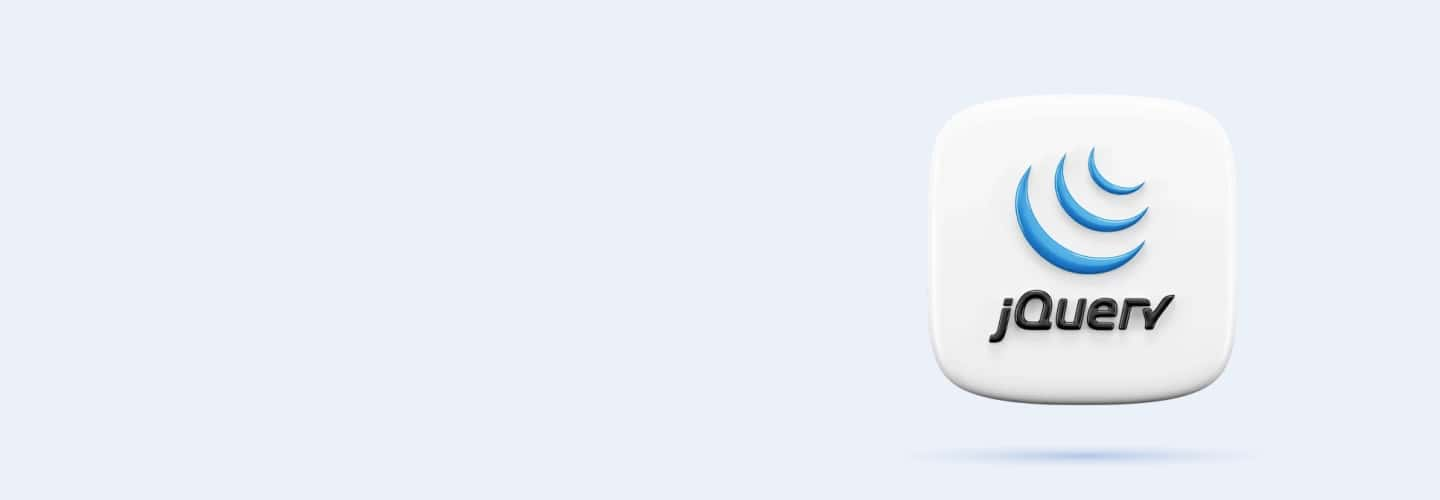
Q31
Q31 Which jQuery function is used to check whether an event is prevented from its default action?
.isDefaultPrevented()
.isPrevented()
.defaultPrevented()
.checkDefault()
Q32
Q32 How do you bind a 'mouseover' event to all paragraphs?
$('p').on('mouseover', function())
$('p').mouseover()
$('p').bind('mouseover')
$('p').trigger('mouseover')
Q33
Q33 What jQuery method would you use to simulate a 'click' event on a button?
$('#button').simulateClick()
$('#button').trigger('click')
$('#button').click()
$('#button').invokeClick()
Q34
Q34 Which method is used to attach a single event handler to multiple events in jQuery?
.one()
.single()
.first()
.on()
Q35
Q35 How do you add a 'dblclick' event to an element with the ID 'dblClickMe'?
$('#dblClickMe').dblclick(function())
$('#dblClickMe').on('dblclick')
$('#dblClickMe').trigger('dblclick')
$('#dblClickMe').bind('dblclick', function())
Q36
Q36 What might cause a 'click' event to trigger multiple times unintentionally?
Multiple event bindings
Obsolete jQuery version
Syntax errors
Incorrect selector
Q37
Q37 If a 'hover' event does not fire as expected, what could be a possible cause?
Element is hidden
Event is not properly bound
CSS issue
All of the above
Q38
Q38 A developer notices that their 'keydown' event isn't working on a dynamically added input field.
What is the likely issue?
Incorrect event binding
Event delegation not used
Outdated jQuery library
None of the above
Q39
Q39 Which method is used to gradually change the opacity of an element?
.fade()
.opacityChange()
.fadeTo()
.transparent()
Q40
Q40 What jQuery function is used to hide elements with a sliding motion?
.slideUp()
.slideHide()
.hideSlide()
.toggleSlide()
Q41
Q41 Which method stops all currently running animations on the selected elements?
.stopAnimations()
.end()
.stop()
.halt()
Q42
Q42 What does the .animate() method in jQuery do?
Creates CSS animations
Manipulates element sizes
Performs custom animations
None of the above
Q43
Q43 How do you make an element visible with a fading effect?
.fadeIn()
.visible()
.showFade()
.appear()
Q44
Q44 How can you animate an element to move to a new position using jQuery?
.moveTo()
.animate({top: '50px', left: '100px'})
.shift()
.relocate()
Q45
Q45 What method is used to execute a series of animations one after another on an element?
.queue()
.series()
.sequence()
.chain()
Q46
Q46 Which jQuery method is used to toggle between hiding and showing elements with a sliding effect?
.slideToggle()
.toggleSlide()
.slide()
.hideShowSlide()
Q47
Q47 How do you apply a bouncing effect to an animation in jQuery?
.bounce()
.animate({bounce: '20px'})
.effect('bounce')
.jump()
Q48
Q48 What is the correct way to slow down an animation effect in jQuery?
.slow()
.delay()
.animate({speed: 'slow'})
.setSpeed('slow')
Q49
Q49 How can you link multiple animations to create a continuous effect without gaps?
.link()
.chain()
.concat()
.queue()
Q50
Q50 Why might an animation not start immediately as expected?
Animation queue is full
CSS conflicts
JavaScript errors
All of the above
Q51
Q51 What could cause an element to fail to animate as expected in jQuery?
Incorrect CSS properties
Animation method not supported
Syntax errors
All of the above
Q52
Q52 How do you add a new element to the end of a specific div using jQuery?
.appendTo('#divId')
.append('#divId')
.insertTo('#divId')
.attachTo('#divId')
Q53
Q53 Which method is used to replace all matched elements with new content?
.replace()
.replaceWith()
.replaceAll()
.exchange()
Q54
Q54 How do you remove all child nodes from a specific element using jQuery?
.empty()
.clear()
.removeChildren()
.deleteAll()
Q55
Q55 What method is used to get the direct parent of an element in jQuery?
.parent()
.getParent()
.up()
.ancestor()
Q56
Q56 How can you clone an element including all data and events associated with it in jQuery?
.clone(true)
.copy()
.duplicate()
.replicate()
Q57
Q57 How do you perform a deep traversal to find all descendants matching a selector in jQuery?
.find()
.search()
.descend()
.traverse()
Q58
Q58 Which method is used to set the HTML content of an element?
.html()
.setContent()
.setHTML()
.fill()
Q59
Q59 How do you attach a 'click' event to an element that exists now or in the future?
.live('click', function())
.bind('click', function())
.on('click', function())
.attach('click', function())
Q60
Q60 How can you add a CSS class to a set of selected elements in jQuery?
.addClass('new-class')
.pushClass('new-class')
.insertClass('new-class')
.applyClass('new-class')