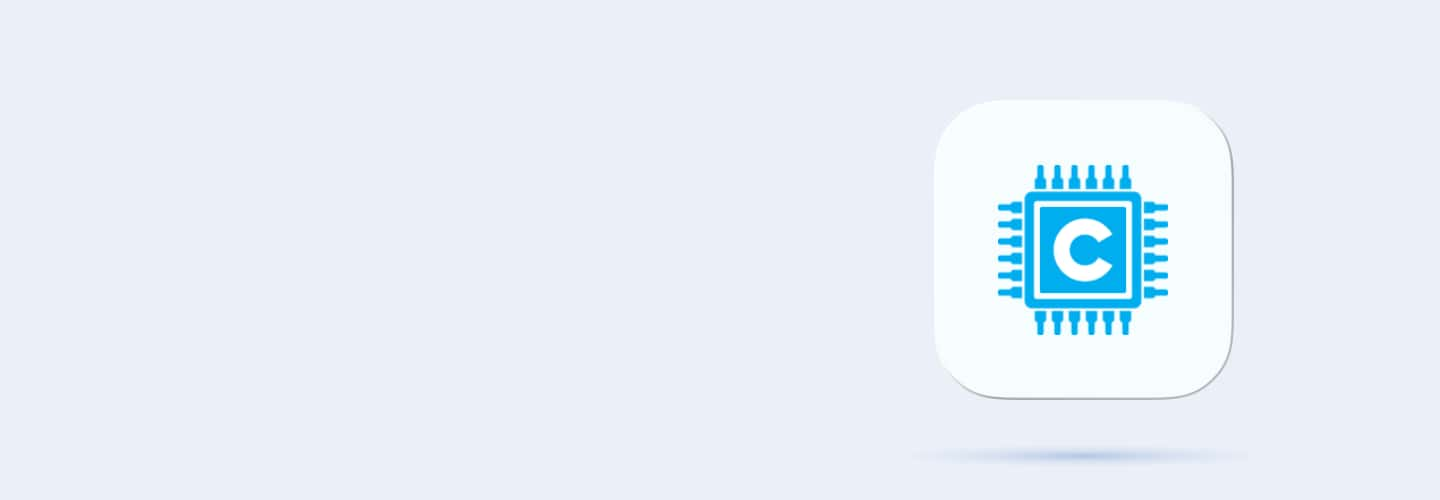
Q61
Q61 What will the following code do?
void attribute((interrupt)) ISR(void) { /* code */ }
Generates a warning
Executes as ISR
Throws an error
Creates a function
Q62
Q62 Which instruction enables global interrupts in a typical microcontroller?
SETI
EI
CLI
RESET
Q63
Q63 What will happen with this code?
void ISR() { static int count = 0; count++; }
Count resets to 0
Count increments
Error
Infinite loop
Q64
Q64 How does this ISR behave?
void ISR(void) { if (flag)
{
return;
} flag = 1; }
Executes once
Executes only if flag is 0
Infinite loop
Error
Q65
Q65 An interrupt fails to trigger the ISR. What is the most likely cause?
ISR not defined
Global interrupts disabled
Wrong interrupt vector
ISR is improperly linked
Q66
Q66 A high-priority interrupt delays the execution of a low-priority interrupt. What is this behavior called?
Interrupt nesting
Interrupt masking
Interrupt priority
Interrupt queueing
Q67
Q67 A system hangs after an interrupt. What could be the issue?
Infinite loop in ISR
Stack overflow
Incorrect return address
Uncleared interrupt flag
Q68
Q68 Which type of memory is used to store variables that are initialized during runtime?
ROM
RAM
EEPROM
Flash Memory
Q69
Q69 What is the primary purpose of the stack in memory management?
To store instructions
To store global variables
To store function call data
To store static variables
Q70
Q70 What happens when a memory leak occurs in an embedded system?
Memory is automatically freed
Memory becomes unavailable
Memory remains unused
Memory is recycled
Q71
Q71 Which memory region is used for dynamically allocated memory in embedded systems?
Stack
Heap
Code Segment
Data Segment
Q72
Q72 What is the purpose of memory-mapped I/O in embedded systems?
To execute code
To access peripherals
To allocate memory
To store global variables
Q73
Q73 What will this code do?
int *ptr = malloc(sizeof(int));
if(ptr == NULL)
printf("Error");
Allocates memory
Prints "Error"
Does nothing
Throws an error
Q74
Q74 What will happen if free() is called on a NULL pointer?
Segmentation fault
Program crashes
Nothing happens
Memory is corrupted
Q75
Q75 What will be the output of the code?
int *p = (int *)malloc(4);
*p = 10;
free(p);
printf("%d", *p);
10
Garbage value
0
Segmentation fault
Q76
Q76 How does this code behave?
int arr[5];
printf("%d", arr[10]);
Prints 0
Segmentation fault
Garbage value
Error
Q77
Q77 A dynamically allocated memory block is accessed after being freed. What could be the consequence?
Memory corruption
Segmentation fault
Undefined behavior
Reduced performance
Q78
Q78 A stack overflow occurs in an embedded system. What is the most likely reason?
Too many function calls
Global variable overflow
Incorrect malloc usage
Heap corruption
Q79
Q79 A program exhibits random crashes. Debugging reveals issues with dangling pointers. What causes this?
Uninitialized variables
Memory freed incorrectly
Incorrect pointer arithmetic
Heap overflow
Q80
Q80 What is the primary purpose of a timer in embedded systems?
Generate delays
Store data
Handle interrupts
Enable memory management
Q81
Q81 Which of the following registers typically stores the current value of a timer?
Control register
Data register
Timer register
Interrupt flag register
Q82
Q82 What is the role of a prescaler in timer programming?
Increase timer frequency
Reduce timer resolution
Divide clock frequency
Store timer values
Q83
Q83 What is the difference between a timer and a counter in embedded systems?
Timer uses external events
Counter uses internal clock
Timer uses internal clock
No difference
Q84
Q84 Which mode in a timer is used to generate a Pulse Width Modulation (PWM) signal?
Overflow mode
Capture mode
Compare mode
Normal mode
Q85
Q85 What will the following code do?
TCCR0 |= (1 << CS01);
Starts timer with prescaler
Stops the timer
Resets the timer
Enables timer interrupt
Q86
Q86 What will happen in this code?
if (TIFR & (1 << TOV0)) { TIFR |= (1 << TOV0); }
Stops timer overflow
Resets timer overflow flag
Starts timer overflow
Configures timer overflow
Q87
Q87 What does this configuration do?
TCCR1A = 0x00; TCCR1B = (1 << CS10);
Enables timer with no prescaler
Configures PWM
Stops timer
Enables interrupt
Q88
Q88 What will happen in this code?
OCR0 = 100;
while (1) {
if (TCNT0 == OCR0) PORTB ^= (1 << PB0);
}
Toggles PB0 at 100 counts
Toggles PB0 at 255 counts
No output
Infinite loop
Q89
Q89 A timer fails to start in your program. What is the likely reason?
Incorrect prescaler
Interrupt not enabled
Timer overflow
Wrong initialization
Q90
Q90 A timer generates incorrect delays. What could be the possible cause?
Incorrect clock frequency
Incorrect timer mode
Wrong prescaler
All of these