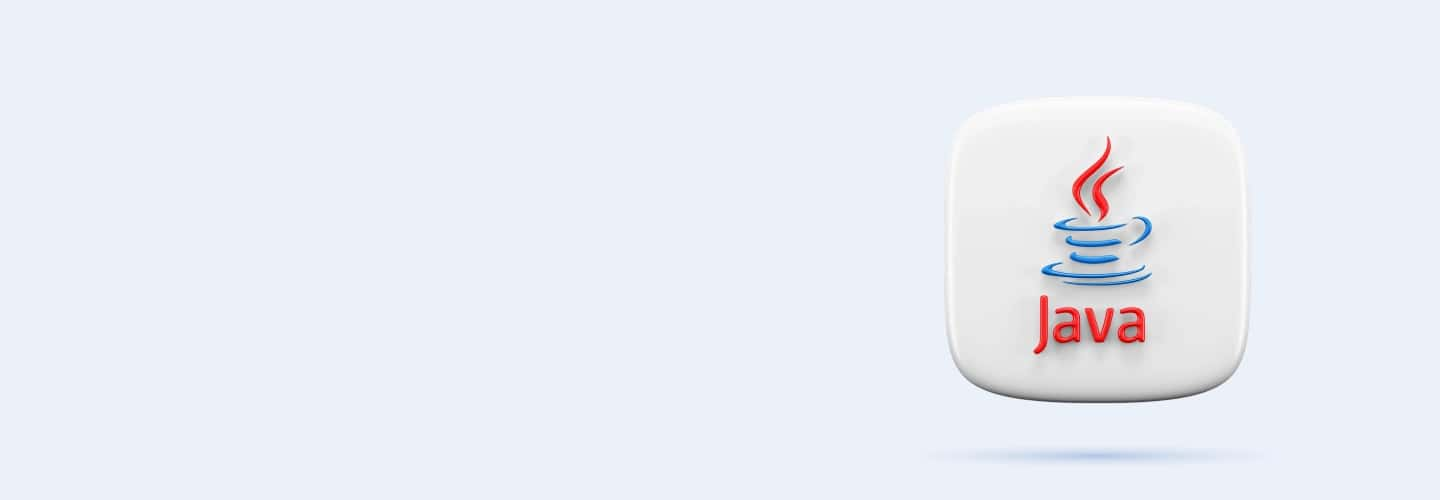
Q121
Q121 In Java, what happens when two string literals with the same content are created?
They refer to different objects in memory
They refer to the same object in the String Constant Pool
A runtime error occurs
A new object is created each time
Q122
Q122 What will this Java code output?
String s1 = "Java";
String s2 = "Java";
System.out.println(s1 == s2);
true
false
An error occurs
None of the above
Q123
Q123 What does this Java code do?
StringBuilder sb = new StringBuilder("Java");
sb.append(" Programming");
System.out.println(sb);
Appends " Programming" to sb and prints "Java Programming"
Creates a new StringBuilder object
Throws an error
Prints "Java"
Q124
Q124 Analyze the output of this code snippet:
StringBuffer buffer = new StringBuffer("Java");
buffer.reverse();
System.out.println(buffer);
avaJ
Java
A runtime error occurs
None of the above
Q125
Q125 What will this pseudocode output?
SET str = "Java" SET builder = StringBuilder(str) builder.append("Script") PRINT builder
JavaScript
Java
JavaScriptJava
Error
Q126
Q126 Analyze this pseudocode:
CREATE buffer = StringBuffer("Java") CALL buffer.reverse() PRINT buffer
avaJ
Java
A runtime error occurs
None of the above
Q127
Q127 What will be the result of this pseudocode?
SET str1 = "Java" SET str2 = "Java" IF str1 EQUALS str2 PRINT "Equal" ELSE PRINT "Not Equal"
Equal
Not Equal
Error
None
Q128
Q128 Identify the issue in this code snippet:
StringBuilder sb = "Java"; sb.append("Script");
Incorrect initialization of StringBuilder
No issue
append method used incorrectly
Syntax error
Q129
Q129 Spot the mistake:
StringBuffer buffer = new StringBuffer("Java");
buffer.setLength(2);
System.out.println(buffer);
It will print "Ja"
The setLength method is used incorrectly
No error
Syntax error
Q130
Q130 Find the error in this Java code:
String s1 = new String("Java");
String s2 = new String("Java");
System.out.println(s1 == s2);
s1 and s2 refer to the same object
No error
s1 and s2 refer to different objects
Syntax error
Q131
Q131 What is the main difference between a single-threaded and a multi-threaded application?
