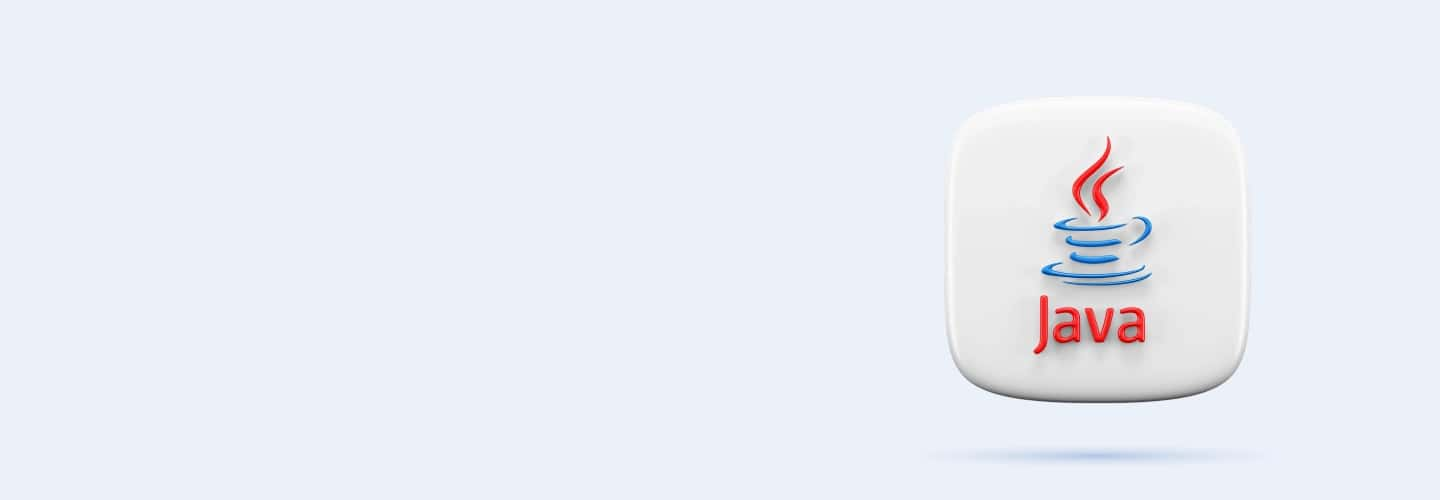
Q61
Q61 What will the following code output:
class Test {
static int x = 10;
} public class Main {
public static void main(String[] args) {
Test t1 = new Test(); System.out.println(t1.x); } }
10
0
Error
Null
Q62
Q62 Analyze the output of this code snippet:
class Car {
String model; Car(String model) { this.model = model;
}
} public class Main {
public static void main(String[] args) { Car myCar = new Car("Tesla"); System.out.println(myCar.model); } }
Tesla
null
Error
Model
Q63
Q63 What does this Java code do?
class Animal {
void sound() {
System.out.println("Generic Sound");
}
} class Dog extends Animal { void sound() { System.out.println("Bark"); } } public class Main { public static void main(String[] args) { Animal myAnimal = new Dog(); myAnimal.sound(); } }
Prints "Generic Sound"
Prints "Bark"
Error
Does nothing
Q64
Q64 What will this pseudocode output?
CLASS Vehicle SET wheels = 4 METHOD showWheels PRINT wheels END CLASS Main CREATE Vehicle v CALL v.showWheels
4
0
Error
None
Q65
Q65 Determine the output of this pseudocode:
CLASS Animal METHOD sound PRINT "Generic Sound" END CLASS Dog EXTENDS Animal METHOD sound PRINT "Bark" END CLASS Main CREATE Dog d CALL d.sound
Generic Sound
Bark
Error
None
Q66
Q66 What will be the result of this pseudocode?
