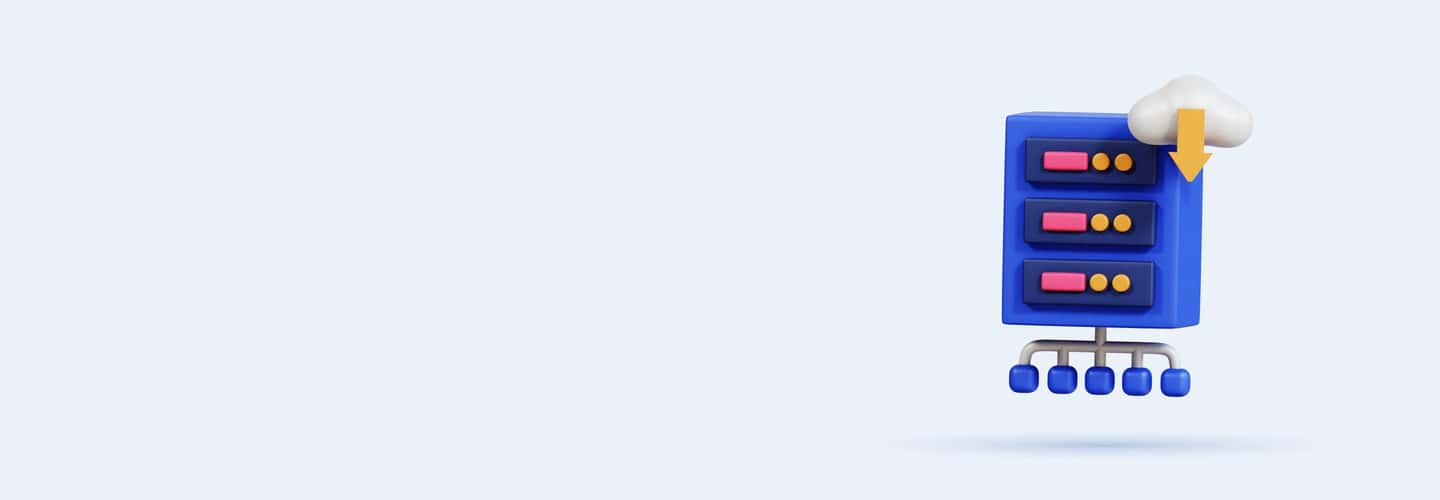
Q91
Q91 In the context of hash tables, what does "load factor" refer to?
The ratio of the number of entries to the number of buckets in the table
The maximum number of collisions allowed before resizing
The percentage of keys that are null
The average search time for an entry
Q92
Q92 What strategy can significantly reduce the chance of collisions in a hash table?
Using a prime number for the size of the hash table
Increasing the size of the keys
Decreasing the number of entries
Using multiple hash functions for the same key
Q93
Q93 How do you access a value stored in a hash table given its key?
By computing the hash of the key and searching linearly
By directly indexing the array with the key
By computing the hash of the key and using it as an index
By sorting the keys and performing a binary search
Q94
Q94 What is the most common method to handle collisions in a hash table programmatically?
Open addressing with linear probing
Storing values in a list at each index
Doubling the hash table size on a collision
Using a secondary hash function
Q95
Q95 How do you ensure that a hash table remains efficient as more entries are added?
By periodically decreasing the table size
By rehashing all entries into a larger table when the load factor reaches a threshold
By limiting the number of entries
By converting the table to a binary search tree on overflow
Q96
Q96 Which approach is best for storing values that have the same hash key in a hash table?
Overwriting the previous value
Linking new values to the existing ones in a linked list
Ignoring new values with duplicate keys
Storing values in an adjacent table
Q97
Q97 A developer notices that retrieval times from a hash table are consistently slow.
What is a likely reason?
The hash function is too complex
The load factor is too high, causing excessive collisions
The keys are not distributed uniformly
All entries are stored in a single bucket
Q98
Q98 During testing, a hash table's add operation sometimes fails to insert new elements.
What could be the problem?
The hash function always returns the same value
Collisions are not handled correctly
The table is full and cannot resize
The key is null
Q99
Q99 A hash table implementation experiences intermittent performance degradation.
What might be causing this issue?
Inconsistent hash function performance
Varying sizes of entries
Periodic table resizing operations
Non-uniform key distribution
Q100
Q100 What is the key principle behind dynamic programming?
Breaking down problems into smaller subproblems
Finding the quickest solution without regard for correctness
Using recursion exclusively
Memorizing intermediate results
Q101
Q101 In which scenario would a greedy algorithm be preferred over dynamic programming?
When an optimal solution needs to be guaranteed for all cases
When subproblems overlap and are dependent
When subproblems are independent and a local optimum is acceptable
When the problem size is very small
Q102
Q102 What distinguishes a greedy algorithm from a dynamic programming approach?
