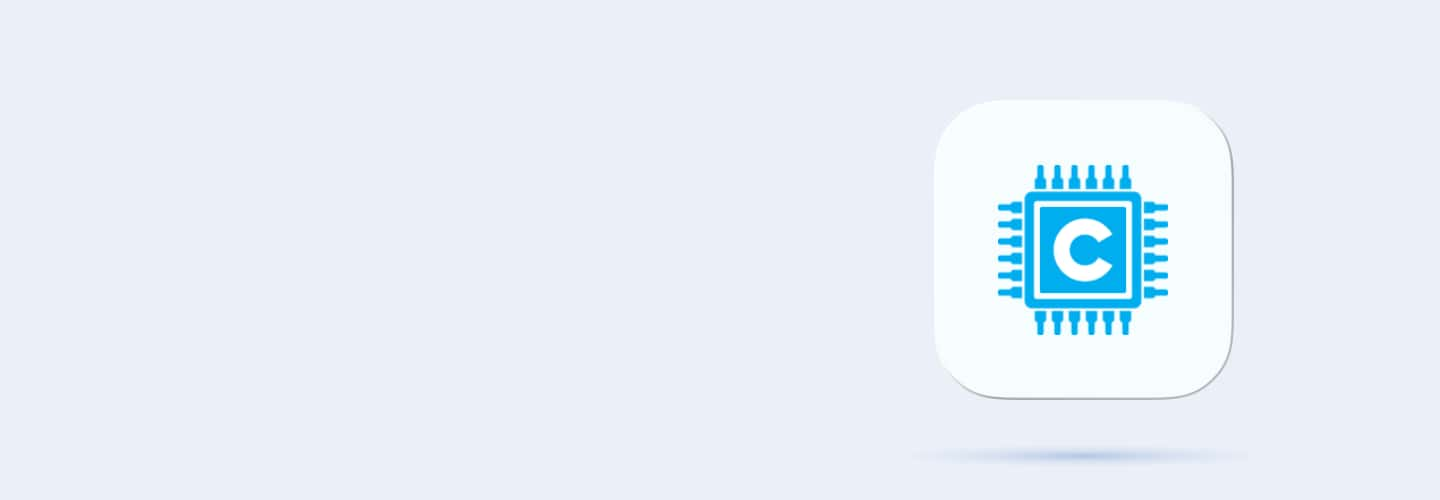
Q121
Q121 What does this code snippet do?
TWSR = (TWSR & 0xF8) | (1 << TWPS0);
Sets I2C clock speed
Sets I2C address
Configures I2C prescaler
Enables I2C interrupts
Q122
Q122 The I2C bus is not transmitting data. What could be a potential cause?
Incorrect clock frequency
Slave device address mismatch
Incorrect pull-up resistors
Data collision
Q123
Q123 What is the purpose of a breakpoint in debugging?
To stop execution at a specific line
To optimize the code
To execute a function
To generate an error
Q124
Q124 Which tool is commonly used to step through code during debugging?
Logic analyzer
JTAG debugger
Oscilloscope
Power supply
Q125
Q125 What is the role of the watchpoint in embedded debugging?
To monitor variable values during execution
To stop execution at a specific line
To measure program execution time
To monitor memory usage
Q126
Q126 Which of the following can be a consequence of using excessive logging in embedded systems?
Faster code execution
Memory leaks
Improper interrupt handling
Increased program size
Q127
Q127 What will this code snippet do?
while (1)
{
if (x == 5)
{
break;
}
}
Terminates the loop when x is 5
Runs indefinitely
Throws an error
Loops 5 times
Q128
Q128 What does this code snippet do?
int a = 10;
printf("Value: %d", a);
Prints the value of a as 10
Prints an error
Prints a memory address
Does nothing
Q129
Q129 A program does not display expected output. What could be the first step in debugging?
Check for syntax errors
Check for variable initialization
Examine code comments
Examine program output
Q130
Q130 A program crashes when accessing a specific memory address. What is the most likely cause?
