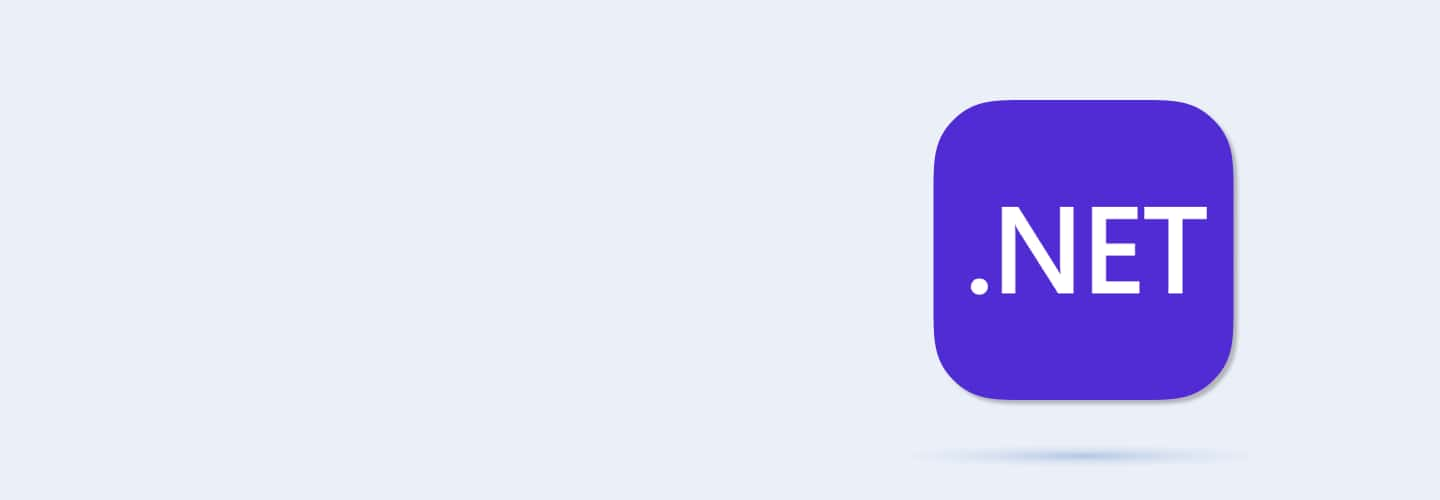
Q31
Q31 An application runs slowly due to frequent garbage collection. What could help improve performance?
Remove all variables
Optimize memory usage
Use fewer libraries
Disable garbage collection
Q32
Q32 What is the purpose of the .NET Framework Class Library (FCL)?
To manage memory
To provide a collection of reusable classes
To compile code
To store user data
Q33
Q33 What is an assembly in .NET?
A text file
A set of reusable code units
An HTML page
A user password
Q34
Q34 Which type of assembly can be shared across applications in .NET?
Private assembly
Global assembly
Local assembly
Static assembly
Q35
Q35 Which file extension is commonly used for .NET assemblies?
.dll
.exe
.cs
.html
Q36
Q36 What is the role of the Global Assembly Cache (GAC) in .NET?
Manages system memory
Executes code
Stores shared assemblies
Generates reports
Q37
Q37 What is the difference between an assembly and a namespace?
Assemblies organize code, namespaces compile code
Namespaces organize code, assemblies contain compiled code
Namespaces compile code, assemblies execute code
Assemblies store runtime data, namespaces create libraries
Q38
Q38 Which command would you use to install an assembly into the Global Assembly Cache?
install-gac assembly.dll
gacutil -i assembly.dll
addgac assembly.dll
import assembly.dll
Q39
Q39 How would you reference an external library in a .NET project?
import library.dll
using library;
AddReference library.dll
#include library.dll
Q40
Q40 In C#, which keyword is used to access classes from an external namespace?
load
import
using
reference
Q41
Q41 A developer encounters a "MissingAssemblyException." What could be a likely cause?
Incorrect file path
Corrupt CLR
Assembly not found
User permission error
Q42
Q42 How can you resolve a version conflict for an assembly in the GAC?
Reinstall .NET
Add assembly manually
Specify version in config file
Change project settings
Q43
Q43 An assembly fails to load due to version incompatibility. What could fix this issue?
Update assembly reference
Modify GAC
Delete library
Change system path
Q44
Q44 Which of the following is a value type in .NET?
string
int
object
dynamic
Q45
Q45 What is the default value of an integer variable in .NET?
null
0
1
undefined
Q46
Q46 Which data type can hold floating-point numbers in .NET?
int
float
char
bool
Q47
Q47 Which keyword in C# represents a data type that can hold any type of data?
var
dynamic
object
string
Q48
Q48 Which of the following data types is immutable in .NET?
int
StringBuilder
List
string
Q49
Q49 Which code snippet declares a boolean variable in C#?
bool isTrue = true;
boolean isTrue = true;
bool isTrue = 1;
bool isTrue: true
Q50
Q50 How would you declare a constant integer variable with a value of 10 in C#?
int constant = 10;
const int constant = 10;
constant int = 10;
readonly int constant = 10;
Q51
Q51 Which C# code snippet demonstrates declaring an array of integers?
int[] numbers = new int[5];
int numbers = new int;
int array numbers;
int[5] numbers;
Q52
Q52 A developer encounters an "OverflowException" when assigning a value. What could be the cause?
Incorrect type
Value exceeds data type limit
Syntax error
Null reference
Q53
Q53 A developer encounters a "NullReferenceException." What is the likely issue?
Variable is null
Memory leak
Data type mismatch
Invalid format
Q54
Q54 An integer variable is displaying unexpected values. What might be a possible cause?
Incorrect data type
Variable not initialized
Overflow
Syntax error
Q55
Q55 Which class is the base class for all exceptions in .NET?
ApplicationException
SystemException
Exception
ErrorException
Q56
Q56 What is the purpose of a try block in .NET?
To execute code unconditionally
To handle exceptions
To contain code that may throw exceptions
To store debug logs
Q57
Q57 Which block is used to execute cleanup code regardless of whether an exception occurs?
catch
finally
try
throw
Q58
Q58 Which of the following exceptions is thrown when accessing an array out of its bounds?
ArgumentException
IndexOutOfRangeException
OverflowException
DivideByZeroException
Q59
Q59 What is the purpose of the throw keyword in .NET exception handling?
To declare an exception
To log an exception
To pass an exception to higher-level code
To stop code execution
Q60
Q60 Which code snippet demonstrates handling a division by zero exception in C#?
try { int x = 10 / 0; }
try { int x = 10 / 0; } catch (DivideByZeroException ex) { }
int x = 10 / 0;
try { x = 10 / 0; }