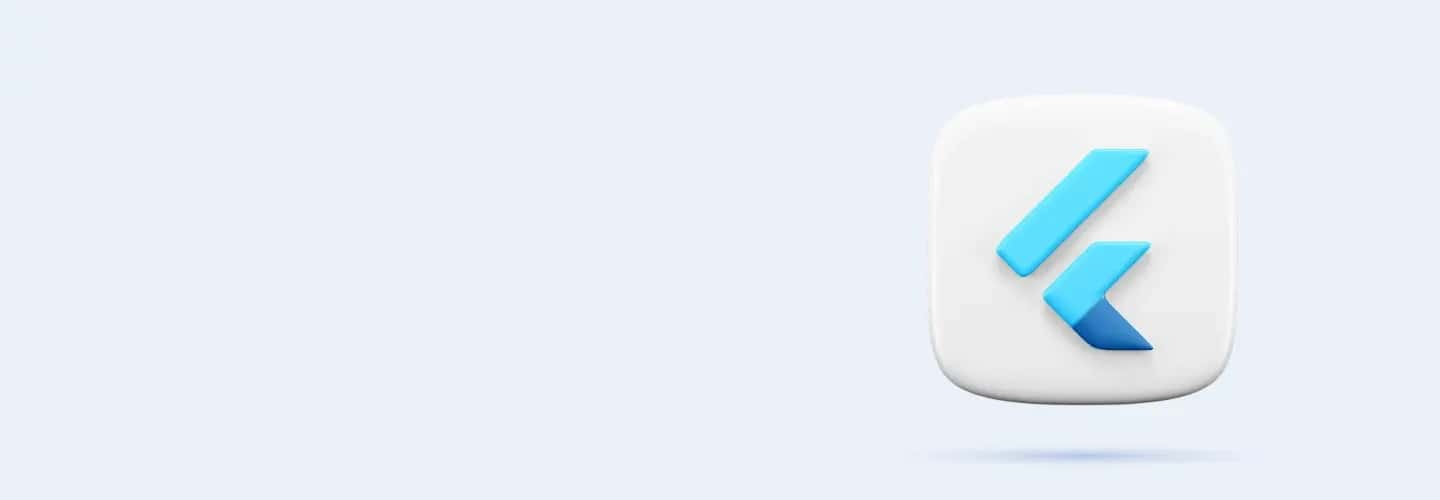
Q121
Q121 What is the role of the expect function in Flutter tests?
To compare actual and expected results
To render the widget
To initialize the test environment
To create mock data
Q122
Q122 What does the following code test?
\n\n\ntestWidgets('MyWidget has a title and message', (WidgetTester tester) async {
\n await tester.pumpWidget(MyWidget());
\n final titleFinder = find.text('Title');
\n final messageFinder = find.text('Message');
\n expect(titleFinder, findsOneWidget);
\n expect(messageFinder, findsOneWidget);
\n});\n
It tests the widget's layout
It tests the widget's interaction
It tests for the presence of text widgets
It tests for the widget's state
Q123
Q123 How would you test a button tap that triggers a state change?
\n\n\ntestWidgets('Button tap increments counter', (WidgetTester tester) async {
\n await tester.pumpWidget(MyApp());
\n await tester.tap(find.byType(ElevatedButton));
\n await tester.pump();
\n expect(find.text('1'), findsOneWidget);
\n});\n
It tests the button's color
It tests the button's visibility
It tests the state change after button tap
It tests the button's size
Q124
Q124 What could be a reason for a widget test failing unexpectedly?
Incorrect widget key
Missing dependencies
Incorrect test setup
All of the above
Q125
Q125 How can you debug a failing Flutter test that involves asynchronous operations?
Use print statements
Use debugPrint
Use expectAsync
Use await and async
Q126
Q126 What approach can you take to isolate and identify the cause of a test failure in Flutter?
Remove all expect statements
Test the app manually
Run the test in verbose mode
Break the test into smaller tests
Q127
Q127 Which Flutter tool is used for debugging applications?
Flutter Doctor
Flutter Analyzer
Flutter Debugger
Flutter Inspector
Q128
Q128 What does the flutter analyze command do?
Compiles the code
Runs the application
Analyzes the code for issues
Deploys the application
Q129
Q129 How does the FlutterError class help in error handling?
