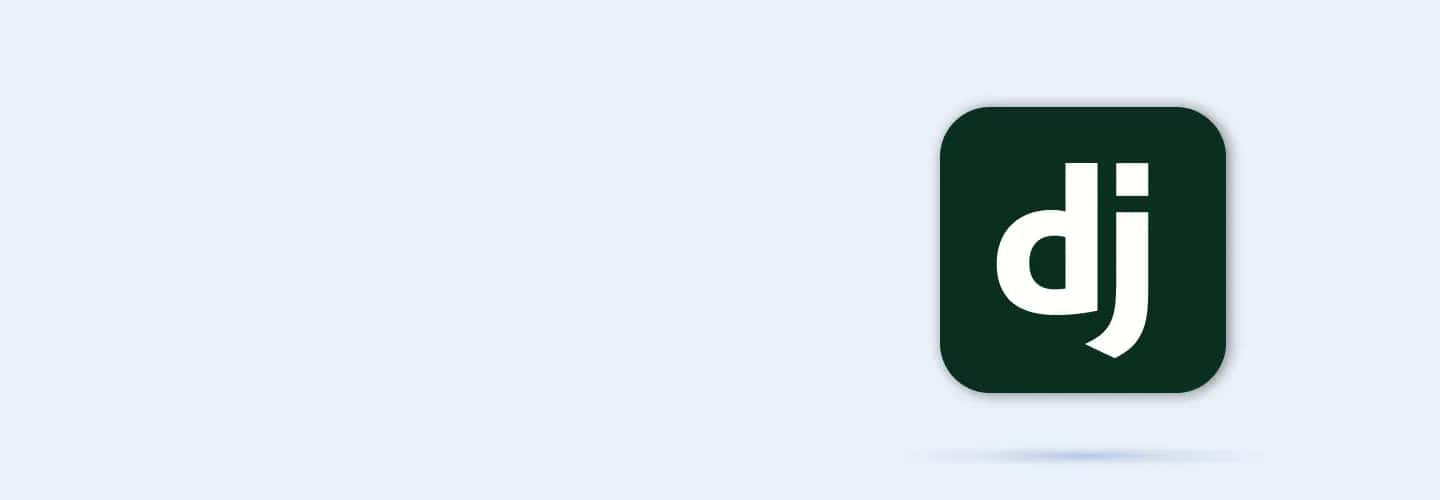
Q91
Q91 What is the primary purpose of Django's admin interface?
To manage static files
To provide a web-based interface for managing database content
To handle URL routing
To manage user authentication
Q92
Q92 How do you register a model with the Django admin site?
By adding the model to the INSTALLED_APPS setting
By defining a register method in the model
By using the admin.site.register method
By using the admin.register decorator
Q93
Q93 What is the purpose of the Admin class in Django?
To define URL patterns for the admin site
To customize the display of a model in the admin interface
To handle form validation in the admin site
To manage user authentication in the admin site
Q94
Q94 How can you customize the list display of a model in the Django admin?
By defining a list_display attribute in the Admin class
By overriding the display method in the model
By adding a display_fields attribute in the Admin class
By defining a list_fields attribute in the Admin class
Q95
Q95 How do you make a model field read-only in the Django admin?
By defining a readonly_fields attribute in the Admin class
By overriding the save method in the model
By defining a read_only method in the Admin class
By adding the field to the readonly attribute in the model
Q96
Q96 How do you filter the records displayed in the admin list view?
By defining a list_filter attribute in the Admin class
By defining a filter method in the model
By adding the filter conditions to the model's Meta class
By using the filter_by attribute in the Admin class
Q97
Q97 How do you add a search functionality to the admin list view?
By overriding the search method in the model
By defining a search_fields attribute in the Admin class
By using the search_by attribute in the Admin class
By adding the search conditions to the model's Meta class
Q98
Q98 You cannot see a model in the Django admin site.
What is a likely cause?
The model does not have a primary key
The model is not added to INSTALLED_APPS
The model is not registered in the admin site
The model's __str__ method is missing
Q99
Q99 What should you check if the Django admin site is not loading properly?
