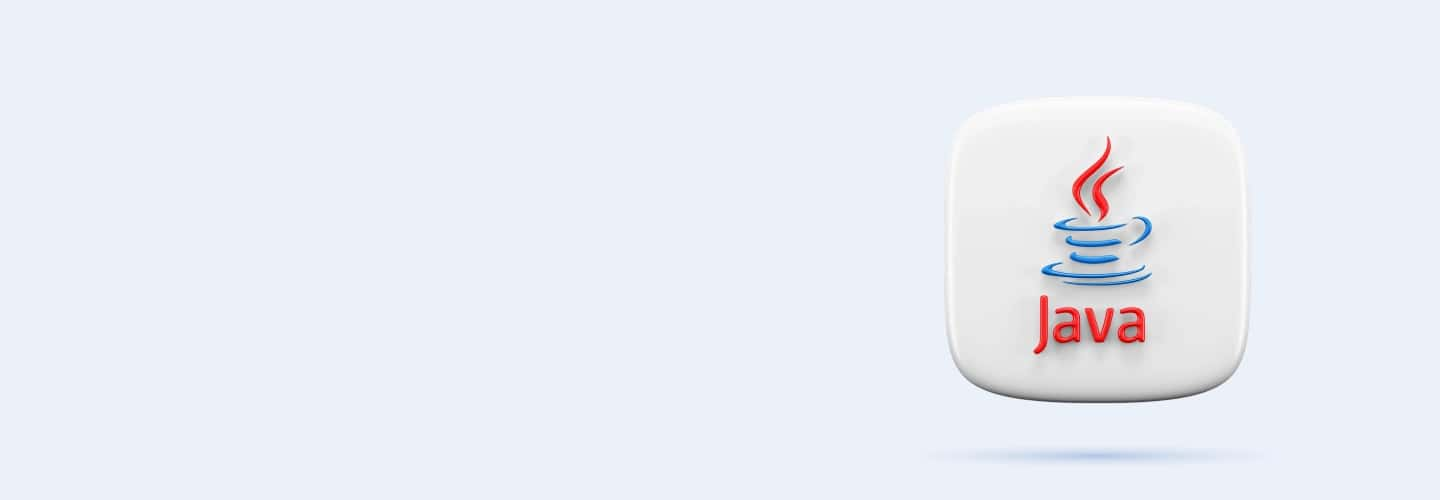
Q31
Q31 Find the error in this code:
int num = 1;
do{
System.out.println(num);
num++;
}
while(num <= 3);
System.out.println(num);
Infinite loop
Syntax error
Prints wrong values
No error
Q32
Q32 Which class is commonly used for simple keyboard input in Java?
Scanner
InputStream
BufferedReader
FileReader
Q33
Q33 In Java, what is the default value of an array of integers?
0
null
1
-1
Q34
Q34 What does 'BufferedReader' in Java provide that 'Scanner' does not?
Faster input reading
Input from file only
Different data types
Graphical interface
Q35
Q35 How do you access the third element in an array named 'arr'?
arr[2]
arr[3]
arr(2)
arr(3)
Q36
Q36 What will happen if you try to access an index outside the bounds of an array in Java?
Compile-time error
Runtime error: ArrayIndexOutOfBoundsException
No error
Logical error
Q37
Q37 What is the purpose of the 'length' attribute in a Java array?
To determine the size of the array
To modify the size of the array
To sort the array
To initialize the array
Q38
Q38 Which of these is not a valid way to instantiate an array in Java?
int[] arr = new int[5];
int arr[] = new int[5];
int arr[] = {1, 2, 3, 4, 5};
int arr[] = int[5];
Q39
Q39 In a multi-dimensional array, how do you access the element in the second row and third column of an array named 'matrix'?
matrix[1][2]
matrix[2][3]
matrix[2][2]
matrix[1][3]
Q40
Q40 What will the following code output:
int[] arr = {1, 2, 3};
for(int num : arr) {
System.out.println(num);
}
1 2 3
123
Error
None
Q41
Q41 What does this Java code do?
int[][] arr = {{1, 2}, {3, 4}};
for(int i = 0;
i < arr.length;
i++) {
for(int j = 0;
j < arr[i].length;
j++) {
System.out.print(arr[i][j] + " "); } System.out.println();
}
Prints a 2D array in matrix form
Prints only the first row of the array
Prints the sum of the array elements
Gives an error
Q42
Q42 What will be the result of executing this code snippet?
