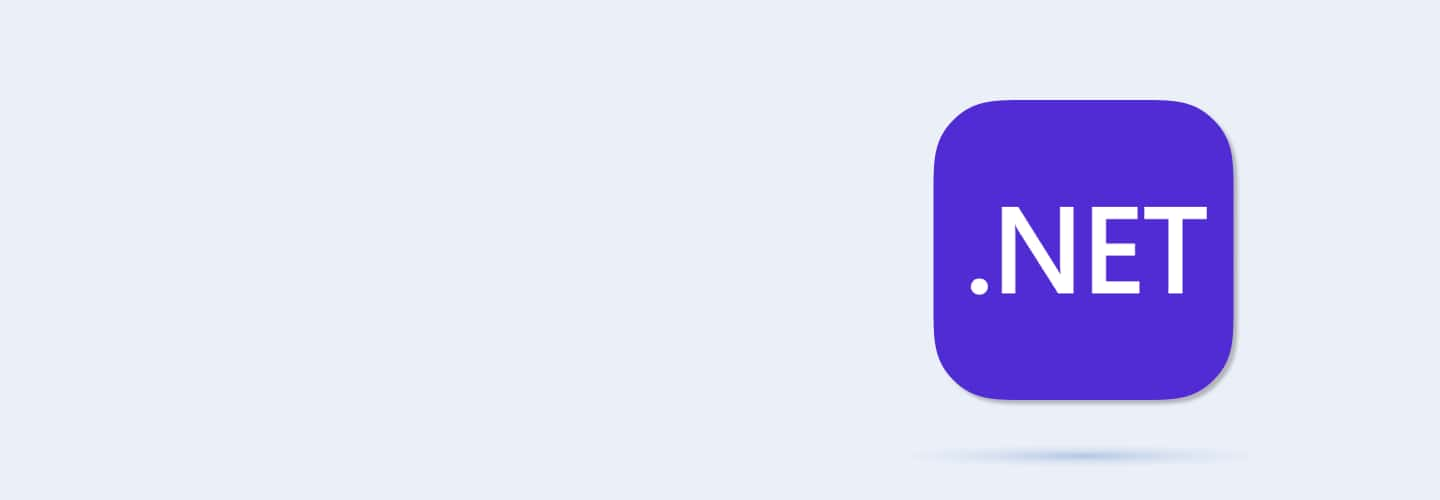
Q61
Q61 Which exception is caught by the catch (Exception ex) statement?
Only Exception
All exceptions derived from Exception
System-level errors
Only runtime errors
Q62
Q62 How do you rethrow an exception in C# without resetting the stack trace?
throw;
throw ex;
throw new Exception(ex);
rethrow ex;
Q63
Q63 A "NullReferenceException" occurs. What is the most likely reason?
Object is null
File not found
Syntax error
Array index out of bounds
Q64
Q64 An application frequently throws an OutOfMemoryException. What could be a solution?
Increase system memory
Optimize memory usage
Use more variables
Reduce CPU usage
Q65
Q65 A developer wants to log details of an exception without disrupting execution. What is a possible approach?
Use throw;
Log in catch block and continue
Avoid catch block
Use finally block
Q66
Q66 Which concept of OOP allows the creation of objects from a class?
Inheritance
Encapsulation
Polymorphism
Instantiation
Q67
Q67 What is encapsulation in OOP?
Combining methods and properties
Hiding data
Allowing multiple forms
Creating objects
Q68
Q68 Which OOP feature allows a derived class to inherit properties from a base class?
Abstraction
Polymorphism
Inheritance
Encapsulation
Q69
Q69 Which keyword is used in C# to prevent a class from being inherited?
sealed
static
readonly
protected
Q70
Q70 What is the purpose of polymorphism in OOP?
To hide data
To enhance readability
To enable objects to take many forms
To provide inheritance
Q71
Q71 How would you declare a simple class named Person in C#?
class Person { }
Person class { }
object Person { }
struct Person { }
Q72
Q72 Which C# keyword is used to call a base class constructor from a derived class?
this
super
base
inherit
Q73
Q73 Which code snippet correctly demonstrates method overriding in C#?
override void Print()
override method Print()
override class Print()
new method Print()
Q74
Q74 How would you declare an abstract method named Calculate in an abstract class?
abstract void Calculate();
abstract Calculate();
void abstract Calculate();
Calculate abstract void();
Q75
Q75 A developer encounters an error saying "Object reference not set to an instance of an object." What is likely the cause?
The object is null
The object has been disposed
Data type mismatch
Method does not exist
Q76
Q76 A method is not accessible outside its class, even when the instance is created. What could be the reason?
The method is public
The method is private
The method is static
The method is sealed
Q77
Q77 A derived class cannot inherit members of a base class. What might be the cause?
Members are sealed
Members are internal
Members are protected
Members are abstract
Q78
Q78 Which of the following is a generic collection in .NET?
Array
List
Hashtable
ArrayList
Q79
Q79 What is the primary benefit of using generics in .NET?
Reduces memory usage
Improves code readability
Increases type safety
Improves debugging
Q80
Q80 Which namespace provides collection classes like List
System
System.Generic
System.Collections.Generic
System.Data
Q81
Q81 Which interface is implemented by collections that can be iterated in a foreach loop?
IEnumerable
IEnumerator
IList
ICollection
Q82
Q82 What is the difference between Dictionary<K, V> and Hashtable in .NET?
Both are type-safe
Hashtable is generic
Dictionary
Hashtable is faster
Q83
Q83 How would you declare a generic List of integers in C#?
List numbers = new List
List
List numbers
List
Q84
Q84 Which code snippet demonstrates adding a key-value pair to a Dictionary<string, int> in C#?
dict.Add("one", 1);
dict.Insert("one", 1);
dict["one"] = 1;
dict.put("one", 1);
Q85
Q85 How do you remove an item with a specific key from a Dictionary<int, string> in C#?
dict.Delete(1);
dict.Remove(1);
dict.Clear(1);
dict.Erase(1);
Q86
Q86 A KeyNotFoundException occurs when accessing a dictionary. What is likely the cause?
The key is missing
The value is null
The dictionary is empty
The key is duplicated
Q87
Q87 An InvalidOperationException occurs when modifying a collection during iteration. What is a possible solution?
Use a for loop
Use a foreach loop
Use a sorted collection
Clear the collection first
Q88
Q88 A developer wants to prevent duplicate keys in a collection. Which collection should they use?
List
Hashtable
Dictionary
SortedSet
Q89
Q89 Which namespace provides classes for file handling in .NET?
System.Data
System.IO
System.File
System.Text
Q90
Q90 Which class in .NET is used to read text from a file?
TextReader
StreamReader
FileReader
TextWriter