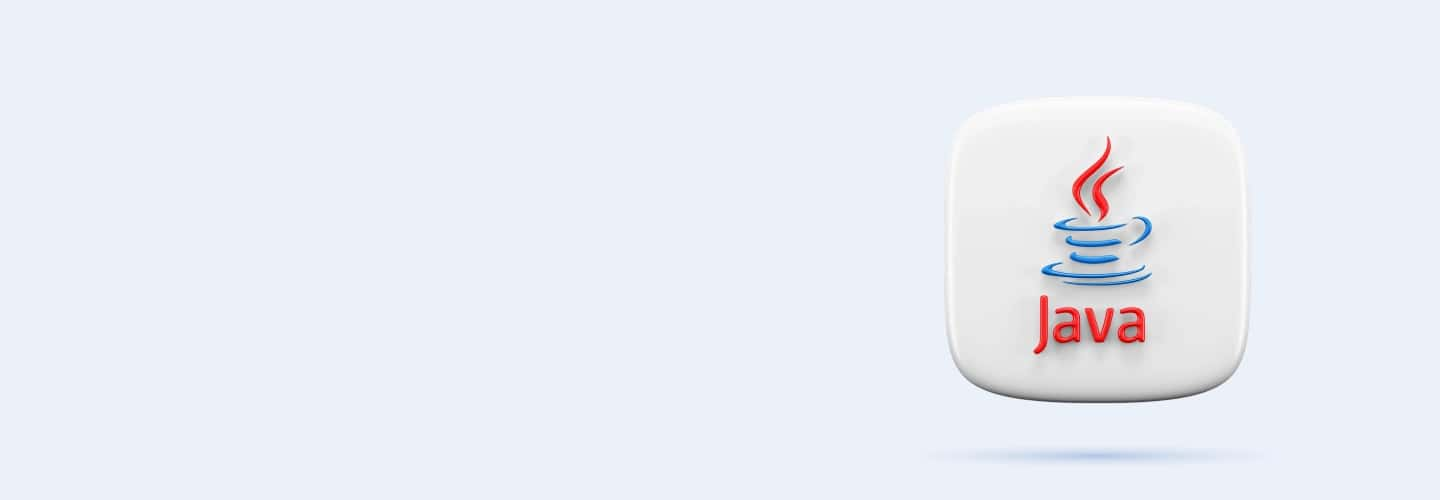
Q91
Q91 Determine the output of this pseudocode:
TRY PRINT "Start" THROW NEW EXCEPTION "Failed" CATCH EXCEPTION PRINT "Caught" END TRY PRINT "End"
Start Caught End
Start Failed
Caught
Start Caught
Q92
Q92 What will be the result of this pseudocode?
SET arr = [1, 2, 3]
TRY
PRINT arr[3]
CATCH EXCEPTION
PRINT "Array Error" END TRY
1 2 3
Array Error
Error
None
Q93
Q93 Identify the issue in this code snippet:
try {
int x = 5 / 0;
}
catch (Exception e) {
e.printStackTrace();
}
finally {
System.out.println("Done");
}
Division by zero
No error
Missing throw statement
Syntax error
Q94
Q94 Spot the mistake:
try {
String s = null;
System.out.println(s.length());
}
catch (NullPointerException e) {
System.out.println("Null Error");
}
No error
Null Error should not be caught
s should be initialized
The length() method cannot be used on strings
Q95
Q95 Find the error in this Java code:
try {
File file = new File("test.txt");
FileReader fr = new FileReader(file);
}
catch (FileNotFoundException e) {
System.out.println("File not found");
}
File not found
No error
Missing import statements for File and FileReader
Syntax error
Q96
Q96 Identify the flaw in this Java code:
class CustomException extends Exception { }
try {
throw new CustomException();
}
catch (CustomException e) {
System.out.println("Custom exception caught");
}
CustomException should not be caught
CustomException should extend RuntimeException
Missing try block
No error
Q97
Q97 What are generics in Java?
Templates for creating collections
Special methods in Java
Memory allocation techniques
Data types in Java
Q98
Q98 What is the primary advantage of using generics in Java?
