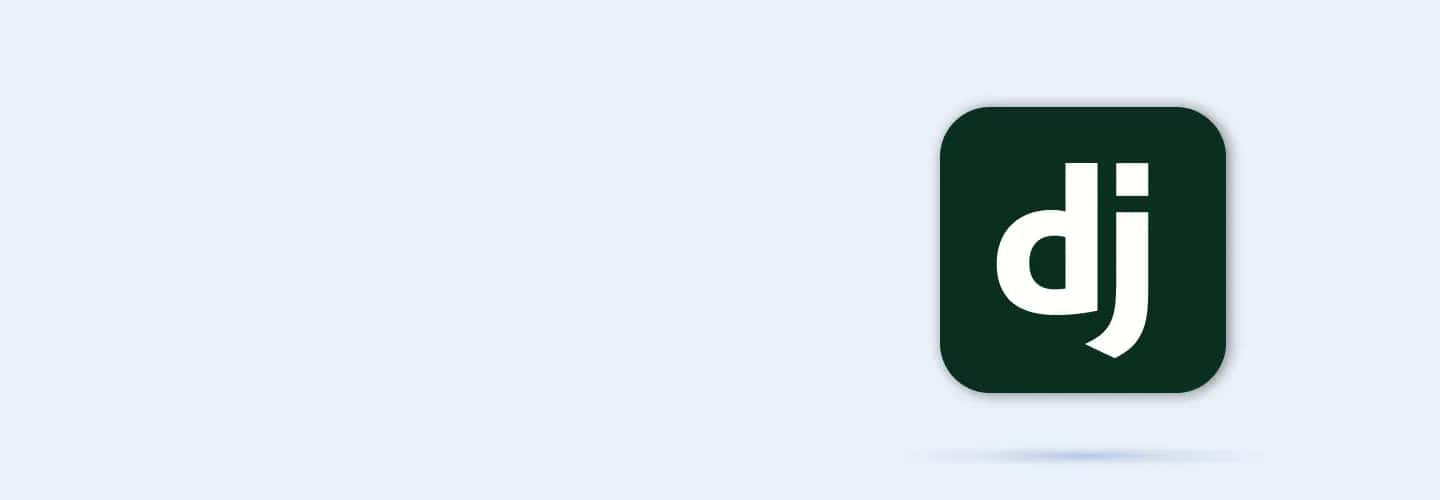
Q1
Q1 What is the primary purpose of Django in web development?
To provide a framework for client-side scripting
To create static HTML websites
To facilitate the rapid development of secure and maintainable web applications
To manage client-side databases
Q2
Q2 Which design pattern does Django follow?
Model-View-Controller (MVC)
Model-View-Presenter (MVP)
Model-Template-View (MTV)
Model-View-ViewModel (MVVM)
Q3
Q3 What is Django's primary language?
Java
C++
Python
Ruby
Q4
Q4 Which of the following is a core feature of Django?
Automatic admin interface
Client-side form validation
Real-time data processing
Mobile application support
Q5
Q5 What command is used to start a new Django project?
django-admin startapp
django-admin startproject
django startproject
django startapp
Q6
Q6 Which file in a Django project contains the project's settings?
views.py
urls.py
settings.py
models.py
Q7
Q7 How does Django ensure security in its framework?
By using client-side scripting
By providing built-in security features like CSRF protection
By requiring developers to write security code manually
By relying on external security plugins
Q8
Q8 What is the role of the Django ORM?
To manage client-side databases
To handle HTTP requests
To map database schema to Python objects
To render templates
Q9
Q9 Which Django template tag is used to insert the value of a variable into the template?
{{ variable }}
{% variable %}
{% include variable %}
{{ include variable }}
Q10
Q10 A Django application throws an ImproperlyConfigured error at startup.
What is the most likely cause?
Database server is down
Missing a required setting in settings.py
URL configuration is incorrect
Incorrect template syntax
Q11
Q11 Which command is used to install Django via pip?
pip install django
pip get django
pip fetch django
pip import django
Q12
Q12 What is the default port number used when running the Django development server?
8000
8080
5000
3000
Q13
Q13 Which of the following is NOT a valid way to start the Django development server?
python manage.py runserver
python manage.py startserver
python manage.py runserver 0.0.0.0:8000
django-admin runserver
Q14
Q14 What is the purpose of the django-admin command-line utility?
To create static files
To handle database migrations
To manage Django projects and apps
To start a web server
Q15
Q15 Which file is automatically generated when you create a new Django project?
views.py
urls.py
settings.py
manage.py
Q16
Q16 What is the purpose of the INSTALLED_APPS setting in Django?
To list the apps that are available for installation
To list the apps that are enabled in the project
To list the apps that are part of the Django framework
To list the apps that are external dependencies
Q17
Q17 Which Python version is required for Django 3.0 and above?
Python 2.7
Python 3.5
Python 3.6
Python 3.8
Q18
Q18 How do you create a new Django app named "blog"?
django-admin startproject blog
django-admin createapp blog
python manage.py startapp blog
python manage.py createapp blog
Q19
Q19 Which command is used to apply database migrations in Django?
python manage.py migrate
python manage.py makemigrations
python manage.py migrate --apply
python manage.py applymigrations
Q20
Q20 How do you create a new Django project named "myproject"?
django-admin startproject myproject
django-admin createproject myproject
python manage.py startproject myproject
python manage.py createproject myproject
Q21
Q21 A Django project is not recognizing changes in the models.py file.
What is the most likely cause?
The development server is not running
Migrations have not been created or applied
The settings.py file is missing
The database is not connected
Q22
Q22 You receive a "ModuleNotFoundError" when running python manage.py runserver.
What is the most likely cause?
The virtual environment is not activated
The Django version is outdated
The settings.py file is misconfigured
The database server is down
Q23
Q23 When running python manage.py runserver, you get an "Address already in use" error.
How can you resolve this?
Change the port number in the settings.py file
Restart the database server
Use a different port number when running the server
Clear the browser cache
Q24
Q24 What is the purpose of the urls.py file in a Django project?
To define the models
To configure settings
To map URLs to views
To manage static files
Q25
Q25 Which directory typically contains the settings.py file in a Django project?
templates
static
project_name
app_name
Q26
Q26 What is the role of the manage.py file in a Django project?
To handle database migrations
To create new apps
To manage the project from the command line
To configure URL routing
Q27
Q27 Which file contains the WSGI application used by Django’s development server?
settings.py
urls.py
wsgi.py
asgi.py
Q28
Q28 In a Django project, where do you typically define your database models?
views.py
models.py
urls.py
admin.py
Q29
Q29 What is the purpose of the init.py file in a Django app directory?
To configure settings
To initialize the app
To define URL patterns
To manage static files
Q30
Q30 How do you include an app's URL patterns in the main project's urls.py file?
path('app_name/', include('app_name.urls'))
url('app_name/', include('app_name.urls'))
path('app_name/', include(app_name.urls))
include('app_name.urls')