String[] names = {"Java", "Python", "C++"};
System.out.println(names[1].length());
4
5
6
Error
Q43
Q43 Analyze this code:
int[] nums = new int[3];
nums[1] = 10;
int x = nums[1] + nums[0];
System.out.println(x);
0
10
Error
None
Q44
Q44 What will this pseudocode output?
SET arr = [1, 2, 3] FOR EACH num IN arr PRINT num
1 2 3
123
Error
None
Q45
Q45 Determine the output of this pseudocode:
SET arr = [10, 20, 30]
SET sum = 0
FOR i = 0 TO LENGTH(arr) - 1
INCREMENT sum BY arr[i]
PRINT sum
60
10 20 30
Error
None
Q46
Q46 What will be the result of this pseudocode?
SET matrix = [[1, 2], [3, 4]]
PRINT matrix[0][1]
1
2
3
4
Q47
Q47 Identify the issue in this code snippet:
int[] numbers = new int[5];
for(int i = 0;
i <= numbers.length;
i++) {
System.out.println(numbers[i]);
}
Out of bounds array access
No issue
Syntax error
Logic error
Q48
Q48 Spot the mistake in this code:
String[] names = {"Java", "Python", "C"};
for(int i = 0;
i < names.length;
i++) {
System.out.println(names[i].length());
}
Prints incorrect lengths
No error
Syntax error
Logic error
Q49
Q49 Find the error in this Java code:
int[][] matrix = new int[2][2];
matrix[0][0] = 1;
matrix[0][1] = 2;
matrix[1][0] = 3;
System.out.println(matrix[1][1]);
Prints incorrect value
No error
Syntax error
Missing initialization for matrix[1][1]
Q50
Q50 Identify the flaw in this Java code:
char[] chars = new char[-1];
Negative array size
Syntax error
No error
Logic error
Q51
Q51 What is the primary feature of Object-Oriented Programming in Java?
Inheritance
Abstraction
Encapsulation
Polymorphism
Q52
Q52 Which of these is not a principle of Object-Oriented Programming?
Inheritance
Polymorphism
Compilation
Abstraction
Q53
Q53 What does 'inheritance' in Java imply?
A class can call methods of another class
A class shares structure and behaviors from another class
A class must override all methods of another class
A class is a blueprint for objects
Q54
Q54 What is an instance variable in Java?
A variable defined inside a method
A variable defined outside of any method but inside a class
A static variable
A final variable
Q55
Q55 Which keyword is used for inheritance in Java?
extends
implements
inherits
super
Q56
Q56 What is the purpose of a constructor in Java?
To create an instance of a class
To initialize variables
To define methods
To declare classes
Q57
Q57 In Java, how is a 'static' variable different from an 'instance' variable?
Static variables are shared across all instances of a class
Static variables are reinitialized with each object instance
Instance variables are shared across all instances of a class
Instance variables are constants
Q58
Q58 What is polymorphism in Java?
The ability of a variable to hold different data types
The ability of a class to implement multiple methods with the same name
The ability of a method to perform different tasks based on the context
The ability of a class to extend multiple classes
Q59
Q59 What distinguishes an abstract class from a regular class in Java?
An abstract class cannot create objects
An abstract class only contains abstract methods
An abstract class cannot contain constructors
An abstract class cannot have instance variables
Q60
Q60 Which access modifier in Java makes a member accessible only within its own class?
private
public
protected
default