Memory corruption
Buffer overflow
Incorrect pointer access
Invalid function call
Q131
Q131 What is the purpose of using loop unrolling in embedded systems?
To reduce memory usage
To increase execution time
To improve performance by reducing loop overhead
To simplify the code
Q132
Q132 What is the advantage of using DMA (Direct Memory Access) in embedded systems?
It increases processor load
It reduces memory usage
It reduces I/O operation overhead and improves data transfer efficiency
It simplifies code complexity
Q133
Q133 How does optimizing memory access patterns contribute to improved embedded system performance?
It reduces CPU clock speed
It decreases memory size
It minimizes wait times for memory access by aligning data in cache-friendly patterns
It increases the complexity of memory management
Q134
Q134 What does this code do?
#pragma optimize = off;
Enables compiler optimization
Disables compiler optimization
Provides a warning to the compiler
Sets memory alignment
Q135
Q135 What will happen when this optimization is applied?
#pragma GCC push_options
Saves previous compiler settings
Modifies compiler settings permanently
Disables optimization
Restores default settings
Q136
Q136 A program is running slower after enabling optimizations. What could be the reason?
Optimizations disabled
Incorrect memory access pattern
Over-optimization of code
Under-optimization of code
Q137
Q137 A variable declared as static in embedded C is not updated as expected. What could be the issue?
Variable shadowing
Improper use of pointers
Incorrect memory address access
Compiler optimization on static variables
Q138
Q138 What is the primary purpose of using low-power modes in embedded systems?
To save data
To reduce power consumption
To increase processor speed
To enhance memory performance
Q139
Q139 What is the main benefit of using sleep modes in microcontrollers?
Reduces memory usage
Increases processing power
Minimizes power consumption while maintaining state
Reduces I/O operation time
Q140
Q140 Why is clock gating used in embedded systems for power management?
To stop clock signals to certain peripherals
To increase clock speed
To reduce power to the processor
To reduce memory usage
Q141
Q141 What does this code do?
PRR |= (1 << PRUSART0);
Disables USART0 module
Enables USART0 module
Resets USART0 module
Configures USART0 baud rate
Q142
Q142 What will happen in this code snippet?
if (SLEEP_MODE_IDLE)
{
sleep_mode();
}
Puts the system into idle sleep mode
Puts the system into active mode
Exits the sleep mode
Waits for an interrupt
Q143
Q143 A microcontroller is not entering its low-power sleep mode as expected. What could be the issue?
The sleep mode is not enabled
An interrupt is preventing sleep mode
The clock speed is too high
The memory is not optimized
Q144
Q144 The microcontroller is consuming more power than expected, even in idle mode. What is a likely cause?
Incorrect power management settings
Too many active peripherals
Incorrect sleep mode configuration
High clock frequency
Q145
Q145 In an embedded application for a smart thermostat, which sensor is most likely to be used for temperature measurement?
Accelerometer
Thermistor
Gyroscope
Proximity sensor
Q146
Q146 In a wearable health monitor, which power management technique would be most beneficial for extending battery life?
Constant voltage regulator
Sleep modes
Dynamic voltage scaling
Clock gating
Q147
Q147 In a traffic monitoring system, what does the following code do?
if (sensorData > threshold)
{
alert();
}
Sends an alert if traffic exceeds threshold
Records sensor data
Stores traffic data
Activates traffic signal
Q148
Q148 What will be the effect of this code in a motor control system?
if (sensorInput == HIGH)
{
motorStart();
}
Starts the motor when sensor input is high
Stops the motor when sensor input is high
Starts the motor when sensor input is low
No effect
Q149
Q149 A sensor in an embedded system is providing erratic readings. What could be a common cause?
Incorrect calibration
Faulty wiring
Signal interference
Software bug
Q150
Q150 An embedded system is failing to start after a firmware update. What could be the issue?
Incorrect bootloader configuration
Low battery voltage
Faulty sensor initialization
Excessive interrupt handling