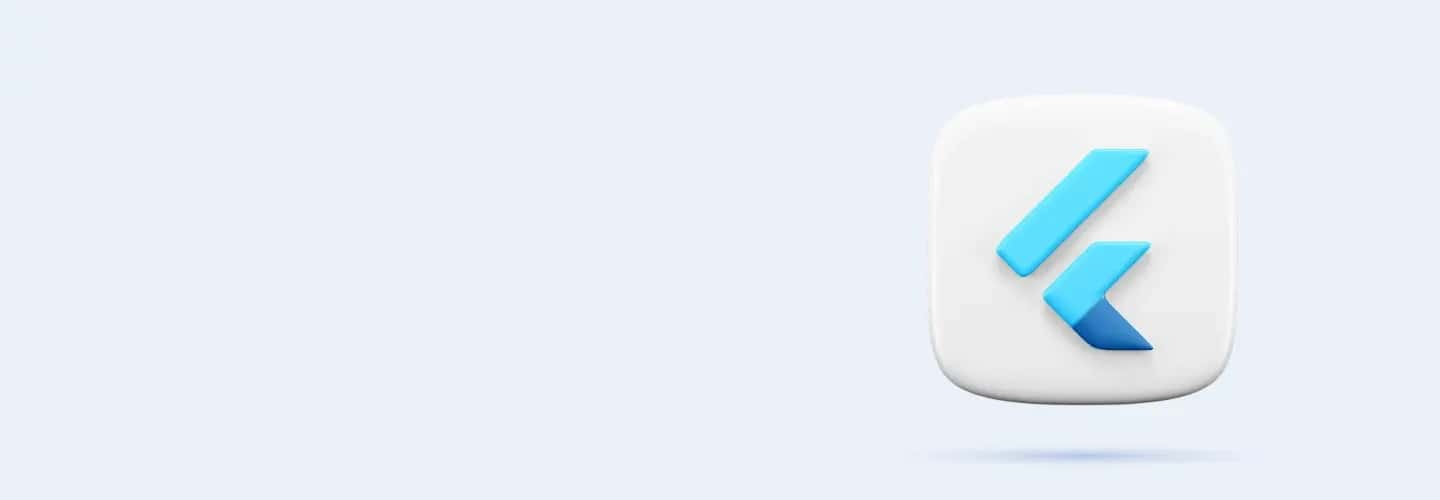
Q61
Q61 How does the ChangeNotifier class in Flutter work for state management?
It re-renders the entire widget tree
It notifies listeners when a change occurs
It prevents state changes
It provides a local state
Q62
Q62 Which of the following is a disadvantage of using setState for state management?
It is difficult to implement
It is not scalable for large applications
It doesn't support state changes
It doesn't work with StatelessWidgets
Q63
Q63 What is the output of the following code?
\n\n\nclass MyWidget extends StatefulWidget {
\n @override\n _MyWidgetState createState() => _MyWidgetState();\n}\n\nclass _MyWidgetState extends State
0
1
Counter increments by 1 on button press
Counter decrements by 1 on button press
Q64
Q64 How would you implement a Provider for state management in Flutter?
Using a StatefulWidget
Using a StatelessWidget
Using an InheritedWidget
Using a ChangeNotifierProvider
Q65
Q65 What does the following code snippet demonstrate?
\n\n\nclass Counter with ChangeNotifier {
\n int _count = 0;
\n int get count => _count;\n void increment() {
\n _count++;\n notifyListeners();
\n }
\n}\n
Basic state management
Advanced state management
State management using ChangeNotifier
State management using Bloc
Q66
Q66 What is a common issue when using setState that prevents the UI from updating?
Not calling setState inside the build method
Calling setState inside the build method
Not calling setState at all
Calling setState with no state change
Q67
Q67 How would you debug a state management issue where the state is not updating as expected?
Rebuild the app
Use print statements to trace state changes
Ignore the issue
Remove the stateful widget
Q68
Q68 Why might a ChangeNotifier not notify its listeners even when notifyListeners is called?
The ChangeNotifier is not initialized
Listeners are not registered properly
There is no state change
The app is in debug mode
Q69
Q69 Which widget is used to define routes in a Flutter application?
