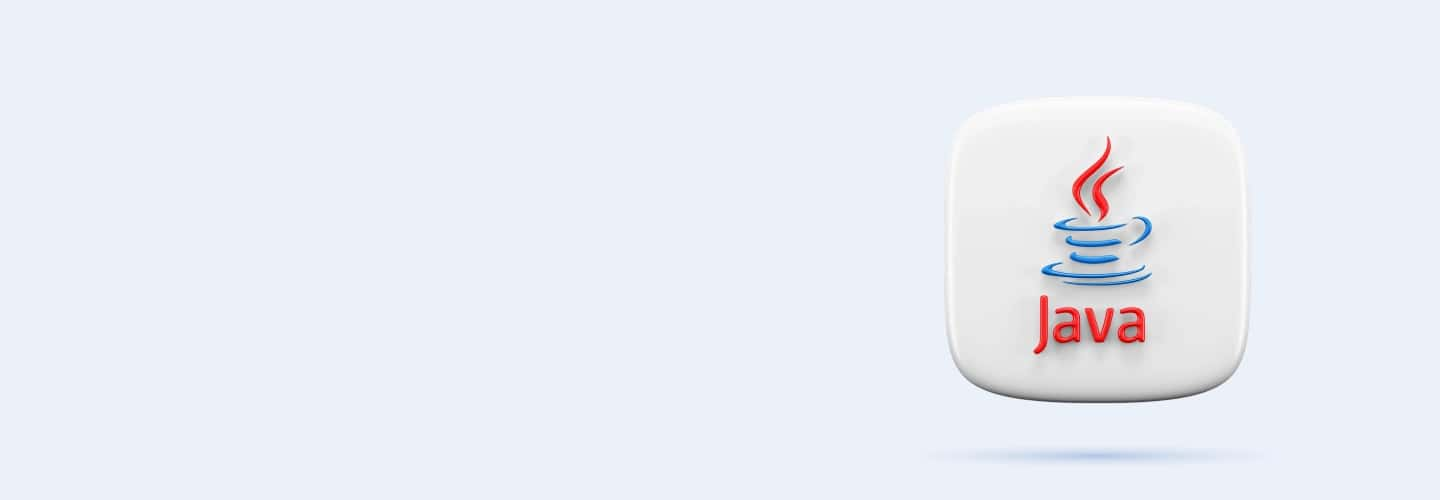
Q1
Q1 Which of these is not a feature of Java?
Object-oriented
Platform-independent
Compiled
Interpreted language
Q2
Q2 Which component of Java is responsible for running the compiled Java bytecode?
JDK
JVM
JRE
JIT
Q3
Q3 What is the purpose of the PATH environment variable in Java?
To locate Java libraries
To store Java bytecode
To locate the Java compiler
To optimize Java code
Q4
Q4 Which feature of Java makes it possible to run a Java program on different platforms?
Object-Oriented
Platform-Independent
Syntax
Memory Management
Q5
Q5 In Java, how should class names be written?
camelCase
snake_case
PascalCase
kebab-case
Q6
Q6 Which of these is a single-line comment in Java?
Q7
Q7 Which data type would be best for storing a person's age in Java?
int
double
long
byte
Q8
Q8 What is the default value of a boolean variable in Java?
true
false
0
null
Q9
Q9 What is the result of this operation in Java:
(int)(7.9)?
7
7.9
8
Syntax Error
Q10
Q10 Which keyword is used to define a constant variable in Java?
final
static
const
immutable
Q11
Q11 What is the range of the short data type in Java?
-32768 to 32767
-128 to 127
-2147483648 to 2147483647
0 to 65535
Q12
Q12 What will be the output of the following code snippet:
int a = 10;
int b = 20;
System.out.println(a + b);
10
20
30
Error
Q13
Q13 Identify the output of this code:
boolean isJavaFun = true;
System.out.println(!isJavaFun);
true
false
Error
null
Q14
Q14 What does the following Java code print?
int x = 5;
int y = x++;
System.out.println(y);
5
6
Syntax Error
None of the above
Q15
Q15 What is the output of this pseudocode?
SET x = 10
IF x > 5
THEN PRINT "Greater"
ELSE PRINT "Lesser"
Greater
Lesser
Error
No output
Q16
Q16 Evaluate this pseudocode:
SET a = 3 SET b = 4 PRINT a * b
7
12
Error
None of the above
Q17
Q17 Determine the output:
SET num = 8
IF num MOD 2 = 0
THEN PRINT "Even"
ELSE
PRINT "Odd"
Even
Odd
Error
No output
Q18
Q18 Identify the error in this code:
int[] nums = new int[2]; nums[0] = 1; nums[1] = 2; nums[2] = 3;
Array index out of bounds
Incorrect array declaration
No error
Syntax error
Q19
Q19 Spot the mistake in this code snippet:
int i = 0;
while(i < 10) {
i++;
}
System.out.println(i);
Infinite loop
Syntax error
No error
Prints 0
Q20
Q20 Which control structure is used to execute a block of code multiple times?
if
switch-case
for
try-catch
Q21
Q21 What will be the output of the following code snippet:
if(false){
System.out.println("True");
}
else{
System.out.println("False");
}
True
False
Error
No Output
Q22
Q22 In a 'switch-case' statement, what is the role of the 'break' keyword?
To pause the execution
To terminate the case block
To skip to the next case
To repeat the case block
Q23
Q23 What is the difference between 'while' and 'do-while' loops in Java?
Syntax only
The 'do-while' loop executes at least once
Execution speed
No difference
Q24
Q24 Which keyword is used to exit a loop prematurely in Java?
break
continue
exit
stop
Q25
Q25 What will the following loop print?
for(int i = 0;
i < 3;
i++){
System.out.println(i);
}
0 1 2
1 2 3
0 1 2 3
None
Q26
Q26 What is the output of this code?
int x = 1;
while(x < 4){
System.out.println(x); x++;
}
1 2 3
2 3 4
1 2 3 4
1
Q27
Q27 What will this pseudocode output?
SET count = 5 DO PRINT count COUNTDOWN count
5 4 3 2 1
5
1 2 3 4 5
None
Q28
Q28 Analyze this pseudocode:
SET num = 0 WHILE num <= 5 IF num MOD 2 = 0 THEN PRINT num END IF INCREMENT num
0 2 4
0 1 2 3 4 5
2 4
0 2 4 6
Q29
Q29 Identify the error in this code:
for(int i=0;
i<=5;
i++) {
System.out.println(i);
}
System.out.println(i);
Syntax error outside loop
No error
Variable i not accessible outside loop
Infinite loop
Q30
Q30 Spot the mistake:
int counter = 0;
while(counter < 5){
counter++;
}
System.out.println("Count: " + counter);
Loop never terminates
Counter not incremented
No error
Print statement incorrect