The admin app is included in INSTALLED_APPS
The admin URL patterns are defined correctly
The admin templates are not overridden
The admin models are correctly registered
Q100
Q100 What is the primary purpose of testing in Django?
To manage static files
To verify that your code works as expected
To handle URL routing
To manage user sessions
Q101
Q101 Which Django class is used to create a test case?
Test
TestCase
UnitTest
TestSuite
Q102
Q102 What is the purpose of the setUp method in a Django test case?
To define test cases
To initialize test conditions
To clean up after tests
To handle form validation
Q103
Q103 How do you run tests for a Django application?
python manage.py runtests
python manage.py test
python manage.py run
python manage.py testapp
Q104
Q104 How do you create a simple test case in Django?
class MyTest(TestCase): def test_example(self): self.assertEqual(1 + 1, 2)
class MyTest(Test): def test_example(self): self.assertEqual(1 + 1, 2)
class MyTest(UnitTest): def test_example(self): self.assertEqual(1 + 1, 2)
class MyTest(TestSuite): def test_example(self): self.assertEqual(1 + 1, 2)
Q105
Q105 How do you test a Django view using the test client?
response = self.client.post('/url/')
response = self.client.get('/url/')
response = self.client.fetch('/url/')
response = self.client.view('/url/')
Q106
Q106 How do you test a form submission in Django using the test client?
response = self.client.submit('/url/', data)
response = self.client.post('/url/', data)
response = self.client.send('/url/', data)
response = self.client.put('/url/', data)
Q107
Q107 You receive an "AssertionError" when running a test.
What is a likely cause?
The test conditions are not met
The test method is not defined
The test case is not registered
The test client is not initialized
Q108
Q108 How can you check for expected exceptions in a Django test case?
Using the self.assertException method
Using the self.assertRaises method
Using the self.checkException method
Using the self.expectException method
Q109
Q109 What should you check if your Django tests are not discovering test files?
The test file names start with test_
The test methods start with test_
The test files are in the correct directory
The test class inherits from TestCase
Q110
Q110 How do you test the output of a custom management command in Django?
By writing the output to a log file and checking the log
By using the self.assertCommand method
By running the command and capturing the output with StringIO
By using the self.runCommand method
Q111
Q111 What is the primary purpose of Django REST Framework (DRF)?
To manage static files
To build APIs for web applications
To handle URL routing
To manage user authentication
Q112
Q112 Which class is used to create a serializer in Django REST Framework?
Serializer
ModelSerializer
FormSerializer
JSONSerializer
Q113
Q113 What is the purpose of the APIView class in DRF?
To handle form submissions
To define URL patterns
To manage user authentication
To define views for handling API requests
Q114
Q114 How do you specify which fields to include in a DRF serializer?
By defining a fields attribute in the serializer class
By defining a Meta class in the serializer
By defining the fields in the model class
By using the include parameter in the serializer
Q115
Q115 What is the use of the @api_view decorator in DRF?
To restrict access to authenticated users only
To specify allowed HTTP methods for a function-based view
To handle form validation
To manage user permissions
Q116
Q116 How do you create a view in DRF that returns JSON data?
By using the JSONView class
By using the APIView class and returning a Response object
By using the JsonResponse class
By using the View class and returning a JSON string
Q117
Q117 How do you create a serializer for a model in DRF?
By defining a ModelSerializer class and specifying the model in the Meta class
By defining a Serializer class and specifying the model fields
By using the create_serializer function
By using the model_serializer decorator
Q118
Q118 How do you add pagination to a DRF view?
By defining a Pagination class and adding it to the view
By setting the pagination_class attribute in the view
By using the paginate_by decorator
By adding pagination settings to the settings.py file
Q119
Q119 What should you check if your DRF view is not returning the expected data?
The view's queryset
The serializer's fields attribute
The view's get method
The request method used
Q120
Q120 How do you handle validation errors in a DRF serializer?
By raising a ValidationError exception in the validate method
By overriding the is_valid method
By using the clean method
By defining a custom error handler