It logs errors to a file
It displays errors in the console
It provides custom error messages
It helps in exception tracking
Q130
Q130 What is the output of the following code if an exception is thrown?
\n\n\ntry {
\n throw Exception('Error');
\n} catch (e) {
\n print('Caught: $e');
\n}\n
Uncaught error
Exception: Error
Caught: Exception: Error
Error thrown
Q131
Q131 How would you handle an asynchronous error in Flutter?
\n\n\nFuture
\n try {\n var data = await fetchFromApi();
\n } catch (e) {
\n print('Error: $e');
\n }
\n}\n
Using try-catch
Using Future.error
Using async-catch
Using then-catch
Q132
Q132 What is a common reason for a widget not updating in Flutter?
Incorrect state management
Syntax error
Missing dependency
Outdated Flutter version
Q133
Q133 How can you debug a performance issue in Flutter applications?
Use Flutter Doctor
Use Flutter Analyzer
Use Flutter Profiler
Use Flutter Inspector
Q134
Q134 What should you do if you encounter a StateError in your Flutter app?
Restart the app
Check the widget state lifecycle
Update the Flutter SDK
Rebuild the widget tree
Q135
Q135 What is the purpose of using the RepaintBoundary widget in Flutter?
To prevent widget rebuilds
To isolate parts of the widget tree for performance optimization
To handle user input
To improve layout performance
Q136
Q136 How does the const keyword help in optimizing Flutter performance?
It reduces widget size
It prevents widget rebuilds
It simplifies widget structure
It enhances animation performance
Q137
Q137 What is the impact of using setState too frequently in Flutter?
Improves performance
Reduces performance
Has no effect
Increases battery usage
Q138
Q138 How can you optimize a list with a large number of items in Flutter?
\n\n\nListView.builder(
\n itemCount: 1000,
\n itemBuilder: (context, index) {
\n return ListTile(
\n title: Text('Item $index'),
\n );\n
},
\n);\n
Use ListView
Use ListView.builder
Use ListView.custom
Use ListView.separated
Q139
Q139 How would you optimize a widget that builds complex layouts frequently?
\n\n\nclass MyWidget extends StatelessWidget {
\n @override
\n Widget build(BuildContext context) {
\n return ComplexLayoutWidget();
\n }
\n}\n
Use StatefulWidget
Use StatelessWidget
Use Memoization
Use RepaintBoundary
Q140
Q140 What tool can you use to profile performance in a Flutter application?
Flutter Doctor
Flutter Analyzer
Flutter Profiler
Flutter Inspector
Q141
Q141 How can you identify widgets that are rebuilding unnecessarily in Flutter?
Use the debugPrint function
Use the shouldRebuild method
Use the Debug flags
Use the Widget rebuild profiler
Q142
Q142 What could be a reason for jank (stuttering) in a Flutter app animation?
Overdraw in the UI
Optimized layout
Efficient state management
Using stateless widgets
Q143
Q143 What is the first step in preparing a Flutter app for release on the Google Play Store?
Enable ProGuard
Configure app signing
Change app version
Optimize images
Q144
Q144 What file must be configured to set the app's version number and build number in Flutter?
pubspec.yaml
build.gradle
AndroidManifest.xml
MainActivity.java
Q145
Q145 Which tool is used to create a release build for iOS in Flutter?
flutter run
flutter build ios
flutter deploy
flutter release
Q146
Q146 How do you create a signed APK for Android in Flutter?
\n\n\nflutter build apk --release --split-per-abi\n
Using flutter run command
Using flutter build apk command
Using flutter deploy command
Using flutter release command
Q147
Q147 What is the purpose of the following code in the build.gradle file?
\n\n\nsigningConfigs {
\n release {
\n keyAlias 'my-key-alias'
\n keyPassword 'my-key-password'
\n storeFile file('my-key.jks')
\n storePassword 'my-store-password'
\n }
\n}\n
It configures ProGuard
It configures app signing
It configures versioning
It configures dependencies
Q148
Q148 What could be a reason for a Flutter app crash only in release mode?
Debug mode configurations
Missing release configurations
Incorrect widget tree
Using const keywords
Q149
Q149 How can you debug a Flutter app that works in debug mode but fails in release mode?
Check debug output
Check release logs
Check dependencies
Check widget tree
Q150
Q150 Which technique can help minimize the size of a Flutter app for deployment?
Enabling ProGuard
Using minification
Removing unused resources
Using compressed images