Navigator
Routes
MaterialApp
Scaffold
Q70
Q70 What does the Navigator.push method do in Flutter?
Removes the current route
Adds a new route to the stack
Replaces the current route
Closes the current route
Q71
Q71 How can you remove the current route in Flutter?
Navigator.pop
Navigator.push
Navigator.remove
Navigator.replace
Q72
Q72 What is the purpose of the onGenerateRoute property in the MaterialApp widget?
To handle route errors
To define a default route
To dynamically generate routes
To set the initial route
Q73
Q73 Which navigation method would you use to replace the current route with a new route?
Navigator.push
Navigator.pop
Navigator.pushReplacement
Navigator.add
Q74
Q74 What is the result of the following code?
\n\n\nNavigator.push(
\n context,\n MaterialPageRoute(builder: (context) => NewScreen()),
\n);\n
Navigates to NewScreen
Replaces current screen with NewScreen
Removes the current screen
Closes the app
Q75
Q75 How would you navigate back to the previous screen in Flutter?
\n\n\nNavigator.of(context).???;\n
push
pop
replace
pushReplacement
Q76
Q76 What does the following code do?
\n\n\nNavigator.pushAndRemoveUntil(
\n context,
\n MaterialPageRoute(builder: (context) => HomeScreen()),\n (Route
Adds a route to the stack
Removes all previous routes and navigates to HomeScreen
Replaces the current route
Navigates back to the initial route
Q77
Q77 What could be a reason for a navigation error stating "No MaterialLocalizations found"?
Missing Navigator widget
Missing MaterialApp widget
Incorrect route name
Missing Scaffold widget
Q78
Q78 How can you debug a routing issue where a screen is not found in Flutter?
Check for missing Navigator
Check for missing routes in MaterialApp
Check for missing Scaffold
Check for missing context
Q79
Q79 What could cause a "Route not found" error even if the route is defined in the MaterialApp?
Incorrect route name
Incorrect widget used
Navigator not initialized
Context is null
Q80
Q80 Which widget is commonly used to handle form input in Flutter?
TextField
Form
InputForm
FormField
Q81
Q81 How do you validate a form in Flutter?
Using a Validator object
Using the validate method on the form key
Using the checkValidity method
Using the validate attribute
Q82
Q82 What is the purpose of a GlobalKey in a form?
To uniquely identify a form and access its state
To style the form
To handle user input
To create a new form
Q83
Q83 How can you reset the state of a form in Flutter?
By setting the form fields to null
Using the reset method on the form key
Rebuilding the form widget
Using the clear method on the form key
Q84
Q84 What is the output of the following code?
\n\n\nTextField(
\n controller: TextEditingController(text: 'Hello'),
\n)\n
Displays an empty field
Displays 'Hello' in the text field
Throws an error
Displays a password input
Q85
Q85 How do you capture user input from a TextField in Flutter?
\n\n\nTextField(
\n onChanged: (text) {
\n // Capture input here\n },
\n)\n
Using the onSubmitted callback
Using the onChanged callback
Using a Validator
Using a GlobalKey
Q86
Q86 How would you create a form with multiple fields and a submit button in Flutter?
\n\n\nForm(
\n key: _formKey,
\n child: Column(
\n children:
\n }
\n },
\n child: Text('Submit'),
\n ),\n
],\n ),
\n)\n
Using FormField widget
Using Column widget
Using ListView widget
Using Row widget
Q87
Q87 What does the following code snippet demonstrate?
\n\n\nFormField(
\n builder: (FormFieldState
\n return Column(
\n children:
\n TextField(
\n onChanged: (text) {
\n state.didChange(text);
\n },\n ),
\n Text(
\n state.errorText ?? '',
\n style: TextStyle(color: Colors.red),
\n ),
\n ],
\n );
\n },
\n)\n
Basic form handling
Advanced form handling
Custom form field with validation
Form with multiple fields
Q88
Q88 What could be a reason for a TextField not updating its value correctly?
Incorrect onChanged callback
Missing controller
Not using a form key
Missing validation logic
Q89
Q89 How can you debug an issue where the form is not validating as expected?
Ignore the issue
Check the form key and validators
Rebuild the form
Remove the validators
Q90
Q90 Which package is commonly used for making HTTP requests in Flutter?
http
requests
axios
fetch