q102
Q102 What is the purpose of the jQuery.noConflict() method?
To avoid conflicts with other JavaScript libraries that use the $ symbol
To reset jQuery variables
To clear all jQuery data from a page
To reload jQuery settings
Q103
Q103 How does jQuery handle custom events and what are they used for?
Custom events are used to handle native browser operations
They're used to manage AJAX requests exclusively
They allow developers to define and trigger their own events
They are a method to speed up event handling
Q104
Q104 How do you attach a global AJAX event handler that fires whenever an AJAX request completes?
$(document).ajaxComplete(function() {})
$.on('ajaxComplete', function() {})
$.ajaxSetup({complete: function() {}})
$(document).on('ajaxComplete', function() {})
Q105
Q105 What method can be used to extend jQuery's default settings for all AJAX requests?
.extendAjax()
.ajaxExtend()
.ajaxSetup()
.setupAjax()
Q106
Q106 How can you implement a jQuery method that supports promise-like behavior for asynchronous operations?
Using .defer()
Using .promise()
Using .async()
Using .wait()
Q107
Q107 What should you investigate if a custom jQuery plugin does not trigger events as expected?
Plugin's event binding
Conflict with jQuery versions
Syntax errors in event names
Incorrect method calls
Q108
Q108 What is an effective approach to troubleshoot performance issues with jQuery animations on a complex page?
Checking for excessive DOM manipulations
Optimizing event handlers
Utilizing jQuery's Performance tool
Implementing throttling or debouncing techniques
Q109
Q109 What jQuery function is most effective for minimizing DOM access?
.closest()
.find()
.children()
.end()
Q110
Q110 Why is it advisable to use event delegation in jQuery?
Reduces memory usage by handling events at a parent level
Increases the number of event handlers
Simplifies code complexity
Enhances page loading speed
Q111
Q111 What is the impact of chaining multiple methods in jQuery?
Reduces readability
Increases execution time
Optimizes performance by reducing the scope of DOM traversal
Can lead to errors
Q112
Q112 How does minimizing the number of global selectors improve jQuery performance?
Reduces the scope of each search
Lowers memory footprint
Decreases the chance of selecting unintended elements
All of the above
Q113
Q113 What technique should be used to append multiple elements efficiently in jQuery?
Appending each element individually
Creating a string of HTML and appending once
Using a document fragment
Looping through an array of elements
Q114
Q114 How can jQuery's .detach() method be utilized to enhance performance when modifying DOM elements?
Detaching elements, making modifications, and reattaching
Simply removing elements temporarily
Clearing content before reinsertion
Using it instead of .remove()
Q115
Q115 What should be checked first if jQuery animations are slow and jittery?
Check if CSS transitions could be used instead
Ensure no heavy scripts are running concurrently
Verify jQuery version compatibility
Optimize image sizes
Q116
Q116 How do you identify and resolve performance bottlenecks caused by jQuery selectors?
Use browser performance tools to analyze selector efficiency
Simplify selectors
Increase specificity of selectors
All of the above
Q117
Q117 What is the primary function of the jQuery.noConflict() method when using jQuery with other libraries?
To import other libraries into jQuery
To enhance jQuery performance
To relinquish control of the $ symbol
To merge two libraries
Q118
Q118 How does jQuery ensure compatibility when used alongside other JavaScript libraries?
By automatically adjusting its methods
By using its own version of the JavaScript engine
By using a compatibility mode
By minimizing its footprint
Q119
Q119 What strategy should be adopted to handle potential conflicts when integrating jQuery with another library?
Loading jQuery first
Defining all libraries in separate JavaScript files
Using aliasing for jQuery
Loading all libraries asynchronously
Q120
Q120 What is a recommended practice when loading multiple JavaScript libraries including jQuery?
Load libraries synchronously
Load libraries in the footer
Use a single minified file for all libraries
Use asynchronous loading methods