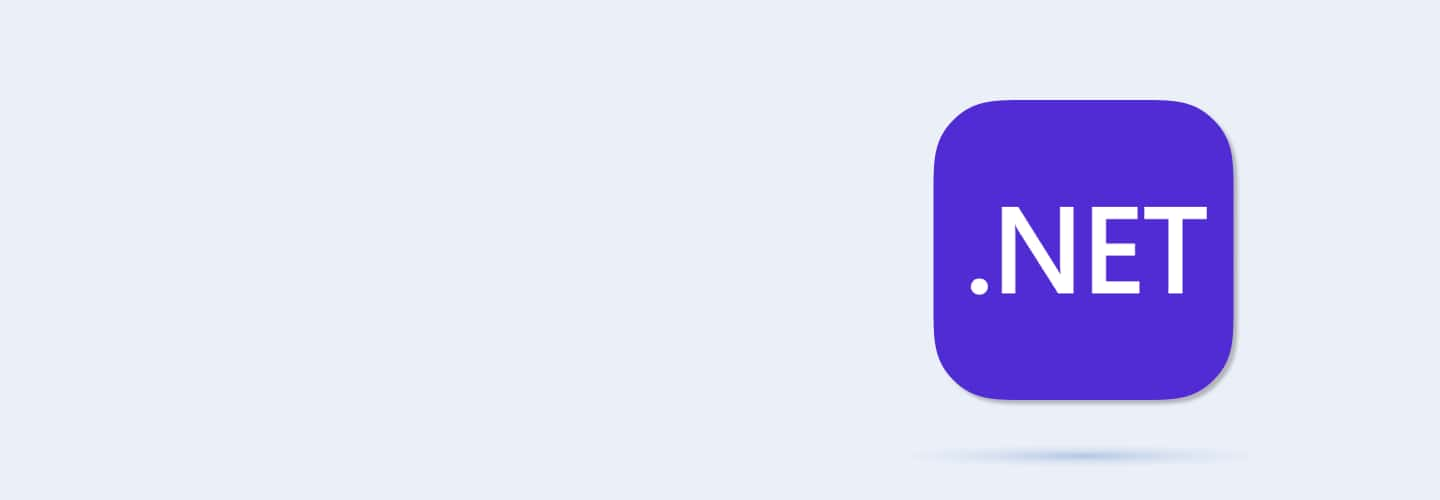
Q91
Q91 Which class would you use to write data to a binary file in .NET?
BinaryReader
BinaryWriter
FileWriter
StreamWriter
Q92
Q92 What does the FileMode.Create option do when opening a file in .NET?
Opens file if it exists
Throws exception if file exists
Creates new file or overwrites existing
Creates file only if it doesn’t exist
Q93
Q93 How would you open a file for reading in C#?
File.OpenWrite("file.txt")
File.OpenRead("file.txt")
File.ReadAll("file.txt")
File.Open("file.txt")
Q94
Q94 Which code snippet correctly appends text to a file in C#?
File.Write("file.txt", "text");
File.WriteAll("file.txt", "text");
File.AppendAllText("file.txt", "text");
File.InsertText("file.txt", "text");
Q95
Q95 How would you delete a file named "oldFile.txt" in C#?
File.Remove("oldFile.txt");
File.Delete("oldFile.txt");
File.Erase("oldFile.txt");
File.Unlink("oldFile.txt");
Q96
Q96 A "FileNotFoundException" occurs. What is the likely reason?
Incorrect permissions
File already open
File does not exist
File is too large
Q97
Q97 A "UnauthorizedAccessException" occurs while accessing a file. What could be a solution?
Check if file is read-only
Recompile the program
Restart the system
Delete the file
Q98
Q98 A developer is unable to read a large file due to memory limitations. What could be a solution?
Increase CPU power
Use File.ReadAllText
Use StreamReader with buffering
Close other applications
Q99
Q99 Which language is primarily used for server-side coding in ASP.NET?
C++
Java
C#
HTML
Q100
Q100 Which directive is used to include a namespace in an ASP.NET page?
#import
#include
@using
@namespace
Q101
Q101 What is the main purpose of ASP.NET Web Forms?
To design mobile applications
To create Windows applications
To develop web applications with a visual interface
To handle database connections
Q102
Q102 What is ViewState in ASP.NET?
Stores client-side data
Stores session data
Maintains state of controls
Stores cookies
Q103
Q103 Which control in ASP.NET allows data binding with multiple data sources, like SQL databases and XML files?
GridView
ListView
FormView
DetailsView
Q104
Q104 How would you create a basic HTML form in an ASP.NET page?
<asp:form>
<form>
<Form>
<asp:htmlform>
Q105
Q105 Which code snippet demonstrates how to retrieve a TextBox value in ASP.NET?
TextBox1.Value
TextBox1.Text
TextBox1.Input
TextBox1.Data
Q106
Q106 How would you bind a GridView control to a data source programmatically in ASP.NET?
GridView.Bind(Data);
GridView.DataBind();
GridView.DataSource = Data; GridView.DataBind();
Bind(GridView, Data);
Q107
Q107 A "NullReferenceException" occurs when accessing a control in ASP.NET. What could be a likely cause?
Page not loaded
Control is null
Invalid variable
Form is closed
Q108
Q108 A developer is unable to retain user input after a postback in ASP.NET Web Forms. What might be a solution?
Enable ViewState
Disable ViewState
Clear Session
Restart application
Q109
Q109 What is ADO.NET primarily used for in .NET?
User interface design
Network communication
Database access
Error handling
Q110
Q110 Which object in ADO.NET is used to establish a connection to a database?
DataReader
DataAdapter
Connection
Command
Q111
Q111 What is the purpose of the DataSet in ADO.NET?
Fetch a single row
Manage database connections
Store data locally
Execute SQL commands
Q112
Q112 Which of the following is a key advantage of using DataReader over DataSet in ADO.NET?
Stores data in memory
Supports disconnected data
Improved performance for read-only data
Easier to update data
Q113
Q113 Which code snippet demonstrates opening a database connection in ADO.NET?
connection.Open();
connection.Start();
connection.Run();
connection.Connect();
Q114
Q114 Which ADO.NET class is used to execute a SQL query that returns a single value, such as a count of rows?
ExecuteNonQuery
ExecuteScalar
ExecuteReader
ExecuteQuery
Q115
Q115 How would you retrieve data from a DataReader in a while loop in C#?
while (reader.Read()) { ... }
for (reader.Next()) { ... }
if (reader.Fetch()) { ... }
loop (reader.Read()) { ... }
Q116
Q116 A "SqlException" is thrown while connecting to a SQL database. What is a likely cause?
Incorrect permissions
Database is read-only
Incorrect connection string
Memory overflow
Q117
Q117 A "DataAdapter.Fill" method fails to retrieve data. What could be a potential cause?
DataReader closed
DataAdapter not initialized
Connection not open
SQL query missing
Q118
Q118 What does LINQ stand for?
Language Integrated Query
Logical Integrated Query
Library Integrated Query
List Integrated Query
Q119
Q119 What is the main advantage of using LINQ in .NET?
Improves runtime performance
Eliminates need for SQL
Type-safe querying
Replaces all SQL databases
Q120
Q120 Which LINQ method is used to filter a sequence based on a predicate?
Select
Where
OrderBy
Join