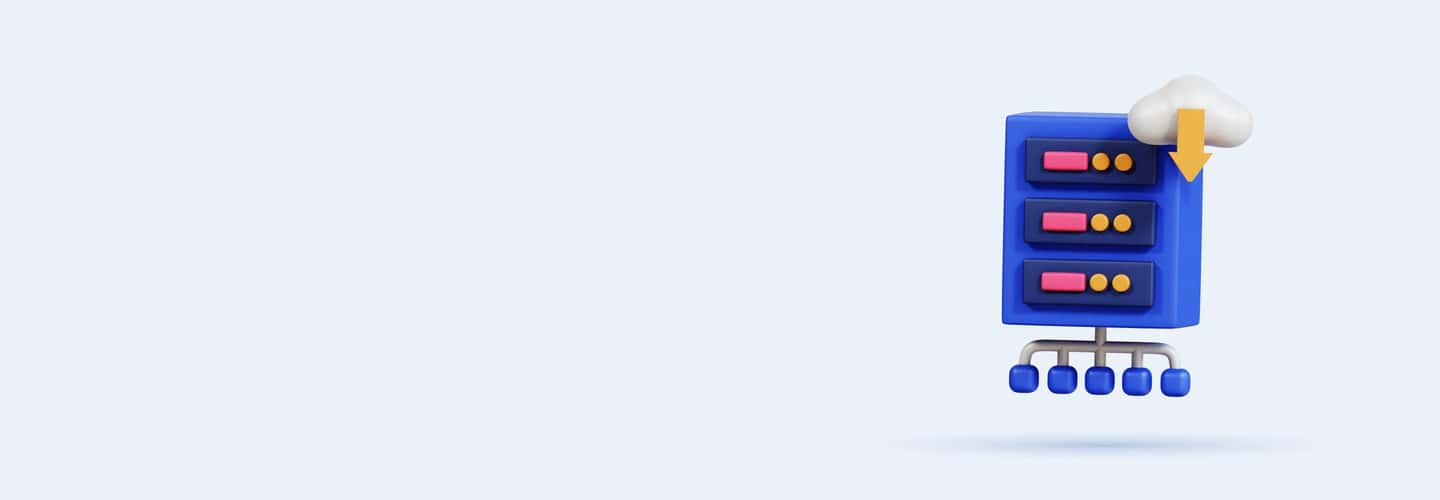
Q61
Q61 Which traversal method is used to visit nodes in a level-by-level manner from left to right in a tree?
Preorder
Inorder
Postorder
Level-order
Q62
Q62 What data structure is best suited for implementing a graph's adjacency list?
Array
Linked list
Hash table
Tree
Q63
Q63 In a directed graph, what does an edge from node A to node B represent?
A bidirectional relationship
A unidirectional relationship from A to B
A hierarchical relationship
A peer-to-peer relationship
Q64
Q64 What is the property of a balanced binary search tree (BST)?
The left and right subtrees' heights differ by at most one
Each subtree is a full tree
Each subtree is a complete binary tree
All leaf nodes are at the same level
Q65
Q65 What is the result of performing an inorder traversal on a binary search tree (BST)?
A random permutation of the tree's elements
The elements of the tree sorted in descending order
The elements of the tree sorted in ascending order
The elements in the order they were inserted
Q66
Q66 How do you find the height of a binary tree?
By counting the number of left children
By counting the number of right children
By calculating the maximum depth from the root to any leaf
By calculating the total number of nodes
Q67
Q67 In graph theory, how is a weighted edge represented in an adjacency list?
As a list of vertex pairs
As a list of vertices with associated edge lists
As a list of tuples, each containing a vertex and the edge weight
As a two-dimensional matrix
Q68
Q68 What algorithm can be used to detect a cycle in a directed graph?
Depth-first search (DFS)
Breadth-first search (BFS)
Kruskal's algorithm
Dijkstra's algorithm
Q69
Q69 You implemented a tree but notice that child nodes are not correctly associated with their parents.
What might be the issue?
