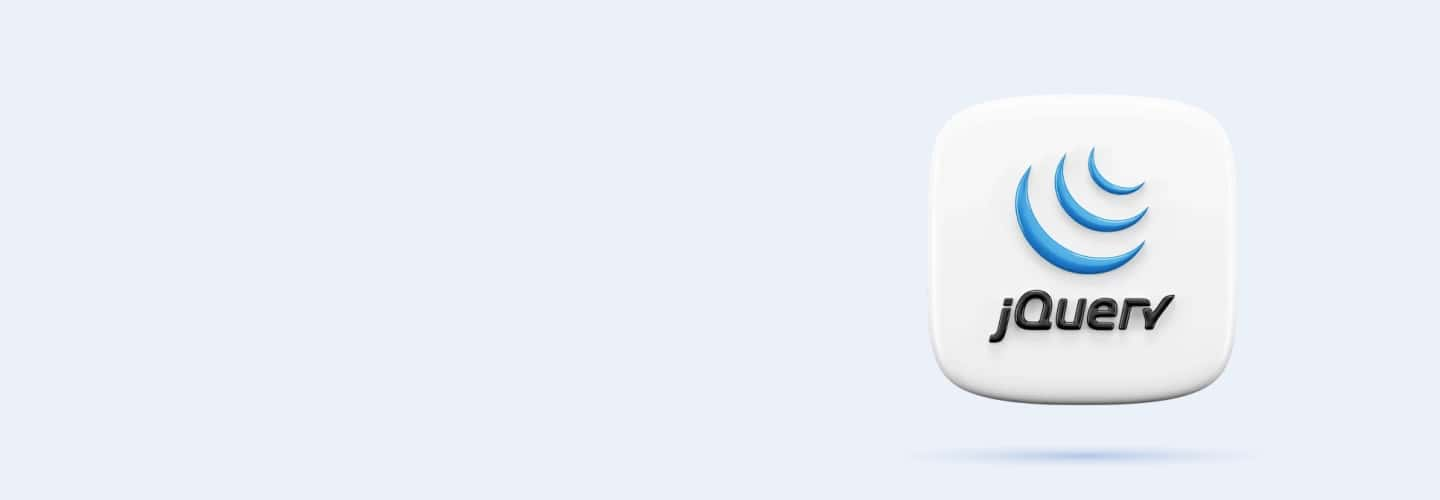
Q61
Q61 How do you dynamically change the style of an element based on certain conditions in jQuery?
.css('style', 'value')
.style('style', 'value')
.changeStyle('style', 'value')
.alter('style', 'value')
Q62
Q62 What could be the reason if .remove() is not removing an element as expected?
Element is not selected correctly
Syntax error in jQuery
Element is hidden
Element is locked
Q63
Q63 Why might changes to the DOM via jQuery not appear immediately in the browser?
Browser caching
DOM is not updated
JavaScript is paused
Synchronous processing issues
Q64
Q64 What is the purpose of the $.ajax() method in jQuery?
To load images asynchronously
To send synchronous JavaScript calls
To perform asynchronous HTTP (Ajax) requests
To reload the page
Q65
Q65 Which method in jQuery is used specifically to load data from the server using a HTTP GET request?
$.get()
$.post()
$.load()
$.fetch()
Q66
Q66 How do you specify the type of data expected back from the server in $.ajax()?
Using the dataType option
Using the type option
Using the method option
Using the data option
Q67
Q67 What does the $.post() method do?
Sends a POST request to the server
Sends a GET request to the server
Deletes data from the server
Updates data on the server
Q68
Q68 Which jQuery method is best for retrieving JSON data from a server?
$.getJSON()
$.getScript()
$.ajax()
$.load()
Q69
Q69 How can you handle multiple AJAX requests simultaneously in jQuery?
Using the $.when() method
Using the $.then() method
Using the $.multiAjax() method
Using the $.all() method
Q70
Q70 What is the use of the timeout option in an AJAX request?
