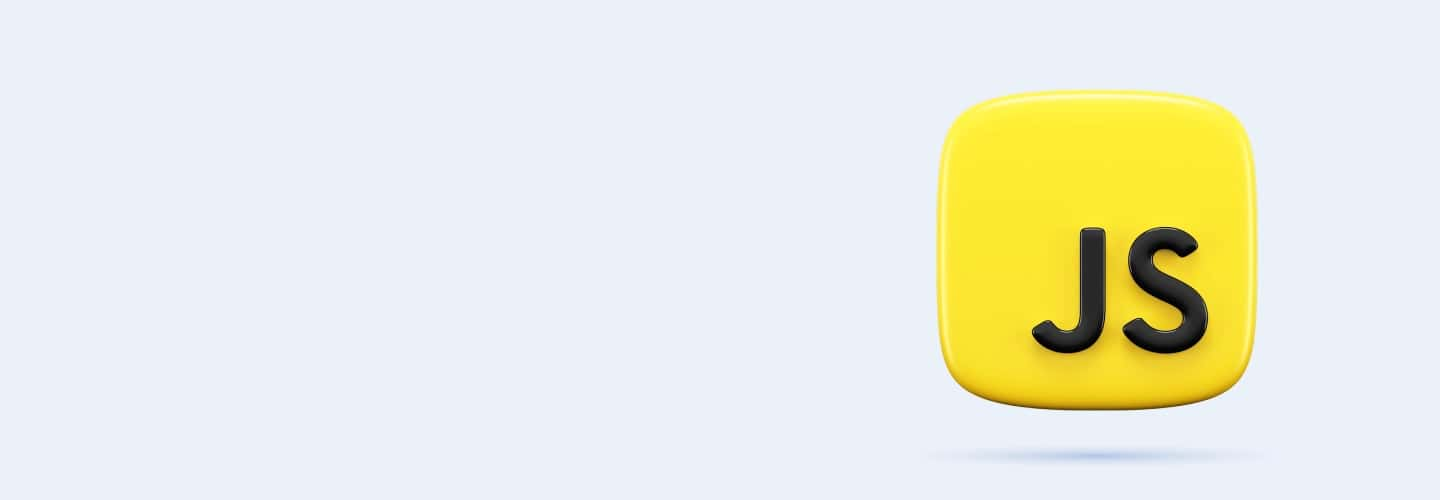
Q121
Q121 What is the problem with this async function?
async function getUser() {
let user = await fetch('/user');
if (user.id === 123) {
return 'Admin';
}
}
The function may return undefined
user.id is always undefined
The fetch request is incorrect
There is no error handling for the fetch
Q122
Q122 What is currying in JavaScript?
The process of breaking down a function into a series of functions that each take a single argument
Transforming a function to manage its state
A way of writing asynchronous code
Optimizing functions for performance
Q123
Q123 How is partial application different from currying?
Partial application is about fixing some arguments to a function, while currying is about breaking down a function into a series of functions that each take a single argument
Partial application is a technique for performance optimization, while currying is for error handling
Partial application deals with asynchronous functions, while currying deals with synchronous ones
There is no difference
Q124
Q124 What will the following curried function output?
function multiply(a) {
return function(b) {
return a * b;
};
}
let multiplyByTwo = multiply(2);
console.log(multiplyByTwo(3));
5
6
8
Syntax error
Q125
Q125 Consider this code:
function add(a) {
return function(b) {
return function(c) {
return a + b + c; };
};
} let addFive = add(2)(3);
console.log(addFive(5));
What is the output?
10
Syntax error
Undefined
15
Q126
Q126 Identify the issue in this partially applied function:
function log(date, importance, message) { console.log([${date.getHours()}:${date.getMinutes()}] [${importance}] ${message}); } let logNow = log.bind(null, new Date()); logNow('INFO', 'Server started');
Incorrect use of bind
Date is not updated correctly
Incorrect number of arguments provided
No error
Q127
Q127 Spot the error in this currying implementation:
function sum(a, b, c) {
return a + b + c;
}
let curriedSum = (a) => (b) => (c) => sum(a, b);
console.log(curriedSum(1)(2)(3));
Missing argument in the final function call
Incorrect return value in curriedSum
sum function is not needed
Syntax error
Q128
Q128 What is encapsulation in object-oriented programming?
The bundling of data and methods that operate on that data
Creating private variables within a class
The ability of an object to take on many forms
A design pattern that ensures only one instance of a class is created
Q129
Q129 In JavaScript, what is a prototype?
A blueprint for creating objects
A function that creates an object
An instance of a class
The object from which a function or object inherits properties
Q130
Q130 How does inheritance work in JavaScript?
Through classes and the extend keyword
Through the prototype chain
Through the use of constructor functions
Through global variables
Q131
Q131 What are the benefits of using ES6 classes over constructor functions?
ES6 classes are more performant
ES6 classes offer private methods and properties
ES6 classes provide a clearer, more concise syntax
Constructor functions do not allow inheritance
Q132
Q132 What is polymorphism in the context of object-oriented programming?
