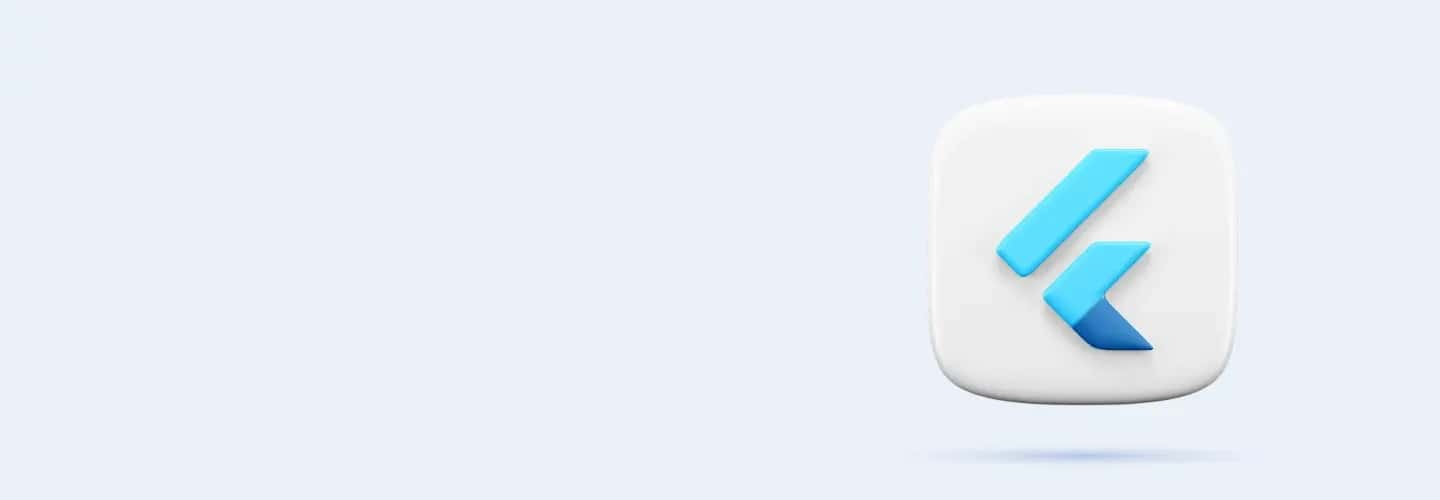
Q91
Q91 What method is used to send a GET request in the http package?
http.send
http.get
http.post
http.fetch
Q92
Q92 What is the purpose of the async and await keywords in Dart?
To handle synchronous operations
To handle asynchronous operations
To define variables
To create classes
Q93
Q93 How do you parse a JSON response in Flutter?
Using the jsonDecode function
Using the jsonEncode function
Using the JSON.parse function
Using the parse function
Q94
Q94 What is the output of the following code?
\n\n\nvar url = 'https://api.example.com/data';
\nvar response = await http.get(url);
\nprint(response.body);\n
Prints the response body
Prints the response headers
Prints the response status code
Throws an error
Q95
Q95 How would you handle an HTTP POST request in Flutter?
\n\n\nvar url = 'https://api.example.com/data';
\nvar response = await http.post(url, body: {'key': 'value'});\n
Using the http.get method
Using the http.post method
Using the http.send method
Using the http.fetch method
Q96
Q96 How do you handle errors when making an HTTP request in Flutter?
\n\n\ntry {
\n var response = await http.get(url);
\n if (response.statusCode == 200) {
\n print('Success');
\n } else {
\n print('Error: ${response.statusCode}');
\n }
\n} catch (e) {
\n print('Exception: $e');
\n}\n
Ignore errors
Check response status code and catch exceptions
Only check response body
Use a different package
Q97
Q97 What could be a reason for a network request failing in Flutter?
Incorrect URL
Valid URL
Strong internet connection
Valid API key
Q98
Q98 How can you debug a network issue where the response is not as expected in Flutter?
Check the request URL
Check the response headers
Check the request and response payloads
All of the above
Q99
Q99 Which package is commonly used for SQLite database integration in Flutter?
sqflite
moor
firebase
realm
Q100
Q100 What method is used to open a database connection in the sqflite package?
openDatabase
connectDatabase
initDatabase
startDatabase
Q101
Q101 How do you define a table schema in SQLite for Flutter?