Faster execution
Reduced memory usage
Stronger type checking at compile-time
Easier code maintenance
Q99
Q99 Which collection type does not allow duplicate elements?
ArrayList
Vector
Set
List
Q100
Q100 What is the difference between a HashMap and a Hashtable in Java?
HashMap is synchronized, Hashtable is not
Hashtable is synchronized, HashMap is not
HashMap allows one null key, Hashtable does not
All of the above
Q101
Q101 In Java Collections Framework, which interface represents a mapping from unique keys to values?
List
Set
Map
Queue
Q102
Q102 What happens when a duplicate key is put into a HashMap?
It replaces the existing key’s value
It is ignored
It throws an exception
A new entry is created
Q103
Q103 What is the difference between the add() and put() methods in Java's Collection Framework?
add() is for lists, put() is for maps
add() inserts at a specific index, put() does not
add() is for sets, put() is for lists
There is no difference
Q104
Q104 Which of the following is true about a TreeMap in Java?
It does not maintain an order
It is not based on the Map interface
It orders elements based on natural ordering or a comparator
It allows null keys
Q105
Q105 What does this Java code output?
Map<Integer, String> map = new HashMap<>();
map.put(1, "Java");
map.put(2, "Python");
System.out.println(map.size());
1
2
3
None
Q106
Q106 Analyze the output of this code snippet:
List
list.add("Java");
list.add("Java");
System.out.println(list.get(1));
Java
Error
null
None
Q107
Q107 What will this Java code print?
Set
set.add(1); set.add(1);
System.out.println(set.size());
1
2
0
Error
Q108
Q108 What is the result of executing this code?
Map<String, Integer> map = new TreeMap<>();
map.put("Python", 3);
map.put("Java", 1);
System.out.println(map);
{Java=1, Python=3}
{Python=3, Java=1}
{1=Java, 3=Python}
Error
Q109
Q109 Determine the output of this pseudocode:
CREATE list = ["Java", "Python", "Java"] FOR EACH element IN list PRINT element
Java Python Java
Java Python
Java
Python Java
Q110
Q110 What will be the result of this pseudocode?
CREATE map SET map["Java"] = 1 SET map["Python"] = 2 SET map["Java"] = 3 PRINT map["Java"]
1
2
3
Error
Q111
Q111 Analyze this pseudocode:
CREATE set ADD "Java" TO set ADD "Python" TO set ADD "Java" TO set IF "Java" IN set PRINT "Yes" ELSE PRINT "No"
Yes
No
Error
None
Q112
Q112 What will this pseudocode output?
CREATE map SET map[1] = "Java" SET map[2] = "Python" SET map[1] = "C++" PRINT map[1]
Java
Python
C++
Error
Q113
Q113 Identify the issue in this code snippet:
List
The list is empty
The get method is used incorrectly
The list should be a LinkedList
No error
Q114
Q114 Spot the mistake:
Set
TreeSet does not allow null
No error
Set should be HashSet
Syntax error
Q115
Q115 Find the error in this Java code:
Map<String, Integer> map = new HashMap<>();
map.put(null, 1);
map.put(null, 2);
System.out.println(map.get(null));
HashMap does not allow null keys
Prints 1
No error
Syntax error
Q116
Q116 Identify the flaw in this Java code:
List
The remove index is out of bounds
LinkedList does not allow insertion at an index
No error
Syntax error
Q117
Q117 What is the String Constant Pool in Java?
A collection of all String literals
A memory area for constant String storage
A pool for reusable String objects
All of the above
Q118
Q118 How does StringBuilder differ from StringBuffer in Java?
StringBuilder is immutable, StringBuffer is not
StringBuilder is faster as it is not synchronized
StringBuilder and StringBuffer have different methods
There is no difference
Q119
Q119 What is the result of concatenating strings using the '+' operator in Java?
A new String object is created every time
The original string is modified
No new objects are created
A StringBuilder object is used internally
Q120
Q120 Why is it recommended to use StringBuilder or StringBuffer for string manipulation in loops?
To reduce memory usage
To increase execution speed
To avoid creating many intermediate String objects
Both B and C