To specify the time before a page reloads
To set the delay before an AJAX call is made
To determine how long an AJAX request should wait for a response
To limit the execution time of callbacks
Q71
Q71 How do you use jQuery to send a form data using AJAX by a POST method?
$.ajax({url: 'submit.php', type: 'POST', data: $('#form').serialize()})
$.postForm('submit.php', '#form')
$.sendData('#form', 'submit.php')
$.submit('#form', 'POST')
Q72
Q72 Which option in jQuery AJAX is used to handle errors?
fail()
error()
reject()
catch()
Q73
Q73 How can you attach a success callback that executes after AJAX content is successfully loaded?
.done()
.success()
.complete()
.after()
Q74
Q74 What method allows you to modify a part of the webpage asynchronously without reloading the whole page?
$.ajax({url: 'update.php', success: function(result) {$('#div1').html(result);}})
$.updatePart('update.php', '#div1')
$.partialLoad('update.php', '#div1')
$.loadFragment('update.php', '#div1')
Q75
Q75 What common issue should be checked if an AJAX call is not initiating?
Incorrect URL format
Broken server link
Misconfigured AJAX settings
All of the above
Q76
Q76 Why might an AJAX request return a 404 error?
The requested resource is not found on the server
The server is down
The URL is incorrect
The data type is mismatched
Q77
Q77 What could cause an AJAX request to a secure server to fail in terms of security?
Cross-origin resource sharing (CORS) restrictions
SSL certificate errors
Both
None of these
Q78
Q78 What is the primary purpose of jQuery plugins?
To add new methods to the jQuery library
To fix bugs in jQuery
To replace jQuery with newer versions
To reduce the size of jQuery scripts
Q79
Q79 How do you initialize a plugin in jQuery?
By calling the plugin's method on a jQuery object
By importing the plugin script
By adding a plugin file to the HTML
By linking the plugin in the HTML head
Q80
Q80 What should you consider about jQuery plugins to prevent conflicts with existing functions?
Their namespacing
Their compatibility with other plugins
Their method of invocation
Their event handling
Q81
Q81 What should you verify about a jQuery plugin before integrating it into your project?
Its compatibility with your jQuery version
Its performance impact on your project
Its license and usage terms
Its update frequency
Q82
Q82 How can you minimize conflicts between multiple jQuery plugins?
By using distinct namespaces for plugin functions
By loading plugins in a specific order
By isolating plugin code in separate files
By ensuring plugins do not share global variables
Q83
Q83 How do you activate a lightbox plugin on image elements in jQuery?
By calling .lightbox() on image selectors
By setting a data-lightbox attribute
By adding a 'lightbox' class to images
By including a lightbox attribute in image tags
Q84
Q84 What is a method to enhance or modify a jQuery plugin?
By extending the plugin's functionality through additional scripts
By rewriting core functions of the plugin
By configuring options provided by the plugin
By linking to updated versions of the plugin
Q85
Q85 What technique ensures efficient loading of a heavy jQuery plugin on needed pages only?
Conditionally loading the plugin based on page content
Using asynchronous script loading techniques
Applying selective script loading based on user actions
Deferring plugin loading until after page load
Q86
Q86 What should you check first if a jQuery plugin is not working as expected?
The jQuery version compatibility
Correct inclusion of the plugin script
No conflicts with other scripts
Correct plugin initialization method
Q87
Q87 How can you troubleshoot a jQuery plugin that behaves differently across browsers?
Checking for console errors related to the plugin
Testing the plugin across different browser versions
Using browser-specific debugging tools
Adjusting plugin settings for compatibility
Q88
Q88 What is jQuery UI primarily used for?
Adding HTML elements to the page
Styling web pages
Enhancing user interface interactions
Optimizing backend processes
Q89
Q89 Which jQuery UI widget is used to make a list of items sortable by dragging and dropping?
.draggable()
.sortable()
.selectable()
.resizable()
Q90
Q90 How do you apply a theme to widgets in jQuery UI?
Using CSS files
Using the ThemeRoller application
Using JavaScript functions
Using .theme() method