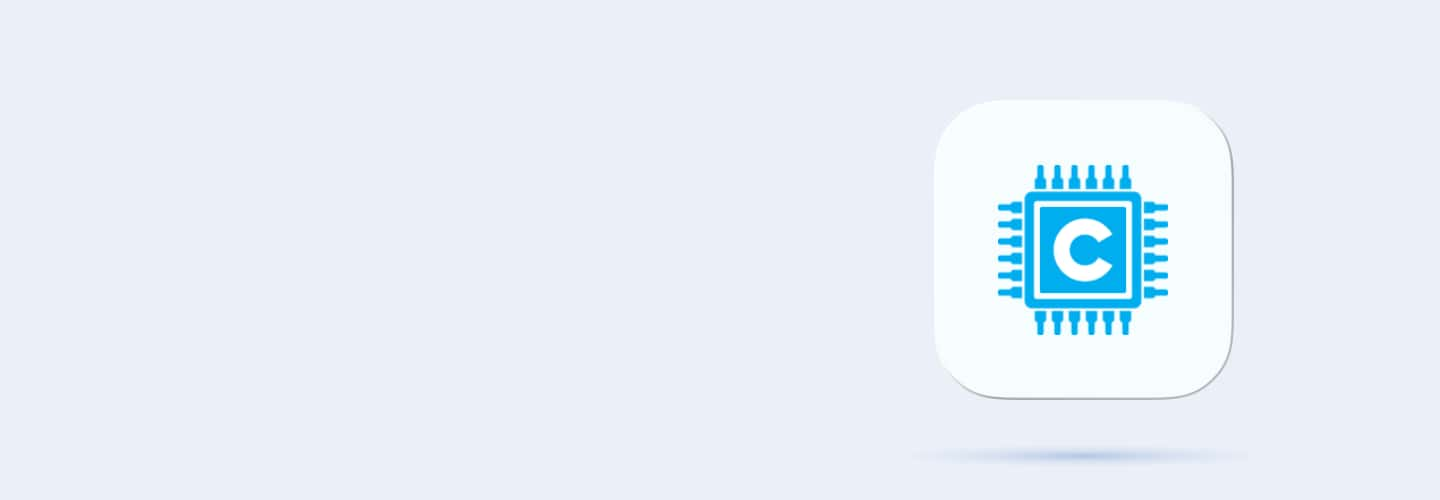
Q1
Q1 What is the primary purpose of using Embedded C?
To create operating systems
To program microcontrollers and develop firmware
To design graphical user interfaces
To create mobile applications
Q2
Q2 Which of the following is a feature of Embedded C?
Object-oriented programming
Real-time capability
Automatic memory management
Dynamic typing
Q3
Q3 Embedded C programs are typically designed for:
Real-time systems
General-purpose applications
Web development
Database management
Q4
Q4 Which of the following is NOT typically a use case for Embedded C?
Programming microcontrollers
Developing firmware
Building real-time operating systems
Designing video games
Q5
Q5 Which of the following statements about Embedded C is true?
It is a hardware-independent language
It is a hardware-specific extension of C
It does not support pointers
It is only used for microprocessors
Q6
Q6 What differentiates Embedded C from standard C?
Usage of dynamic memory allocation
Use of hardware-specific registers
Extensive use of file handling
Focus on graphical interfaces
Q7
Q7 In Embedded C, why is direct hardware manipulation important?
To simplify programming
To improve debugging
To achieve low-level control
To ensure portability
Q8
Q8 Which directive is used to include standard libraries in Embedded C?
#header
#include
#import
#define
Q9
Q9 An Embedded C program does not produce the expected output after interfacing with an external device. What is the most likely issue?
Incorrect clock frequency
Improper use of #define
Missing semicolon
Logical error
Q10
Q10 What is the file extension typically used for Embedded C programs?
.c
.cpp
.ec
.exe
Q11
Q11 Which function is mandatory in every Embedded C program?
main()
start()
init()
execute()
Q12
Q12 What is the typical range of values for an 8-bit unsigned integer in Embedded C?
0 to 255
-128 to 127
0 to 511
-255 to 255
Q13
Q13 In Embedded C, which keyword is used to define a constant value?
static
const
define
volatile
Q14
Q14 What is the main purpose of the volatile keyword in Embedded C?
To optimize the variable
To ensure portability
To prevent compiler optimization
To declare a constant
Q15
Q15 What happens if an Embedded C program tries to divide by zero?
Compiler error
Runtime error
Undefined behavior
Logical error
Q16
Q16 Which section of memory is used to store global and static variables in Embedded C?
Stack
Heap
Data segment
Registers
Q17
Q17 Which type of variables are automatically initialized to zero in Embedded C?
Local
Static
Register
Automatic
Q18
Q18 What will be the output of the following code?
int a = 5, b = 3;
printf("%d", a % b);
2
1
0
5
Q19
Q19 A program runs correctly but produces incorrect output. The issue is identified as a variable that gets modified unexpectedly. What could be the cause?
Use of volatile variable
Stack overflow
Variable shadowing
Watchdog timer not reset
Q20
Q20 What is the size of an int data type on a typical 16-bit microcontroller?
2 bytes
4 bytes
1 byte
8 bytes
Q21
Q21 Which data type is best suited for storing a character in Embedded C?
char
int
float
unsigned int
Q22
Q22 What is the range of an 8-bit signed integer in Embedded C?
0 to 255
-128 to 127
-255 to 255
0 to 127
Q23
Q23 Which of the following is NOT a valid storage class in Embedded C?
auto
register
static
heap
Q24
Q24 What does the extern keyword indicate about a variable in Embedded C?
Local scope
Global declaration
Static allocation
Dynamic initialization
Q25
Q25 What is the default storage class for local variables in Embedded C?
auto
static
register
extern
Q26
Q26 What is the difference between static and register storage classes in Embedded C?
Both are stored in RAM
Static is stored in RAM, register in CPU
Both are global
Static is faster than register
Q27
Q27 What will be the output of the following code?
static int count = 0;
count++;
printf("%d", count);
0
1
Garbage value
Error
Q28
Q28 What will be the output of the following code?
int a = 10; { int a = 20;
printf("%d", a); }
10
20
Error
Garbage value
Q29
Q29 A variable in your program changes its value unexpectedly. What is the most probable cause in the context of storage classes?
Incorrect initialization
Static declaration
Volatile not used
Heap corruption
Q30
Q30 A local variable retains its value even after exiting a function. Which storage class might have been used?
auto
static
register
extern