Single-threaded applications can perform multiple tasks at once
Multi-threaded applications are always faster
Single-threaded applications use less memory
Multi-threaded applications can perform multiple tasks at the same time
Q132
Q132 In Java, what is the difference between a user thread and a daemon thread?
User threads are low priority, daemon threads are high priority
User threads run in the background, daemon threads do not
User threads prevent the JVM from exiting, daemon threads do not
Daemon threads are used for garbage collection
Q133
Q133 What is the primary purpose of the wait() and notify() methods in Java?
To start and stop threads
To manage thread priorities
To handle synchronization between threads
To handle exceptions in threads
Q134
Q134 Analyze the output of this code snippet:
class MyThread extends Thread { public void run() {
for(int i = 0;
i < 5;
i++) {
System.out.println(i); }
}
} public class Main {
public static void main(String[] args) {
MyThread t = new MyThread();
t.start();
for(int i = 5;
i < 10;
i++) {
System.out.println(i); }
}
}
The numbers 0 to 9 in sequential order
The numbers 5 to 9 followed by 0 to 4
A mix of numbers 0 to 9 in no particular order
A runtime error
Q135
Q135 What will this pseudocode output?
CREATE thread START thread PRINT "Thread started" END
Thread started
An error
Nothing
Thread started and then an error
Q136
Q136 Analyze this pseudocode:
CREATE thread1, thread2 START thread1 CALL thread1.wait() START thread2 CALL thread2.notify()
thread2 starts after thread1 waits
thread1 and thread2 run concurrently
thread1 waits indefinitely
An error occurs
Q137
Q137 Identify the issue in this code snippet:
class MyThread extends Thread {
public void run() {
wait(); }
}
Incorrect use of wait()
Syntax error
No issue
Incorrect thread initialization
Q138
Q138 Spot the mistake:
synchronized void myMethod() { notify(); wait(); }
wait() should be called before notify()
notify() and wait() cannot be in the same method
No error
The method is not properly synchronized
Q139
Q139 What is a lambda expression in Java?
A type of exception
A new collection type
A concise way to represent an anonymous function
A data storage format
Q140
Q140 What is the purpose of the Streams API in Java 8?
To handle I/O streams
To create a new type of collection
To enable functional-style operations on collections
To manage threads
Q141
Q141 What are functional interfaces in Java?
Interfaces with only static methods
Interfaces with multiple abstract methods
Interfaces with a single abstract method
Interfaces that support lambda expressions only
Q142
Q142 How does the Optional class help in Java?
It replaces all null values in a program
It is used to avoid NullPointerExceptions
It provides a better way of handling multithreading
It optimizes memory usage
Q143
Q143 What is the advantage of using method references in Java 8?
They make the code run faster
They make the code more readable when referring to methods or constructors
They replace all lambda expressions
They are necessary for functional programming
Q144
Q144 How does the Date and Time API in Java 8 improve over the older java.util.Date class?
It provides more methods for date manipulation
It is thread-safe and immutable
It supports internationalization better
All of the above
Q145
Q145 What is the purpose of the CompletableFuture class in Java?
To simplify multithreading programming
To replace the use of raw threads
To provide a more complex future interface
To handle synchronous programming
Q146
Q146 In Java, what are the benefits of using Callable and Future interfaces?
To handle synchronization between threads
To perform operations in the background and retrieve their results
To handle exceptions in multithreaded code
To manage thread lifecycle
Q147
Q147 Analyze the output of this code snippet:
List
asList(1, 2, 3);
numbers.stream().
map(n -> n * n).
forEach(System.out::println);
Prints the squares of the numbers
Prints the numbers multiplied by 2
Prints the sum of the numbers
Prints the numbers as they are
Q148
Q148 What will this pseudocode output?
CREATE list = [1, 2, 3, 4] CREATE stream = list.stream() FILTER stream WITH (n -> n > 2) PRINT stream
[1, 2, 3, 4]
[3, 4]
[1, 2]
Empty list
Q149
Q149 What is the primary purpose of the Locale class in Java?
To handle currency conversions
To manage different time zones
To represent a specific geographical, political, or cultural region
To change the layout of graphical components
Q150
Q150 What is a key consideration when designing an application to support multiple languages?
Ensuring all text is in English and translated later
Using non-localized date and time formats
Using external files for text to facilitate easy translation
Hardcoding all strings in the application