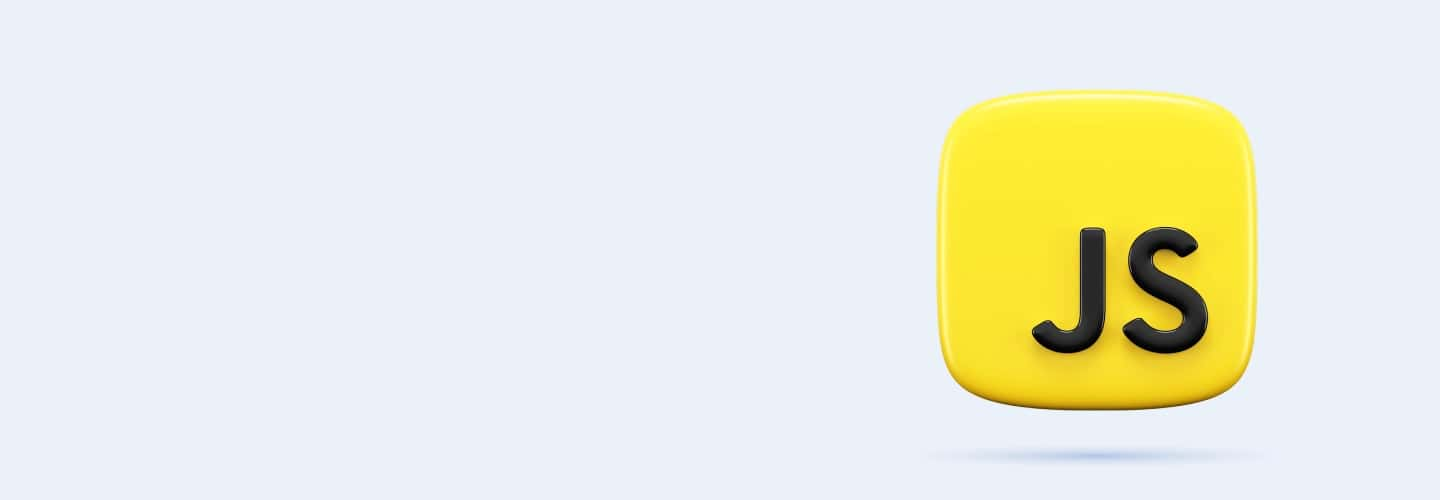
Q91
Q91 Find the mistake in this code:
try {
let x = 10;
if(x > 5) {
throw 'Too high';
}
} catch(e) {
console.log('Error: ', e);
}
Incorrect use of throw
x > 5 should be x < 5
Error in the catch block
No error
Q92
Q92 What is the problem with this code?
try { // code } catch(e) { console.log(e); }
There's no error to catch
The try block is empty
The catch block should have more logic
No error
Q93
Q93 Spot the error in this code:
try {
let obj = JSON.parse('{"name":"Alice"');
} catch(e) {
console.log('Parsing error:', e);
}
Missing closing brace in JSON string
Incorrect use of JSON.parse
Error in the catch block
No error
Q94
Q94 What is an arrow function in ES6?
A function that points to another function
A shorter syntax for writing function expressions
A function used for one-way data binding
A special function for asynchronous programming
Q95
Q95 What ES6 feature allows us to combine multiple strings and variables?
String interpolation
Template literals
Concatenation
Variable injection
Q96
Q96 What is the purpose of the let and const keywords in ES6?
To create global variables
To declare variables with block scope
To declare constants
Both B and C
Q97
Q97 How does the spread operator (...) work in ES6?
It divides an array into individual elements
It merges arrays or object properties
It spreads an object into multiple arrays
It is used for error handling
Q98
Q98 What does the following ES6 code output?
let [a, b] = [1, 2];
console.log(a);
1
2
[1, 2]
undefined
Q99
Q99 Consider the following ES6 code:
const square = x => x * x; console.log(square(4));
What is the output?
4
8
16
Syntax error
Q100
Q100 What will this ES6 code output?
function multiply(a, b = 2) {
return a * b;
}
console.log(multiply(3));
3
6
undefined
NaN
Q101
Q101 Find the mistake in this ES6 code:
let obj = { a: 1, b: 2, a: 3 }; console.log(obj.a);
Repeated property name in object literal
Syntax error in object declaration
Error in console.log statement
No error
Q102
Q102 What is a callback function in JavaScript?
A function that is scheduled to run after a set interval
A function that calls itself
A function passed into another function as an argument to be executed later
A function that runs immediately after declaration
Q103
Q103 What is "Callback Hell" in JavaScript?
A large number of nested callback functions that make the code hard to read and maintain
An error thrown by a failed callback function
A callback function that never gets executed
A standard method for handling asynchronous operations
Q104
Q104 How can "Callback Hell" be avoided in JavaScript?
