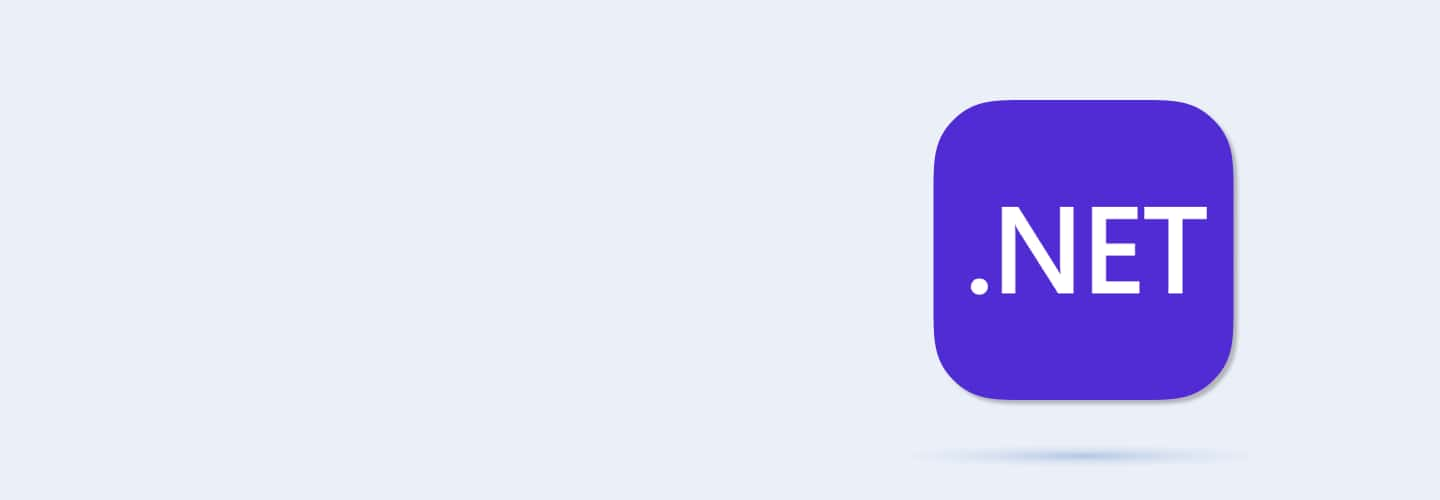
Q121
Q121 Which operator is used in LINQ to join two collections based on a related key?
Join
Union
Concat
Intersect
Q122
Q122 Which LINQ query syntax in C# retrieves even numbers from a list numbers?
from n in numbers where n % 2 == 0 select n;
select n where n % 2 == 0 from numbers;
where n in numbers select n == 0;
select numbers where n % 2 == 0;
Q123
Q123 Which code snippet demonstrates using LINQ’s OrderBy method in C# to sort a list of strings?
list.Sort();
list.OrderBy();
list.OrderBy(x => x);
Order(list);
Q124
Q124 How would you perform a group by operation using LINQ to group students by age?
students.GroupBy(s => s.Age);
students.Select(s => s.Age);
students.SortBy(s => s.Age);
students.ByGroup(s => s.Age);
Q125
Q125 A "NullReferenceException" occurs during a LINQ query. What could be a likely cause?
LINQ syntax error
Null variable in query
Invalid data type
Database connection error
Q126
Q126 A developer notices slow performance when executing a large LINQ query. What could be a potential solution?
Avoid OrderBy
Optimize query structure
Add more conditions
Use foreach loop
Q127
Q127 What is Entity Framework primarily used for in .NET?
User interface design
Database management
Data access and ORM
Network communication
Q128
Q128 Which approach in Entity Framework generates a database schema based on your code?
Database-First
Code-First
Model-First
Query-First
Q129
Q129 What is the purpose of DbContext in Entity Framework?
Manages database schema
Executes SQL commands
Maintains database connection and manages data operations
Handles UI
Q130
Q130 Which Entity Framework feature allows developers to track changes in object states?
Data Binding
Change Tracking
Lazy Loading
Query Compilation
Q131
Q131 Which method is used to save changes made to the database in Entity Framework?
Update()
Execute()
SaveChanges()
ApplyChanges()
Q132
Q132 How would you configure a one-to-many relationship in Entity Framework using the Fluent API?
HasOne().WithOne();
HasOne().WithMany();
HasMany().WithOne();
HasMany().WithMany();
Q133
Q133 Which method in Entity Framework is used to execute raw SQL queries and return entity objects?
ExecuteRaw()
ExecuteQuery()
FromSqlRaw()
SqlQuery()
Q134
Q134 A "DbUpdateException" occurs when updating data in Entity Framework. What is a likely cause?
Foreign key constraint violation
Database offline
Connection string error
Primary key duplication
Q135
Q135 A developer encounters a "LazyInitializationException" in Entity Framework. What could be a solution?
Use SaveChanges()
Use Include() to load related data
Ignore the exception
Rewrite query structure
Q136
Q136 What is the purpose of the SecureString class in .NET?
To store encrypted strings
To securely store sensitive text data in memory
To hash passwords
To manage secure tokens
Q137
Q137 Which .NET class provides methods for hashing data using algorithms like SHA256?
Encryptor
HashAlgorithm
CryptoStream
DataProtector
Q138
Q138 What is the purpose of role-based security in .NET applications?
Control access based on user roles
Encrypt sensitive data
Handle session management
Create user profiles
Q139
Q139 Which .NET class is primarily used for asymmetric encryption?
Aes
Rsa
TripleDES
Sha256
Q140
Q140 Which code snippet demonstrates encrypting data using Aes encryption in .NET?
new Aes().Encrypt(data);
Aes.Create().CreateEncryptor();
Aes.Encrypt(data);
Encrypt(data, Aes);
Q141
Q141 Which method in the CryptoStream class is used to write encrypted data to a stream in .NET?
WriteEncrypted()
Write()
EncryptData()
WriteStream()
Q142
Q142 A developer encounters a "CryptographicException" when decrypting data. What could be a likely cause?
Incorrect password
Key mismatch
File is locked
Data is too large
Q143
Q143 A "SecureString" cannot be converted directly to a string in .NET. What is the most secure way to handle it?
Copy contents to string
Store as plain text
Use Marshal class to convert securely
Read SecureString directly
Q144
Q144 What is the main advantage of .NET Core over the .NET Framework?
Better security
Cross-platform support
Improved UI
Easier syntax
Q145
Q145 Which command is used to create a new .NET Core project?
dotnet make
dotnet new
dotnet create
dotnet init
Q146
Q146 What is the purpose of the dotnet publish command in .NET Core?
To build the application
To start the application
To create a self-contained deployable package
To remove dependencies
Q147
Q147 Which command builds a .NET Core application for production?
dotnet release
dotnet build -c Release
dotnet production
dotnet build -r
Q148
Q148 How would you run a .NET Core application from the command line?
run dotnet
dotnet start
dotnet run
dotnet execute
Q149
Q149 A "Missing runtime" error occurs when deploying a .NET Core application. What is a likely solution?
Reinstall the OS
Deploy with dotnet publish
Increase memory
Use .NET Framework
Q150
Q150 A developer needs to resolve dependency conflicts in a .NET Core project. What tool can assist with this?
NuGet
MSBuild
dotnet clean
Dependency Injection