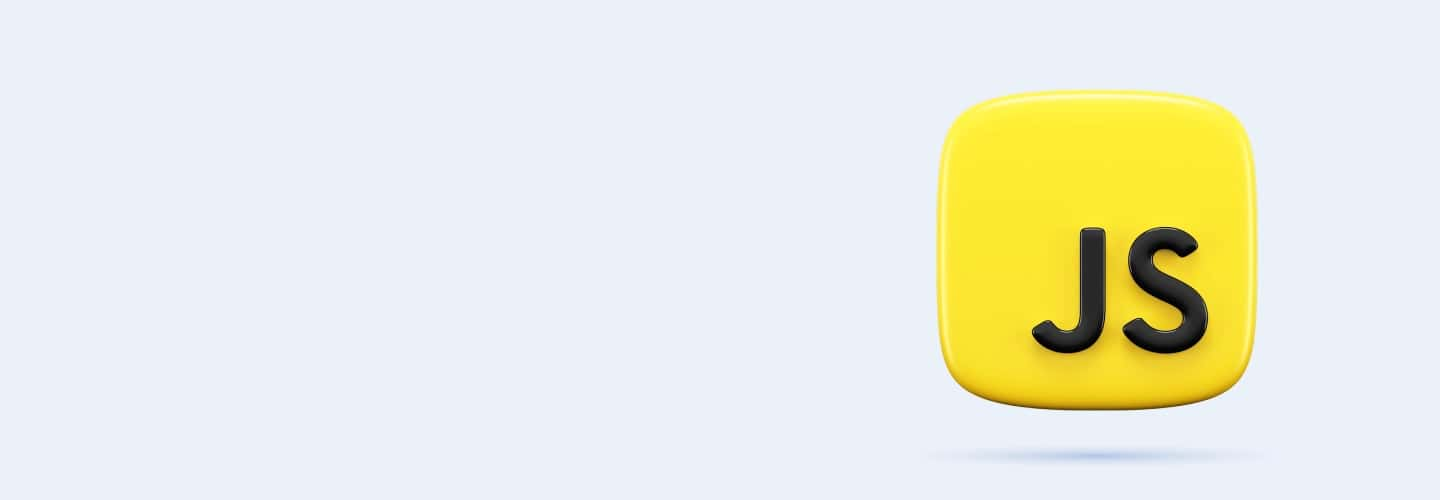
Q61
Q61 Identify the error in this code:
document.getElementByld('demo').innerText = 'Welcome';
Typo in method name
Missing element with id 'demo'
Incorrect property innerText
No error
Q62
Q62 Spot the mistake in this code:
let btn = document.getElementById('submit');
btn.onClick = function() {
console.log('Clicked');
};
Incorrect event handler
Missing element with id 'submit'
Syntax error in the function
No error
Q63
Q63 What is an event in the context of JavaScript?
A user action like a mouse click
A change in the state of the program
A server response
All of the above
Q64
Q64 Which method is commonly used to attach an event handler to an element in JavaScript?
addEventListener()
attachEvent()
onEvent()
bindEvent()
Q65
Q65 What is event bubbling in JavaScript?
When an event on a child element is also triggered on parent elements
When an event triggers a server request
When an event occurs repeatedly in a short period
When an event fails to trigger
Q66
Q66 Which of the following is not a mouse event in JavaScript?
onclick
onmouseover
onmousemove
onchange
Q67
Q67 How can you stop event propagation in JavaScript?
event.stop()
event.preventDefault()
event.stopPropagation()
event.pause()
Q68
Q68 What does the following JavaScript code do?
document.getElementById('btn').onclick = function() {
alert('Button clicked');
};
Displays an alert when a button is clicked
Changes the text of a button
Reloads the page
Does nothing
Q69
Q69 Consider the following code snippet:
element.addEventListener('click', function() {
console.log('Element clicked');
});
What does this code do?
Logs a message when the element is double-clicked
Changes the style of the element when clicked
Logs a message when the element is clicked
Submits a form when the element is clicked
Q70
Q70 What will be the result of this code if div1 is inside div2 in the HTML structure?
document.getElementById('div1').addEventListener('click', function(event) {
console.log('div1 clicked');
event.stopPropagation();
});
document.getElementById('div2').addEventListener('click', function() {
console.log('div2 clicked');
});
Only 'div1 clicked' will be logged
Only 'div2 clicked' will be logged
Both 'div1 clicked' and 'div2 clicked' will be logged
No message will be logged
Q71
Q71 Identify the error in this code:
document.getElementById('myBtn').onClick = function() {
console.log('Button clicked');
};
Incorrect event property
Syntax error
Logic error
No error
Q72
Q72 What is the issue with this code?
button.addEventListener('click', doSomething); function doSomething() {
alert('Clicked');
}
Missing event listener
doSomething is not defined
Incorrect event type
No error
Q73
Q73 What does AJAX stand for in web development?