CLASS Book VARIABLE title = "Java Programming" METHOD getTitle PRINT title END CLASS Main CREATE Book b CALL b.getTitle
Java Programming
null
Error
Title
Q67
Q67 Analyze this pseudocode:
CLASS Shape METHOD area RETURN 0 END CLASS Circle EXTENDS Shape VARIABLE radius = 5 METHOD area RETURN 3.14 * radius * radius END CLASS Main CREATE Circle c PRINT c.area
0
78.5
Error
None
Q68
Q68 Identify the issue in this code snippet:
class Book {
private String title; void setTitle(String title) {
title = title; }
}
The method does not set the instance variable
No issue
Syntax error
Logic error
Q69
Q69 Spot the mistake:
class Calculator {
static void sum(int a, int b) {
System.out.println(a + b);
}
public static void main(String[] args) {
sum(10, 20); }
}
No static context for sum
No error
sum method should return a value
Incorrect parameters
Q70
Q70 Find the error in this Java code:
class Animal {
Animal() {
System.out.println("An animal is created");
}
} class Dog extends Animal {
Dog() { super();
System.out.println("A dog is created");
}
} public class Main {
public static void main(String[] args) {
Dog dog = new Dog();
}
}
Incorrect use of super()
No error
Constructor not called
Syntax error
Q71
Q71 What is an abstract class in Java?
A class that cannot be instantiated and has at least one abstract method
A class with only static methods
A final class
A class that cannot be extended
Q72
Q72 How does StringBuilder differ from StringBuffer in Java?
StringBuilder is synchronized; StringBuffer is not
StringBuilder is faster as it is not synchronized
StringBuilder and StringBuffer have different methods
StringBuilder allows storing strings of variable length
Q73
Q73 In Java, what is an interface?
A fully abstract class
A regular class
A concrete class
A template class
Q74
Q74 Can an interface in Java contain default methods?
Yes, from Java 8 onwards
No, interfaces cannot have default methods
Only in abstract classes
Only static methods are allowed
Q75
Q75 What happens when a class implements an interface in Java?
It must provide implementation for all methods in the interface
It becomes an abstract class
It can no longer extend other classes
It automatically inherits from java.lang.Object
Q76
Q76 Can an interface in Java extend multiple interfaces?
Yes
No
Only if they are marker interfaces
Only abstract classes can extend multiple interfaces
Q77
Q77 What will this Java code output?
interface Printable {
void print();
}
class Test implements Printable { public void print() {
System.out.println("Hello");
} }
public class Main {
public static void main(String[] args) {
Test t = new Test();
t.print(); }
}
Hello
Error
Nothing
Printable
Q78
Q78 Analyze the output of this code snippet:
interface A {
int val = 5;
}
interface B extends A {
int val = 10;
}
class Test implements B {
void display() {
System.out.println(val);
}
} public class Main {
public static void main(String[] args) {
Test t = new Test();
t.display();
}
}
5
10
Error
None
Q79
Q79 What is the primary purpose of a package in Java?
To provide network support
To optimize code performance
To group related classes and interfaces
To manage software versions
Q80
Q80 What does the 'import' statement do in a Java program?
It includes native libraries
It enhances performance
It allows access to classes in packages
It compiles the Java code
Q81
Q81 How do you access a class from a package in Java?
By using the 'package' keyword before the class name
By importing the class
By inheriting the class
By implementing an interface
Q82
Q82 What is the significance of the CLASSPATH environment variable in Java?
It specifies the installation directory of Java
It sets the maximum memory allocation for the Java Virtual Machine
It tells the JVM where to look for user-defined classes and packages
It configures the security settings of the JVM
Q83
Q83 What is an exception in Java?
An error during program execution
A type of Java class
A method declaration
A user input error
Q84
Q84 What is the purpose of a try-catch block in Java?
To handle exceptions
To optimize code
To declare variables
To control program flow
Q85
Q85 What happens if an exception is thrown in a try block and is not caught in the corresponding catch block?
The exception is ignored
The program terminates
The exception is handled by the default handler
The program continues execution
Q86
Q86 Which keyword is used to manually throw an exception in Java?
throw
throws
try
catch
Q87
Q87 What is the difference between checked and unchecked exceptions in Java?
Checked exceptions are detected at compile-time, unchecked at runtime
Checked exceptions are fatal, unchecked are not
Checked exceptions are errors in the code, unchecked are system errors
There is no difference
Q88
Q88 What will the following code output:
try {
int a = 5 / 0;
System.out.println(a);
}
catch (ArithmeticException e) {
System.out.println("Arithmetic Error");
}
Arithmetic Error
5
Error
None
Q89
Q89 Analyze the output of this code snippet:
try {
int[] arr = new int[5];
arr[10] = 100;
System.out.println("Value set");
}
catch (ArrayIndexOutOfBoundsException e) {
System.out.println("Index Error");
}
Value set
Index Error
Error
None
Q90
Q90 What will this pseudocode output?
SET a = 10
SET b = 0
TRY
SET c = a / b
CATCH EXCEPTION PRINT "Error" END TRY
Error
10
0
None