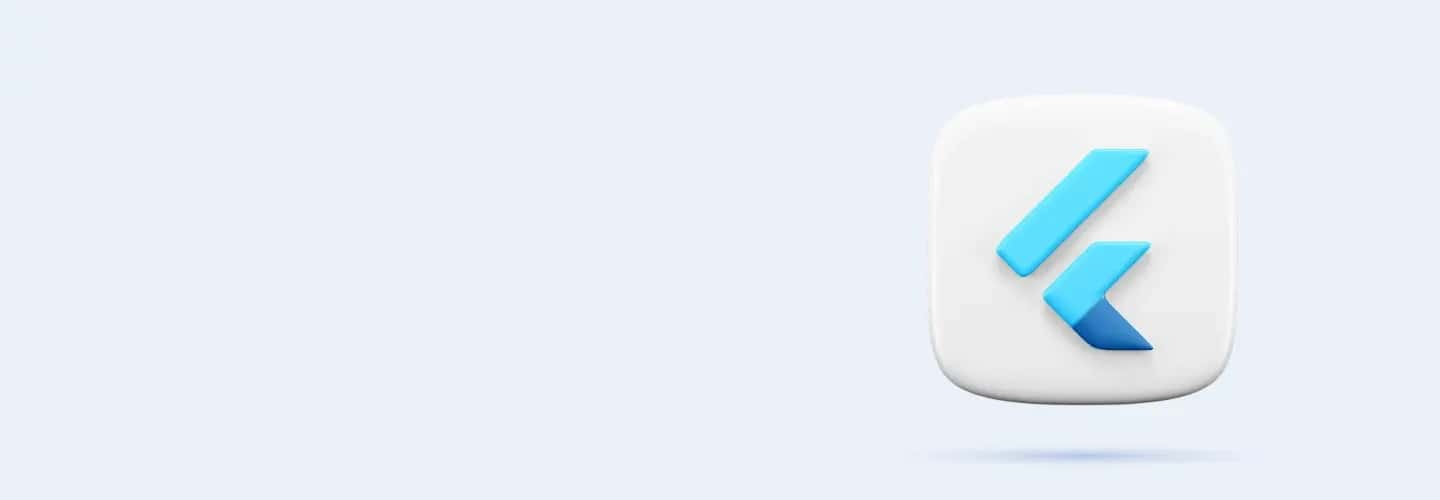
Q1
Q1 What is the primary advantage of using Flutter in mobile app development?
To enable server-side rendering of applications
To provide a back-end framework for mobile apps
To facilitate the rapid development of cross-platform mobile applications with a single codebase
To enhance the security features of mobile applications
Q2
Q2 Who maintains Flutter?
Microsoft
Apple
Q3
Q3 What language is used to write Flutter apps?
Java
Kotlin
Swift
Dart
Q4
Q4 Which of the following platforms is NOT supported by Flutter?
iOS
Android
Web
Nintendo Switch
Q5
Q5 How does Flutter achieve high performance in rendering?
Using WebView
Using a JavaScript engine
Using Skia for rendering
Using native device widgets
Q6
Q6 What is a common use of Flutter's hot reload feature?
To optimize app performance
To reduce the app size
To quickly view changes in the UI without restarting the app
To secure the app from vulnerabilities
Q7
Q7 Which command is used to create a new Flutter project?
flutter run
flutter create
flutter build
flutter start
Q8
Q8 Which widget in Flutter is used to create a visual hierarchy of the UI that controls the user interface layout?
MaterialApp
Widget
Scaffold
Column
Q9
Q9 Which IDE is commonly used for Flutter development?
Visual Studio
PyCharm
Eclipse
Android Studio
Q10
Q10 What command installs Flutter on macOS?
flutter install
brew install flutter
apt-get install flutter
install flutter
Q11
Q11 Which operating system does not support Flutter development?
Windows
macOS
Linux
MS-DOS
Q12
Q12 What is the purpose of the Flutter Doctor command?
To build the app
To run unit tests
To check the environment setup
To deploy the app
Q13
Q13 How do you update the Flutter SDK?
flutter upgrade
flutter update
flutter refresh
flutter reinstall
Q14
Q14 What is the first step in setting up Flutter on a new machine?
Installing the Flutter SDK
Creating a new project
Running flutter doctor
Configuring the IDE
Q15
Q15 What is the correct command to create a new Flutter project named my_app?
flutter new my_app
flutter init my_app
flutter create my_app
flutter start my_app
Q16
Q16 Which file must be configured to use Firebase in a Flutter project?
index.html
main.dart
pubspec.yaml
settings.gradle
Q17
Q17 What command initializes a Flutter app in an existing directory?
flutter create --init
flutter start --init
flutter init
flutter create .
Q18
Q18 What might cause a Flutter project to fail to build after setup?
Incorrect project name
Missing dependencies
Using a dark theme
Incorrect Flutter version
Q19
Q19 If running flutter doctor shows issues, what is a likely first step to resolve them?
Reinstall Flutter
Run flutter clean
Restart the IDE
Follow the recommendations provided by flutter doctor
Q20
Q20 Which of the following is a valid variable declaration in Dart?
var name = "John";
int number = 42;
String text = 'Hello';
All of the above
Q21
Q21 What is Dart primarily used for in Flutter?
Back-end development
Database management
User interface development
None of the above
Q22
Q22 Which keyword is used to define a constant value in Dart?
constant
static
final
const
Q23
Q23 What type does the main function return in a Dart application?
void
int
String
dynamic
Q24
Q24 Which of the following is NOT a built-in type in Dart?
int
float
String
bool
Q25
Q25 What does the async keyword indicate in Dart?
A function is synchronous
A function returns an integer
A function is asynchronous
A function returns a string
Q26
Q26 Which Dart collection is used to store key-value pairs?
List
Set
Map
Queue
Q27
Q27 What is the output of the following Dart code snippet?
\n\n\nvoid main() {
\n List
\n print(numbers[1]);
\n}\n
0
1
2
3
Q28
Q28 How do you declare a nullable variable of type int in Dart?
int? num;
int num?;
nullable int num;
int num = null;
Q29
Q29 What is the correct way to handle a potential null value using null-aware operators in Dart?
\n\n\nint? a = null;
\nint b = a ?? 0;
\nprint(b);\n
0
null
Throws an error
undefined
Q30
Q30 If a Dart program throws a NoSuchMethodError, what is a likely cause?
A method is missing a return type
A method is called on a null object
A method is missing parameters
A method is using the wrong return type