Asynchronous JavaScript And XML
Automated JavaScript And XHTML
Advanced JavaScript And XML
Asynchronous Java And XML
Q74
Q74 What is the main advantage of using AJAX in a web application?
Faster server response time
Increased security
Reduced server load and asynchronous requests
Simpler coding requirements
Q75
Q75 What is the purpose of the Fetch API in JavaScript?
To send data to a server
To manipulate the DOM
To retrieve resources asynchronously over the network
To store data in the browser
Q76
Q76 What is the difference between the Fetch API and XMLHttpRequest?
Fetch can only send asynchronous requests
Fetch returns a promise, making it easier to work with asynchronous operations
XMLHttpRequest is faster than Fetch
Fetch cannot handle responses in JSON format
Q77
Q77 What does the following JavaScript code do?
fetch('https://api.example.com/data'
)
.then(response => response.json())
.then(data => console.log(data));
Sends data to 'https://api.example.com/data'
Requests data from 'https://api.example.com/data' and logs it
Creates a new resource at 'https://api.example.com/data'
Deletes data from 'https://api.example.com/data'
Q78
Q78 What will this Fetch API code snippet do?
fetch('https://api.example.com/update'
, {
method: 'POST',
body: JSON.stringify({name: 'Alice'})
})
.then(response => response.json())
.then(data => console.log(data));
Retrieves data from the URL
Updates data at the URL and logs the response
Deletes data at the URL
Submits data to the URL and logs the response
Q79
Q79 How does this code handle errors when using the Fetch API?
fetch('https://api.example.com/data'
)
.then(response => {
if (!response.ok) {
throw new Error('Network response was not ok');
}
return response.json();
})
.catch(error => console.error('Fetch error:', error));
It logs errors to the console
It redirects the user to an error page
It retries the fetch request
It sends an error report to the server
Q80
Q80 Identify the issue in this fetch request:
fetch('https://api.example.com/data'
, {
method: 'GET',
body: JSON.stringify({id: 1})
});
GET requests should not have a body
The URL is incorrect
The method should be POST
There is a syntax error
Q81
Q81 Spot the error in this code:
fetch('https://api.example.com/data'
)
.then(response => console.log(response.json()));
Improper use of response.json()
Missing catch for error handling
The URL is incorrect
There is a syntax error
Q82
Q82 What is the purpose of the try...catch statement in JavaScript?
To test code for errors
To speed up code execution
To declare variables
To loop through arrays
Q83
Q83 What type of error is thrown when an undefined variable is used in JavaScript?
SyntaxError
TypeError
ReferenceError
RangeError
Q84
Q84 Which statement is used to manually throw an exception in JavaScript?
throw
error
exception
raise
Q85
Q85 What does the finally block in a try...catch statement do?
It runs only if no errors occur
It runs after the try and catch blocks, regardless of the result
It runs as a cleanup process
It checks for any remaining errors
Q86
Q86 What is the difference between a SyntaxError and a ReferenceError in JavaScript?
A SyntaxError occurs for mistakes in the code's syntax, while a ReferenceError occurs for illegal or invalid references to variables
A SyntaxError occurs when variables are not found, while a ReferenceError occurs for syntax mistakes
A SyntaxError is a runtime error, while a ReferenceError is a compile-time error
No difference
Q87
Q87 What is the output of this code?
try {
console.log('Start');
throw new Error('An error occurred');
console.log('End');
} catch(e) {
console.log('Caught an error');
}
'Start' 'End' 'Caught an error'
'Start' 'Caught an error'
'Caught an error'
'Start' 'An error occurred'
Q88
Q88 What will this code output?
try {
let x = y;
} catch(e) {
console.log(typeof e);
}
'undefined'
'object'
'string'
'error'
Q89
Q89 What will happen when this code is executed?
try {
null.foo();
}
catch(e) {
console.log(e.name);
} finally {
console.log('Done');
}
Logs 'TypeError' and 'Done'
Logs 'ReferenceError' and 'Done'
Only logs 'Done'
Causes a fatal error
Q90
Q90 Identify the error in this code:
try {
let result = 'Result';
console.log(result);
} catch(e) {
console.log(e);
} finally {
console.log(reslt);
}
Misspelled variable in the finally block
Error in the try block
Error in the catch block
No error