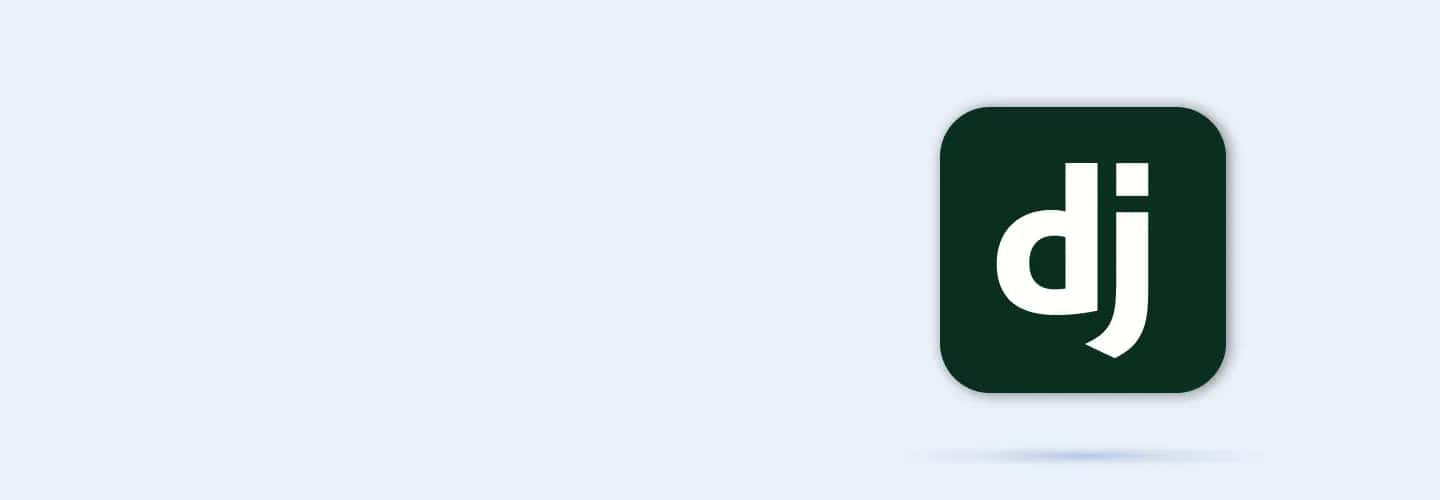
Q121
Q121 What is the primary purpose of middleware in Django?
To handle static files
To process requests and responses
To manage URL routing
To handle database migrations
Q122
Q122 How do you enable middleware in a Django project?
By adding it to the MIDDLEWARE setting
By defining it in urls.py
By adding it to the INSTALLED_APPS setting
By defining it in the views.py file
Q123
Q123 Which method must be implemented in a custom middleware class?
process_request
process_response
call
init
Q124
Q124 What is the role of process_request method in middleware?
To handle static files
To process incoming requests before they reach the view
To manage URL patterns
To handle database queries
Q125
Q125 How can middleware modify the response object?
By overriding the process_response method
By modifying the process_request method
By changing the __init__ method
By using the get_response method
Q126
Q126 How do you create a custom middleware in Django?
By defining a middleware class and adding it to the MIDDLEWARE setting
By creating a middleware function and adding it to the INSTALLED_APPS setting
By defining a middleware class in the urls.py file
By creating a middleware function and adding it to the MIDDLEWARE setting
Q127
Q127 How can you access the request object in a custom middleware?
By using self.request
By passing it to the __init__ method
By implementing the __call__ method and accessing the first parameter
By using self.get_request method
Q128
Q128 What should you check if your custom middleware is not being executed?
The middleware class is defined correctly
The middleware is added to the MIDDLEWARE setting
The process_request method is defined
The process_response method is defined
Q129
Q129 How can you troubleshoot issues with middleware execution order?
By checking the order of middleware classes in the MIDDLEWARE setting
By using print statements
By logging middleware execution
By overriding the __call__ method in all middleware classes
Q130
Q130 What is the primary purpose of deploying a Django application?
To develop the application locally
To make the application accessible to users
To test the application
To manage database migrations
Q131
Q131 Which WSGI server is commonly used for deploying Django applications?
Apache
Nginx
Gunicorn
IIS
Q132
Q132 What is the role of a reverse proxy in Django deployment?
To serve static files
To handle URL routing
To manage database connections
To forward client requests to the application server
Q133
Q133 How do you configure Gunicorn to run a Django application?
By adding Gunicorn to INSTALLED_APPS
By creating a Gunicorn configuration file
By running the gunicorn command with the Django project's WSGI module
By adding Gunicorn to the MIDDLEWARE setting
Q134
Q134 How do you serve static files in a production Django environment?
By using the collectstatic command and configuring the web server to serve the files
By adding static file paths to INSTALLED_APPS
By setting DEBUG = True
By using the runserver command
Q135
Q135 What should you check if your Django application is not accessible after deployment?
The server logs for errors
The database connection settings
The static file configuration
All of these
Q136
Q136 How do you troubleshoot a 502 Bad Gateway error when using Gunicorn with Nginx?
Check the Gunicorn logs for errors
Check the Nginx configuration
Check the Gunicorn configuration
All of these
Q137
Q137 What is the primary goal of performance optimization in Django?
To improve database design
To reduce code complexity
To increase application speed and efficiency
To enhance user authentication
Q138
Q138 Which Django feature can help reduce the number of database queries?
Middleware
Caching
Signal handlers
Form validation
Q139
Q139 What is the purpose of database indexing in Django?
To ensure data consistency
To speed up query performance
To handle URL routing
To manage static files
Q140
Q140 How do you enable query caching in Django?
By using the cache framework and configuring a cache backend
By setting DEBUG = False
By adding CACHE to INSTALLED_APPS
By defining cache settings in the urls.py file
Q141
Q141 How can you optimize database access in Django views?
By using select_related and prefetch_related for related objects
By using raw SQL queries
By disabling the ORM
By using form validation
Q142
Q142 What should you check if your Django application is running slowly?
Database query performance
Middleware configuration
Template rendering time
All of these
Q143
Q143 How do you identify slow database queries in Django?
By using the DEBUG setting
By analyzing the database logs
By using Django Debug Toolbar
By enabling query logging in the database settings
Q144
Q144 What is the primary purpose of security best practices in Django?
To improve database performance
To reduce code complexity
To protect the application from security threats
To manage user authentication
Q145
Q145 Which middleware is used to prevent cross-site request forgery (CSRF) attacks in Django?
AuthenticationMiddleware
CSRFViewMiddleware
XSSProtectionMiddleware
SessionMiddleware
Q146
Q146 What is the role of the SECURE_SSL_REDIRECT setting in Django?
To enforce HTTPS connections
To enable secure cookies
To validate form inputs
To configure database connections
Q147
Q147 How do you set a custom user model in Django?
By defining a CustomUser model and setting AUTH_USER_MODEL in settings.py
By overriding the default User model
By modifying the INSTALLED_APPS setting
By creating a user form
Q148
Q148 How can you secure sensitive data in Django settings?
By using environment variables and secret management tools
By hardcoding sensitive data in settings.py
By using global variables
By storing sensitive data in the database
Q149
Q149 What should you check if your Django application is vulnerable to XSS attacks?
The use of safe filter in templates
The use of mark_safe in the code
The input validation and sanitization
All of these
Q150
Q150 How do you troubleshoot a CSRF token missing error in Django?
Check if CSRFViewMiddleware is enabled
Check if the form contains {% csrf_token %}
Check if the CSRF token is included in AJAX requests
All of these