Using promises and async/await
Increasing the response time of servers
Writing all code in a single callback function
Avoiding the use of callback functions altogether
Q105
Q105 What does the following code do?
function processData(callback) {
if (data) {
callback(null, data);
} else {
callback(new Error('No data'), null);
}
}
Throws an error if there's no data
Processes data asynchronously and executes the callback function
Logs data to the console
Creates a new data object
Q106
Q106 Consider this code:
function firstFunction(params, callback) {
// Do something, then callback()
}
function secondFunction(params) {
firstFunction(params, function(err, data) {
if (err) {
console.log('Error:', err);
} else {
console.log('Data:', data);
}
}); }
What pattern does this represent?
Callback pattern
Module pattern
Observer pattern
Singleton pattern
Q107
Q107 What is the output of this code? function doAsyncTask(cb) { setTimeout(() => { console.log('Async Task Calling Callback'); cb(); }, 1000); } doAsyncTask(() => console.log('Callback Called'));
'Async Task Calling Callback' then 'Callback Called' after 1 second
'Callback Called' then 'Async Task Calling Callback' after 1 second
'Async Task Calling Callback' and 'Callback Called' simultaneously
Nothing, it's an infinite loop
Q108
Q108 Spot the error in this callback usage:
function fetchData(callback) {
if (error) {
callback(error);
} return callback(null, data);
}
The callback is called twice
The data is returned before the callback is called
The error is not properly handled
There is no error
Q109
Q109 What is a Promise in JavaScript?
A function that executes asynchronously
A callback function that executes immediately
An object representing the eventual completion or failure of an asynchronous operation
A data structure for storing multiple callbacks
Q110
Q110 How does promise chaining work in JavaScript?
By returning a new promise from the callback of another promise
By executing multiple callbacks in parallel
By repeatedly calling the same promise
By automatically rejecting a promise after a set timeout
Q111
Q111 What happens if a promise is rejected and there is no .catch() block?
The error is silently ignored
The promise is fulfilled with an undefined value
A TypeError is thrown
An unhandled promise rejection occurs
Q112
Q112 What does the following code do?
new Promise((resolve, reject) => {
setTimeout(() => resolve('Result'), 2000);
})
.then(result => console.log(result));
Executes a function after 2 seconds
Logs 'Result' immediately
Creates a promise that resolves with 'Result' after 2 seconds, then logs it
Repeatedly logs 'Result' every 2 seconds
Q113
Q113 Consider this code:
new Promise((resolve, reject) => {
throw new Error('Error');
})
.catch(error => console.log(error.message))
.then(() => console.log('Completed'));
What will be the output?
'Error' then 'Completed'
Just 'Error'
Just 'Completed'
A runtime error
Q114
Q114 What is the output of this promise chain?
Promise.resolve(1)
.then((x) => x + 1)
.then((x) => {
throw new Error('My Error');
})
.catch(() => console.log('Caught'))
.then(() => console.log('Done'));
'Caught' then 'Done'
'My Error' then 'Done'
Just 'Caught'
Just 'Done'
Q115
Q115 Spot the error in this promise code:
let p = new Promise((resolve, reject) => {
resolve('Success');
reject('Failed');
}).then(result => console.log(result)).catch(error => console.log(error));
The promise should not call both resolve and reject
The .then() method is misplaced
The .catch() block is unnecessary
The promise does not handle asynchronous operations
Q116
Q116 What is the purpose of the async keyword in JavaScript?
To pause the execution of a function
To declare a function as asynchronous
To speed up a function
To handle multiple promises simultaneously
Q117
Q117 How does await work inside an async function?
It stops the entire program until the awaited promise resolves
It waits for a promise to resolve before continuing the execution of the async function
It sends a request to the server
It creates a new thread for asynchronous execution
Q118
Q118 What will the following async function output?
async function fetchData() {
let response = await fetch('https://api.example.com/data'
); let data = await response.json(); console.log(data);
} fetchData();
The contents of 'https://api.example.com/data'
A JavaScript object
An error message
Nothing
Q119
Q119 Consider this code:
async function checkData() {
try {
let response = await fetch('https://api.example.com/data'
);
if (!response.ok)
throw new Error('Error fetching data');
let data = await response.json();
return data;
} catch (error) {
console.error(error);
}
}
What happens when fetch fails?
It logs 'Error fetching data'
It returns 'Error fetching data'
It throws an error but doesn't catch it
It returns undefined
Q120
Q120 Identify the issue in this async function:
async function loadData() {
let data = await fetch('https://api.example.com'
).json();
console.log(data);
}
Incorrect use of await
Syntax error in fetch
No error handling for fetch failure
Missing async keyword