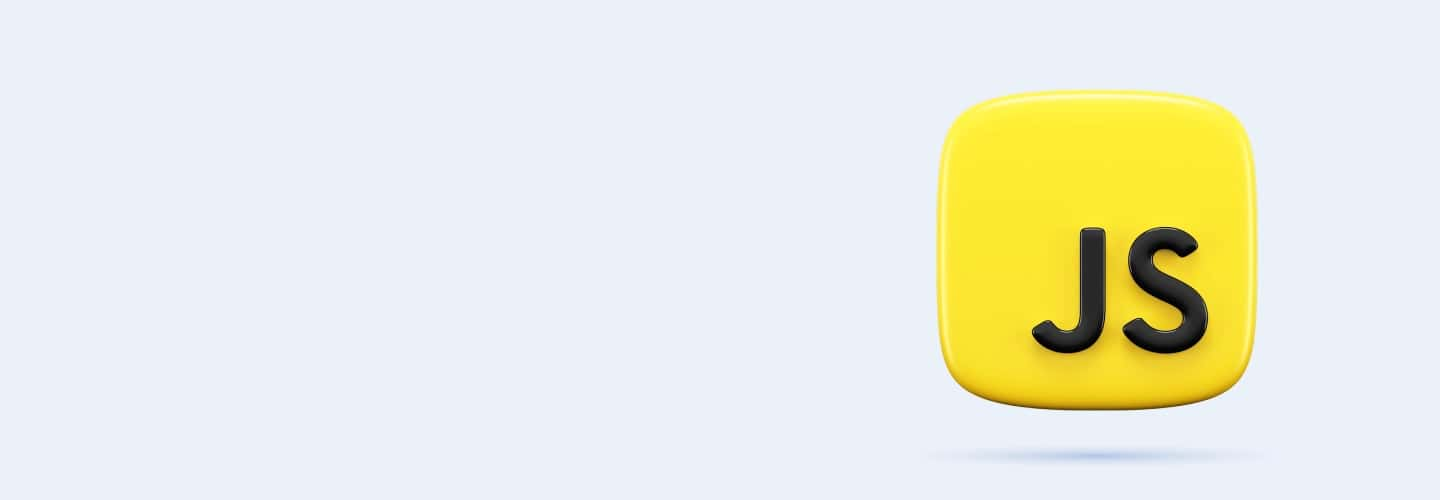
Q31
Q31 What is wrong with this function declaration?
function power(base, exponent) {
if (exponent == 0) return 1;
else return base * power(base, exponent - 1);
} console.log(power(2));
It doesn't handle the case when exponent is not provided
It returns the wrong value
It causes an infinite loop
Syntax error
Q32
Q32 Which method is used to add an element to the end of an array in JavaScript?
push()
unshift()
pop()
shift()
Q33
Q33 How do you find the length of an array in JavaScript?
array.size()
array.length
array.count()
length(array)
Q34
Q34 What does the splice method do in an array?
Copies a portion of an array
Concatenates arrays
Changes the content of an array
Finds an element in an array
Q35
Q35 In JavaScript, how can you check if a variable is an array?
typeof variable
variable.isArray()
Array.isArray(variable)
variable instanceof Array
Q36
Q36 Which of the following array methods in JavaScript does not change the original array?
sort()
splice()
forEach()
push()
Q37
Q37 What is the output of this code snippet?
let arr = [10, 20, 30, 40];
let newArr = arr.map(x => x / 10);
console.log(newArr);
[1, 2, 3, 4]
[10, 20, 30, 40]
[0.1, 0.2, 0.3, 0.4]
Error
Q38
Q38 What is the output of the following code?
let fruits = ['apple', 'banana', 'mango'];
console.log(fruits[1]);
apple
banana
mango
undefined
Q39
Q39 What will be the output of this code snippet?
let numbers = [1, 2, 3];
numbers[10] = 11;
console.log(numbers.length);
3
4
11
10
Q40
Q40 Consider the following code:
let arr = [1, 2, 3];
arr[5] = 5;
console.log(arr.filter(x => x === undefined).length);
What is the output?
0
2
3
5
Q41
Q41 Identify the issue in this array declaration:
let numbers = new Array(-5);
Negative size
Syntax error
Logical error
No error
Q42
Q42 Spot the mistake in this code snippet:
let data = [1, 2, 3];
delete data[1];
console.log(data[1]);
undefined is logged instead of 2
2 is not deleted
Syntax error
No error
Q43
Q43 How do you access the value of a property in a JavaScript object?
object{propertyName}
object[propertyName]
object.propertyName
Both B and C
Q44
Q44 What will be the output of console.log(typeof {})?
'object'
'array'
'null'
'undefined'
Q45
Q45 In JavaScript, what is a method?
A predefined function
A loop inside an object
A function stored as an object property
An external library function
Q46
Q46 How do you create a new object in JavaScript?
Object.create()
new Object()
Both A and B
Neither A nor B
Q47
Q47 What is the purpose of the this keyword in JavaScript objects?
Refer to the global object
Refer to the current object
Create a new object
Duplicate an object
Q48
Q48 What is the result of accessing a property that doesn’t exist on an object?
null
undefined
0
Error
Q49
Q49 What is the output of the following code snippet?
let obj = { a: 1, b: 2 };
console.log(obj.a);
1
2
undefined
Error
Q50
Q50 Consider the code:
let person = { name: 'Alice', age: 25 };
delete person.age; console.log(person.age);
What is the output?
'Alice'
25
undefined
Error
Q51
Q51 Given the following code, what is printed to the console?
let obj1 = { a: 1 };
let obj2 = { a: 1 };
console.log(obj1 === obj2);
true
false
null
Error
Q52
Q52 Find the issue in this object definition:
let obj = { '1a': 10, b: 20 };
console.log(obj.1a);
Invalid property name
Syntax error in console.log
Incorrect value assignment
No error
Q53
Q53 Identify the error in this object method:
let obj = {
greet: function() {
return 'Hello, ' + name;
}
};
console.log(obj.greet());
name is not defined
greet is not a function
Syntax error
No error
Q54
Q54 What does DOM stand for in web development?
Document Object Model
Data Object Management
Direct Object Manipulation
Display Object Model
Q55
Q55 Which JavaScript method is used to select an HTML element by its ID?
getElementById()
querySelector()
getElementsByClassName()
getElementsByTagName()
Q56
Q56 How can you change the text content of an HTML element in the DOM using JavaScript?
element.textContent = 'new text'
element.innerHTML = 'new text'
Both A and B
Neither A nor B
Q57
Q57 What is the difference between innerHTML and textContent properties in DOM manipulation?
innerHTML can include HTML tags; textContent cannot
textContent is faster than innerHTML
textContent can include HTML tags; innerHTML cannot
There is no difference
Q58
Q58 What is the output of this code if the HTML contains an element with id demo and text 'Hello'?
document.getElementById('demo').textContent = 'Hi'; console.log(document.getElementById('demo').textContent);
'Hello'
'Hi'
undefined
Error
Q59
Q59 Consider this code:
let items = document.querySelectorAll('.item');
console.log(items.length);
What does it output if there are three elements with class item in the HTML?
3
'item'
undefined
Error
Q60
Q60 What will be the result of the following code if the HTML has multiple p tags?
let paragraphs = document.getElementsByTagName('p');
for(let i = 0; i < paragraphs.length; i++) {
paragraphs[i].style.color = 'blue';
}
All p tags turn blue
Only the first p tag turns blue
A TypeError occurs
No change in p tags