The ability to create multiple instances of a class
The ability of different classes to be treated as instances of the same class
The process of a function taking multiple forms
The ability of a class to inherit from multiple classes
Q133
Q133 What does this ES6 class syntax do?
class Animal {
constructor(name) {
this.name = name;
}
speak() {
console.log(${this.name} makes a noise.);
}
}
Defines an Animal class with methods for each animal
Creates an instance of the Animal class
Defines an Animal class with a constructor and a speak method
Declares a global function named Animal
Q134
Q134 Consider this code:
class Rectangle {
constructor(width, height) {
this.width = width;
this.height = height;
}
get area() {
return this.width * this.height;
}
} let rect = new Rectangle(5, 10);
console.log(rect.area);
What is the output?
15
50
'5x10'
Syntax error
Q135
Q135 What will the following inheritance code output?
class Animal {
speak() {
return 'I am an animal';
}
}
class Dog extends Animal {
speak() {
return super.speak() + ', but more specifically a dog';
}
}
let dog = new Dog();
console.log(dog.speak());
'I am an animal'
'I am an animal, but more specifically a dog'
'I am a dog'
Syntax error
Q136
Q136 Identify the mistake in this class definition:
class Person {
constructor(name) {
name = name;
}
}
The constructor doesn't properly set the property
The class should also have methods
Syntax error in class definition
No error
Q137
Q137 Spot the error in this class inheritance:
class Animal { constructor(name) {
this.name = name;
}
}
class Cat extends Animal {
constructor(name, lives) {
super();
this.lives = lives;
}
}
Missing name argument in the super call
Cat should not have additional properties
Syntax error in extending the class
No error
Q138
Q138 What is Cross-Site Scripting (XSS) in the context of web security?
Stealing cookies and session tokens
Injecting malicious scripts into webpages viewed by other users
Breaking into databases through the web interface
Disabling websites with Denial of Service attacks
Q139
Q139 What is Content Security Policy (CSP) and how does it help in web security?
A policy that restricts the types of content that can be loaded on a webpage to prevent XSS attacks
A server configuration that encrypts all data leaving a website
A JavaScript function that validates user input on a webpage
A protocol for secure communication between a website and its database
Q140
Q140 How can the following JavaScript code be a security risk?
document.write(userInput);
It can lead to XSS if userInput is not properly sanitized
It may slow down the website
It can overwrite important data
It can make the site non-responsive
Q141
Q141 What security issue does this code present, and how can it be mitigated?
let userDetails = JSON.parse(localStorage.getItem('userDetails'));
The code may execute malicious JavaScript from localStorage, which can be mitigated by validating and sanitizing the data before using it
The data might be outdated, leading to performance issues
The code can be blocked by browser security settings
The code exposes user details in the global scope
Q142
Q142 Identify the security flaw in this code snippet:
let username = document.querySelector('#username').value;
if (username.length < 3) {
alert('Username too short');
}
Potential Cross-Site Scripting vulnerability
Improper validation of the username length
Lack of encryption for the username
No security flaw
Q143
Q143 Spot the problem in this JavaScript security code:
fetch('https://api.example.com/data'
, {
credentials: 'include'
})
.then(response => response.json())
.then(data => console.log(data));
It allows Cross-Origin Resource Sharing (CORS) without proper checks
It sends credentials without checking the response type
There's no error handling for the fetch request
The data from the response is logged, which can be a security risk
Q144
Q144 What are localStorage and sessionStorage in web development?
Server-side storage mechanisms
Cookie-based storage mechanisms
Client-side storage mechanisms that store data locally in the user's browser
Database storage solutions provided by the browser
Q145
Q145 What is the main difference between localStorage and sessionStorage?
localStorage is larger in size than sessionStorage
localStorage persists data across browser sessions, while sessionStorage clears it when the session ends
sessionStorage is more secure than localStorage
localStorage can store complex data types, while sessionStorage cannot
Q146
Q146 How can web storage be made more secure against XSS attacks?
By encrypting the data stored in web storage
By using cookies instead of web storage
By storing only non-sensitive data in web storage
By limiting the size of the data stored
Q147
Q147 What does this code do?
localStorage.setItem('user', 'Alice');
console.log(localStorage.getItem('user'));
Retrieves a user's details from the server
Saves the user 'Alice' in the session storage and logs it
Saves the user 'Alice' in the local storage and logs it
Creates a cookie named 'user' with the value 'Alice'
Q148
Q148 Consider this web storage code:
sessionStorage.setItem('sessionData', JSON.stringify({ id: 123, token: 'abc' }));
let data = JSON.parse(sessionStorage.getItem('sessionData'));
console.log(data.id);
What is the output?
{ id: 123, token: 'abc' }
123
'abc'
undefined
Q149
Q149 What will be the result of this code if localStorage already has 10 items?
for (let i = 0; i < 5; i++) {
localStorage.setItem(key${i}, value${i});
}
console.log(localStorage.length);
5
10
15
Error
Q150
Q150 Identify the issue in this code snippet:
localStorage.setItem('settings', { theme: 'dark', layout: 'grid' });
let settings = localStorage.getItem('settings');
console.log(settings.theme);
The object is not correctly stored as a string
The localStorage API is misused
The settings object does not have a theme property
No error