The tree is incorrectly initialized as a graph
Child nodes are added to the wrong parent
The tree structure does not support hierarchy
Nodes are not properly linked
Q70
Q70 A graph's adjacency matrix does not reflect the correct connections between nodes.
What is a possible mistake?
The matrix dimensions are incorrect
Edges are added to the wrong cells in the matrix
The matrix is not updated when edges are added or removed
Both B and C
Q71
Q71 In a depth-first search (DFS) implementation for a graph, you find that the algorithm does not visit all nodes.
What could be wrong?
The algorithm does not account for disconnected components
The starting node is chosen incorrectly
The visitation markers are not reset between runs
The recursion depth is limited
Q72
Q72 What is the best-case time complexity of QuickSort?
O(n log n)
O(n)
O(log n)
O(n^2)
Q73
Q73 Which sorting algorithm is inherently stable?
QuickSort
HeapSort
MergeSort
BubbleSort
Q74
Q74 What does "stability" in sorting algorithms refer to?
Performance under stress
Maintaining relative order of equal elements
Minimal memory usage
Fastest possible sorting time
Q75
Q75 What is the worst-case time complexity of binary search on a sorted array of n elements?
O(1)
O(log n)
O(n)
O(n log n)
Q76
Q76 Which sorting algorithm is most efficient for a dataset with a known, limited range of integer values?
QuickSort
BubbleSort
CountingSort
InsertionSort
Q77
Q77 What principle do divide and conquer sorting algorithms utilize?
Splitting data into smaller parts and individually sorting them
Random element selection
Linear traversal
Direct element swapping
Q78
Q78 Why is QuickSort generally preferred over MergeSort for sorting arrays in practice?
Lower space complexity
Faster average sorting times
Simpler to implement
Stable sorting guaranteed
Q79
Q79 Which line correctly initializes a pivot in QuickSort for an array segment between low and high?
int pivot = arr[high];
int pivot = arr[(low + high) / 2];
int pivot = arr[low];
int pivot = high;
Q80
Q80 What technique improves QuickSort's performance on small arrays?
Insertion sort hybrid
Random pivot selection
Decreasing recursion depth
Increasing stack size
Q81
Q81 What action does the merge function in MergeSort primarily perform?
Splitting the array
Sorting the subarrays
Merging the sorted subarrays
Comparing each element
Q82
Q82 How is a binary search algorithm adapted for a rotated sorted array?
Adjust search based on middle element comparison
Double the search range every step
Search only one half of the array
Re-sort the array before searching
Q83
Q83 A developer's InsertionSort algorithm isn't sorting correctly.
What's a common error to check?
Misplacing the key element
Incorrect index calculation
Skipping elements
Incorrectly comparing elements
Q84
Q84 In a flawed binary search implementation, the search misses the target.
What's a likely cause?
Incorrect middle index calculation
Not checking the sorted array's bounds
Ignoring the target comparison
All conditions are met but still failing
Q85
Q85 QuickSort is causing a stack overflow error.
What's a probable cause?
Excessive recursion on large datasets
Incorrect pivot selection leading to unbalanced partitions
Non-terminating recursive calls
All conditions are met but still failing
Q86
Q86 What is a hash table?
A data structure that stores key-value pairs in a linear array
A data structure that organizes data for quick search, insertion, and deletion based on keys
A data structure for storing hierarchical data
A data structure that stores data in nodes with a key and multiple values
Q87
Q87 Which of the following is a common use case for a hash table?
Implementing a database indexing system
Storing preferences of a user in a web application
Performing quick searches in a large dataset
All of the above
Q88
Q88 What is a collision in a hash table?
When two keys have the same value
When a hash function returns the same index for different keys
When a hash table exceeds its load factor
When a key is lost due to overwriting
Q89
Q89 What is the primary challenge in designing a hash function for a hash table?
Ensuring it is reversible
Minimizing the occurrence of collisions
Ensuring it produces a unique output for each input
Maximizing the computational complexity
Q90
Q90 Which technique is commonly used to resolve collisions in a hash table?
Linear probing
Using a binary search tree
Doubling the size of the table when full
Storing all entries in a single linked list