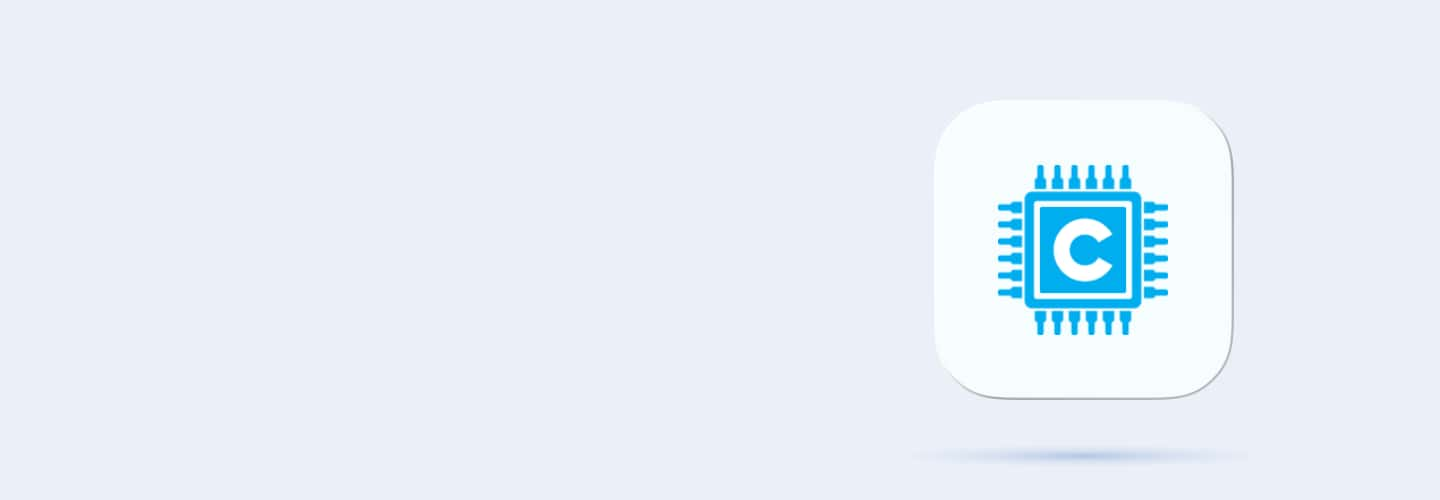
Q31
Q31 A global variable declared with the static keyword is not accessible in another file. Why?
Compiler error
Limited scope
Variable shadowing
Incorrect linkage
Q32
Q32 What is the result of the bitwise AND operation 5 & 3?
1
2
3
7
Q33
Q33 Which of the following operations sets a particular bit in a number to 1?
OR with a mask
AND with a mask
XOR with a mask
NOT with a mask
Q34
Q34 How do you check if the nth bit of a number is set?
AND with a mask
OR with a mask
XOR with a mask
NOT operation
Q35
Q35 What does the left shift operator (<<) do in Embedded C?
Divides the number
Multiplies the number
Shifts bits to the right
Clears the bits
Q36
Q36 Which bitwise operation is used to toggle (flip) a specific bit in a number?
AND
OR
XOR
NOT
Q37
Q37 What happens if a signed integer undergoes a left shift and the most significant bit (MSB) becomes 1?
Logical error
Overflow
MSB is ignored
Program crash
Q38
Q38 What will be the output of this code?
int x = 5;
printf("%d", x << 1);
5
10
0
15
Q39
Q39 Given the code
int x = 6; x = x & (~(1 << 1));
printf("%d", x);,
what will the output be?
2
4
6
0
Q40
Q40 What will the output of the code be?
int x = 9; x = x ^ (1 << 3);
printf("%d", x);
1
0
9
1
Q41
Q41 A variable behaves unexpectedly during bitwise operations. What could be a possible cause?
Incorrect mask
Memory leak
Pointer error
Clock mismatch
Q42
Q42 A program calculates incorrect values during a left shift operation. What could be the issue?
Signed overflow
Logical error
Clock skew
Incorrect data type
Q43
Q43 A bitwise AND operation between two variables gives unexpected results. What is the most likely issue?
Uninitialized variable
Memory overflow
Interrupt error
Incorrect loop
Q44
Q44 What does a pointer store in C?
Data value
Address of another variable
Data type
Size of variable
Q45
Q45 Which operator is used to dereference a pointer?
&
*
->
[]
Q46
Q46 What is a NULL pointer?
Pointer pointing to zero address
Pointer pointing to garbage
Uninitialized pointer
Constant pointer
Q47
Q47 Can a pointer to a variable be assigned to a pointer of a different data type?
Yes, with casting
No
Yes, directly
Only for integers
Q48
Q48 Which of the following is a valid declaration of a pointer to a function in C?
int *func()
int (*func)()
int &func()
int (*func[])()
Q49
Q49 What is the purpose of a double pointer in C?
To point to an array
To store a pointer to another pointer
To allocate memory
To access hardware registers
Q50
Q50 What will the output of the following code be?
int x = 10;
int *p = &x;
printf("%d", *p);
0
10
Address of x
Error
Q51
Q51 What will the output of this code be?
int arr[] = {1, 2, 3};
int *p = arr;
printf("%d", *(p+1));
1
2
3
Error
Q52
Q52 Given
int **pp; int a = 5;
int *p = &a; pp = &p;,
what does **pp represent?
Address of a
Value of a
Value of p
Error
Q53
Q53 A pointer is dereferenced, but the program crashes. What could be the most likely cause?
NULL pointer
Uninitialized variable
Memory overflow
Infinite loop
Q54
Q54 A pointer to dynamically allocated memory is reassigned without freeing it. What could happen?
Memory leak
Stack overflow
Segmentation fault
Incorrect pointer arithmetic
Q55
Q55 A pointer arithmetic operation produces incorrect results. What is the most likely issue?
Misaligned pointer
Incorrect data type
Pointer overflow
All of the above
Q56
Q56 What is an interrupt in Embedded C?
A delay in the program
A function call
A signal to the processor
A system error
Q57
Q57 Which type of interrupt can be ignored by the processor?
Maskable
Non-maskable
Software
Hardware
Q58
Q58 What is the purpose of the Interrupt Service Routine (ISR)?
To handle an interrupt
To execute the main function
To save stack pointers
To delay execution
Q59
Q59 What is typically saved by the processor when an interrupt occurs?
Only program counter
Stack pointer
Program counter and CPU state
Interrupt vector
Q60
Q60 What is the vector table used for in interrupt handling?
To store variables
To hold function addresses
To define memory
To list interrupt addresses