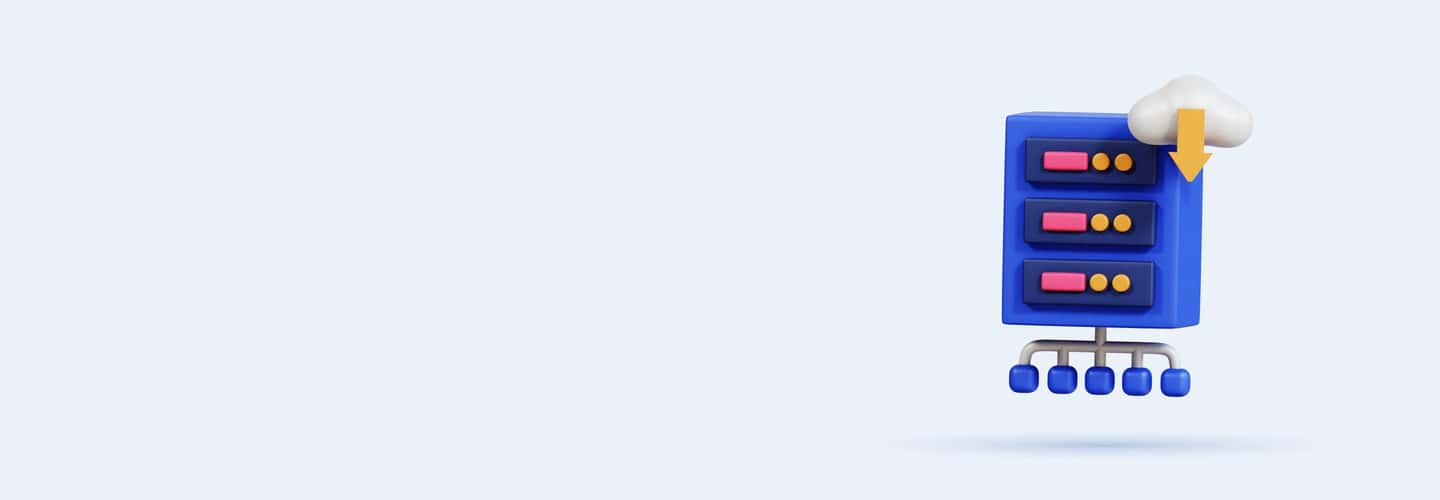
Q31
Q31 A programmer expects the following JavaScript code to update an array but it does not:
const arr = [1, 2, 3]; arr = [4, 5, 6];.
What is the mistake?
Attempting to reassign a constant array
Incorrect syntax for array update
Using the wrong data type
None of the above
Q32
Q32 Why does string.split('').reverse().join('') in JavaScript return a reversed string?
The split method incorrectly splits the string
The reverse method does not work on strings
The join method concatenates incorrectly
None of the above
Q33
Q33 In a program designed to find the longest string in an array of strings, the output is always the first string. What is the likely error?
Not updating the longest string variable inside the loop
Using the wrong comparison operator
Not initializing the longest string variable
All of the above
Q34
Q34 What distinguishes a singly linked list from a doubly linked list?
Each node has one pointer in a singly linked list and two in a doubly linked list
Singly linked lists are faster
Doubly linked lists cannot have cycles
All of the above
Q35
Q35 What operation is typically more efficient in a linked list compared to an array?
Accessing an element by index
Appending an element to the end
Inserting an element at the beginning
Searching for an element
Q36
Q36 Which of the following scenarios is a linked list NOT suitable for?
When elements need to be accessed sequentially
When memory usage is a concern
When fast access to elements by index is required
When adding or removing elements frequently
Q37
Q37 In a linked list, what does the head pointer signify?
The middle node of the list
The last node of the list
The first node of the list
A random node in the list
Q38
Q38 How do you detect a cycle in a linked list?
By checking if the next pointer of any node is null
Using a hash table to store visited nodes
Comparing each node with every other node
Using two pointers at different speeds
Q39
Q39 What is the time complexity of finding the middle element in a singly linked list?