Greedy algorithms consider all possible solutions before making a choice
Dynamic programming uses recursion to solve subproblems
Greedy algorithms make the locally optimal choice at each step
Dynamic programming cannot handle overlapping subproblems
Q103
Q103 What is memoization in the context of dynamic programming?
Storing the results of expensive function calls and returning the cached result when the same inputs occur again
Randomly selecting subproblems to solve
Optimizing memory usage by deleting unnecessary data
A technique for improving the runtime of greedy algorithms
Q104
Q104 Which problem is a classic example that can be solved efficiently using dynamic programming?
The Traveling Salesman Problem
Sorting algorithms
The Knapsack Problem
Binary search
Q105
Q105 How do greedy algorithms differ in their approach to solving problems compared to brute force methods?
Greedy algorithms evaluate all possible paths before choosing the shortest
Greedy algorithms make a series of localized decisions to find a solution
Brute force methods use recursion exclusively
Brute force methods cannot find global optima
Q106
Q106 In dynamic programming, what is meant by "optimal substructure"?
A problem that can be divided into subproblems, which are not related
A problem that does not have a defined optimal solution
A problem where the optimal solution can be constructed from optimal solutions of its subproblems
A problem that can only be solved in linear time
Q107
Q107 How is the Fibonacci sequence efficiently calculated using dynamic programming?
By using a loop to iteratively calculate each number
By storing each Fibonacci number calculated in an array and using it for future calculations
By recursion without memoization
By guessing and checking
Q108
Q108 What technique is used in dynamic programming to transform a recursive solution into an iterative one?
Memoization
Tabulation
Backtracking
Divide and conquer
Q109
Q109 For a problem with overlapping subproblems and optimal substructure, which approach is most suitable?
Greedy algorithm
Dynamic programming
Divide and conquer
Backtracking
Q110
Q110 What is a key advantage of using dynamic programming over naive recursion for problems like calculating the nth Fibonacci number?
It reduces the computational complexity
It eliminates the need for calculation
It uses less memory
It relies on simpler mathematical concepts
Q111
Q111 Why might a greedy algorithm fail to find the least cost path in a graph?
Because it makes globally optimal choices at each step
Because it makes locally optimal choices without considering the future
Because it evaluates every possible path before making a decision
Because it uses dynamic programming techniques
Q112
Q112 A dynamic programming solution is running slower than expected.
What could be a reason?
The problem does not have overlapping subproblems
Subproblems are not being correctly memoized
There are too many subproblems
The base cases are defined incorrectly
Q113
Q113 What issue could arise when implementing a greedy algorithm for a complex optimization problem?
Overlooking better solutions due to making premature decisions
Incorrectly assuming the problem has overlapping subproblems
Using too much memory
Not using recursion enough
Q114
Q114 What is the primary purpose of Dijkstra's algorithm in graph theory?
To find the shortest path between all pairs of nodes
To detect cycles within the graph
To find the shortest path from a single source to all other nodes in the graph
To create a minimum spanning tree
Q115
Q115 What distinguishes depth-first search (DFS) from breadth-first search (BFS) in graph traversal?
DFS uses a queue, while BFS uses a stack
DFS can find the shortest path; BFS cannot
BFS uses a queue, while DFS uses a stack
DFS is recursive, while BFS cannot be made recursive
Q116
Q116 In graph theory, what is a strongly connected component?
A subset of vertices that are mutually reachable by any other vertex in the subset
A component where each vertex is connected to every other vertex by a direct edge
A set of vertices with no incoming edges
A set of vertices in a directed graph where each vertex has the same degree
Q117
Q117 What does the Bellman-Ford algorithm accomplish?
Finding the shortest path in a graph with negative edge weights
Creating a minimum spanning tree
Finding the maximum flow in a network
Detecting and breaking cycles in a directed graph
Q118
Q118 What is the primary difference between Prim's and Kruskal's algorithms?
Prim's algorithm is used for shortest path finding, while Kruskal's is used for minimum spanning trees
Prim's requires a starting vertex; Kruskal's does not
Prim's is a greedy algorithm; Kruskal's is not
Prim's can handle negative edge weights; Kruskal's cannot
Q119
Q119 Why are topological sorts important in graph algorithms?
They are used to detect cycles in undirected graphs
They provide a way to schedule tasks with dependencies
They find the shortest path in weighted graphs
They compute the maximum flow in networks
Q120
Q120 How do you implement a graph traversal to check if a graph is bipartite?
By using a depth-first search and assigning colors to each node
By finding the shortest path between all pairs of nodes
By creating a minimum spanning tree
By performing a matrix multiplication