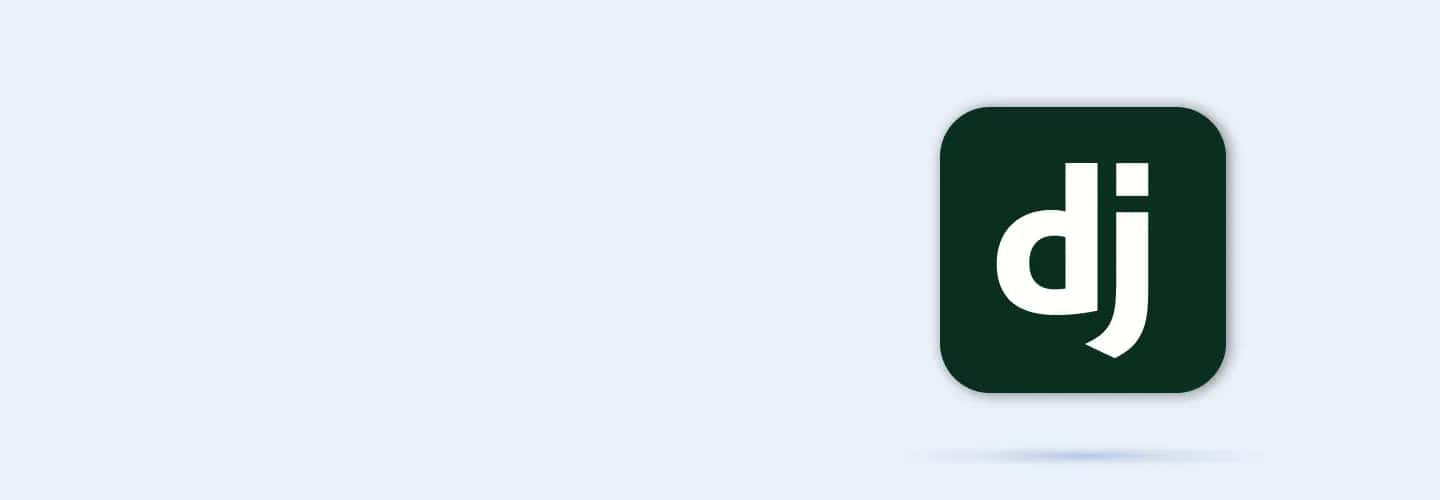
Q61
Q61 Which method is used to save an object to the database in Django?
save()
create()
commit()
store()
Q62
Q62 What is the purpose of the str method in a Django model?
To define the string representation of the model
To define model fields
To handle form validation
To manage URL patterns
Q63
Q63 What does the related_name attribute in a Django model field specify?
The name of the related model
The type of the relationship
The name of the reverse relation from the related model back to this one
The database column name
Q64
Q64 How do you define a CharField with a maximum length of 100 characters in a Django model?
models.CharField(max_length=100)
models.CharField(length=100)
models.CharField(max_length=100, unique=True)
models.CharField(size=100)
Q65
Q65 How do you create a one-to-many relationship in Django?
models.ForeignKey('RelatedModel')
models.OneToOneField('RelatedModel')
models.ManyToManyField('RelatedModel')
models.CharField('RelatedModel')
Q66
Q66 How do you define a model with an auto-incrementing primary key in Django?
models.IntegerField(primary_key=True)
models.AutoField(primary_key=True)
models.CharField(primary_key=True)
models.AutoIncrementField(primary_key=True)
Q67
Q67 How do you create a many-to-many relationship in Django?
models.ManyToManyField('RelatedModel')
models.ForeignKey('RelatedModel')
models.OneToOneField('RelatedModel')
models.ManyToOneField('RelatedModel')
Q68
Q68 You encounter an IntegrityError when saving an object.
What is a likely cause?
The object does not have a primary key
A required field is missing a value
The database is disconnected
The object has an invalid field type
Q69
Q69 How can you check for any pending migrations in Django?
python manage.py checkmigrations
python manage.py showmigrations
python manage.py check
python manage.py listmigrations
Q70
Q70 What might cause a ProgrammingError related to a missing table when running a query?
The table was dropped manually
Migrations were not applied
The database is corrupted
The table name is misspelled
Q71
Q71 What is the primary purpose of forms in Django?
To manage database schemas
To handle URL routing
To handle user input and validation
To manage static files
Q72
Q72 Which Django class is used to create a form?
Form
ModelForm
FormView
FormHandler
Q73
Q73 What is the role of the ModelForm class in Django?
To create a form based on a model
To create a model based on a form
To handle form submissions
To manage form validation
Q74
Q74 How do you specify a custom validation method for a form field in Django?
By defining a clean_
By defining a validate_
By overriding the validate method in the form class
By adding the validation logic in the model class
Q75
Q75 Which method is used to check if a form is valid in Django?
is_valid()
validate()
clean()
is_clean()
Q76
Q76 How do you create a form with a CharField in Django?
forms.CharField(max_length=100)
models.CharField(max_length=100)
fields.CharField(max_length=100)
forms.CharField(length=100)
Q77
Q77 How do you create a ModelForm for a model named Article?
forms.ArticleForm(model=Article)
forms.ModelForm(model=Article)
class ArticleForm(forms.ModelForm): model = Article
class ArticleForm(forms.ModelForm): class Meta: model = Article
Q78
Q78 How do you add a custom validation rule for a field in a Django form?
By defining a custom validator function and adding it to the field's validators list
By defining a validate_
By overriding the validate method in the form class
By adding the validation logic in the model class
Q79
Q79 You receive a "This field is required" error when submitting a form.
What is the most likely cause?
The field is not included in the form submission
The field has a default value
The form is not valid
The field type is incorrect
Q80
Q80 You receive a "ValidationError" when trying to save a form.
What should you check?
The form's is_valid() method
The form's cleaned_data attribute
The model's clean() method
The form's full_clean() method
Q81
Q81 How can you identify which form fields are causing validation errors in Django?
By printing the form's errors attribute
By checking the form's is_valid() method
By reviewing the form's cleaned_data attribute
By inspecting the form's error_messages attribute
Q82
Q82 What is the primary purpose of authentication in Django?
To manage URL routing
To handle user sessions
To verify the identity of a user
To manage static files
Q83
Q83 Which Django decorator is used to restrict access to authenticated users only?
@authenticated
@login_required
@require_user
@auth_user
Q84
Q84 What is the purpose of Django's User model?
To define URL patterns
To manage user authentication and permissions
To handle form validation
To manage static files
Q85
Q85 How can you add custom permissions to a Django model?
By defining permissions in the model's Meta class
By adding permissions to the User model
By using the @permission_required decorator
By overriding the save method of the model
Q86
Q86 How do you create a superuser in Django?
python manage.py createsuperuser
python manage.py adduser
python manage.py createsuper
python manage.py superuser
Q87
Q87 How do you log in a user programmatically in a Django view?
user_login()
login()
authenticate()
auth_login()
Q88
Q88 How do you restrict access to a view to users with a specific permission in Django?
@permission_required('app.permission')
@login_required('app.permission')
@auth_permission('app.permission')
@user_passes_test('app.permission')
Q89
Q89 You receive a "Permission Denied" error when accessing a view.
What is a likely cause?
The user is not authenticated
The user does not have the required permission
The URL is incorrect
The view is not decorated with @login_required
Q90
Q90 What should you check if the login_required decorator is not redirecting to the login page?
The LOGIN_URL setting
The view's URL pattern
The template name
The user's permissions