Using SQL commands
Using Dart classes
Using JSON
Using XML
Q102
Q102 Which of the following is a benefit of using an ORM (Object-Relational Mapping) like moor?
Direct SQL queries
Automatic schema updates
Manual data handling
Low-level database access
Q103
Q103 What is the output of the following code?
\n\n\nDatabase db = await openDatabase('my_db.db');
\nawait db.insert('my_table', {'name': 'John', 'age': 30});\n
Inserts data into 'my_table'
Deletes data from 'my_table'
Updates data in 'my_table'
Creates 'my_table'
Q104
Q104 How would you query all rows from a table named 'users' in SQLite using sqflite?
\n\n\nDatabase db = await openDatabase('my_db.db');
\nList
Using db.select method
Using db.fetch method
Using db.query method
Using db.get method
Q105
Q105 How do you update a specific row in a table using sqflite?
\n\n\nawait db.update(
'users',
{'name': 'John'},
where: 'id = ?',
whereArgs: [1]
);\n
Using db.modify method
Using db.update method
Using db.edit method
Using db.change method
Q106
Q106 What could be a reason for a database operation failing in Flutter?
Incorrect table name
Valid query
Proper database connection
Correct SQL syntax
Q107
Q107 What could be a reason for a database operation failing in Flutter even if the query is correct?
Incorrect table name
Database not opened
Incorrect SQL syntax
Concurrency issues
Q108
Q108 What class in Flutter is used to create basic animations?
AnimationController
Animation
Tween
AnimatedBuilder
Q109
Q109 Which widget in Flutter is used to animate the transition between two widgets?
AnimatedBuilder
AnimatedContainer
AnimatedSwitcher
AnimatedOpacity
Q110
Q110 How does the Tween class work in Flutter animations?
It defines the start and end values of an animation
It controls the animation duration
It defines the animation curve
It handles user input
Q111
Q111 What is the role of the TickerProvider in Flutter animations?
It provides a way to create animations
It provides a way to sync animations to the screen refresh rate
It provides a way to handle user input
It provides a way to control animation duration
Q112
Q112 What does the CurvedAnimation class do in Flutter?
It handles the animation duration
It defines the start and end values
It applies a non-linear curve to an animation
It creates a linear animation
Q113
Q113 What is the purpose of the following code snippet?
\n\n\nAnimationController controller = AnimationController(
duration: const Duration(seconds: 2),
vsync: this
);
\nAnimation
begin: 0.0,
end: 1.0
).
animate(controller);\n
It creates a delayed animation
It creates a repeating animation
It creates an animation with a duration of 2 seconds
It creates an animation with a delay of 2 seconds
Q114
Q114 What does the AnimatedBuilder widget do in the following code?
\n\n\nAnimatedBuilder(
\n animation: animation,
\n builder: (context, child) {
\n return Transform.scale(
\n scale: animation.value,
\n child: child,
\n );
\n },
\n child: FlutterLogo(),
\n);\n
It creates a scaling animation
It creates a fading animation
It creates a rotating animation
It creates a color-changing animation
Q115
Q115 What could be a reason for an animation not starting in Flutter?
Incorrect animation duration
Animation controller not initialized
Animation listener not added
Incorrect animation curve
Q116
Q116 How can you debug an animation that is not running smoothly in Flutter?
Check the animation curve
Check the device's performance
Check for unnecessary rebuilds
All of the above
Q117
Q117 What package is commonly used for writing tests in Flutter?
flutter_test
flutter_testing
test_flutter
flutter_dev
Q118
Q118 Which of the following is used to create a widget test in Flutter?
testWidgets
testWidget
widgetTest
unitTest
Q119
Q119 What is the primary purpose of the pumpWidget method in widget testing?
To render a widget
To test widget properties
To simulate user interaction
To create mock data
Q120
Q120 How can you simulate a tap gesture in a Flutter widget test?
By using the pump method
By using the tap method
By using the tester.tap method
By using the simulateTap method