O(1)
O(n)
O(log n)
O(n^2)
Q40
Q40 What does the following code snippet do?
node.next = node.next.next;
Deletes the next node in the list
Inserts a new node after the current one
Swaps two nodes
Duplicates the next node
Q41
Q41 Consider a linked list implementation.
What does head = newNode;
newNode.next = oldHead;
accomplish?
Reverses the linked list
Adds a new node to the end of the list
Adds a new node at the beginning of the list
Deletes the head node
Q42
Q42 In a doubly linked list, each node has a value, a prev, and a next pointer.
How do you insert a new node after a given node?
Update givenNode.next and newNode.prev
Update givenNode.next, newNode.next, newNode.prev, and the next node's prev
Only update newNode.next
Only update newNode.prev
Q43
Q43 A linked list's remove method always deletes the second node, regardless of the input. What is the likely mistake?
Incorrectly updating pointers
Using the wrong comparison operator
Forgetting to check if the list is empty
All of the above
Q44
Q44 Why might a find function in a linked list return null for existing values?
The comparison logic is incorrect
It starts at the wrong node
It skips over nodes
Any of the above
Q45
Q45 In a function intended to add a node at a specific index in a linked list, the node is added at the end regardless of the index.
What's the error?
Not iterating through the list correctly
Not checking if the index is within bounds
Both A and B
None of the above
Q46
Q46 Which data structure uses a FIFO (First In, First Out) approach?
Stack
Queue
Array
Linked List
Q47
Q47 What is the primary difference between a stack and a queue?
The way they store data
The way they access data
Their size limitations
Their implementation complexity
Q48
Q48 In a queue, what operations correspond to adding and removing elements?
push and pop
enqueue and dequeue
insert and delete
add and remove
Q49
Q49 Which of the following is a real-world example of a stack?
A line of people at a ticket booth
The arrangement of plates in a cafeteria
A playlist of songs
The queue at a bus stop
Q50
Q50 How can a stack be implemented?
Using an array or a linked list
Using a hash table
Using a binary tree
Using direct memory access
Q51
Q51 What is the time complexity of accessing the bottom element of a stack?
O(1)
O(n)
O(log n)
O(n^2)
Q52
Q52 Which operation is used to add an element to the top of a stack?
push
pop
enqueue
dequeue
Q53
Q53 Consider a stack implementation. What does the following operation do?
stack.pop()
Adds an element to the stack
Removes the top element from the stack
Peeks at the top element without removing it
Checks if the stack is empty
Q54
Q54 How do you implement a queue using two stacks, stack1 and stack2?
By alternating elements between stack1 and stack2
By pushing elements to stack1 and popping them to stack2 for dequeuing
By using stack1 for enqueue and stack2 for dequeue
None of the above
Q55
Q55 A queue implementation returns incorrect elements when dequeuing.
What could be the problem?
The enqueue operation places elements at the front
The dequeue operation removes elements from the wrong end
Both A and B
None of the above
Q56
Q56 In a stack, the pop operation sometimes returns the correct element and sometimes returns null.
What is the likely error?
The stack is not correctly checking for underflow conditions
The push operation is inconsistent
The stack is overwriting elements
All of the above
Q57
Q57 A stack implemented using an array throws an index out of bounds exception.
What is the most probable cause?
Incorrectly initializing the stack size
Exceeding the stack capacity without resizing
Incorrect index calculation for push/pop
All of the above
Q58
Q58 What feature distinguishes binary trees from other tree types?
Each node has at most two children
Each node has exactly two children
Nodes are organized in binary
Nodes contain binary data
Q59
Q59 Where do you find the smallest element in a binary search tree (BST)?
The leftmost leaf
The rightmost leaf
The root node if the tree is balanced
Directly under the root node if the tree is complete
Q60
Q60 What is a key difference between graphs and trees?
Trees contain cycles, while graphs do not
Graphs contain cycles, while trees do not
Trees can only have one root, while graphs can have multiple roots
Graphs are hierarchical structures, while trees are not