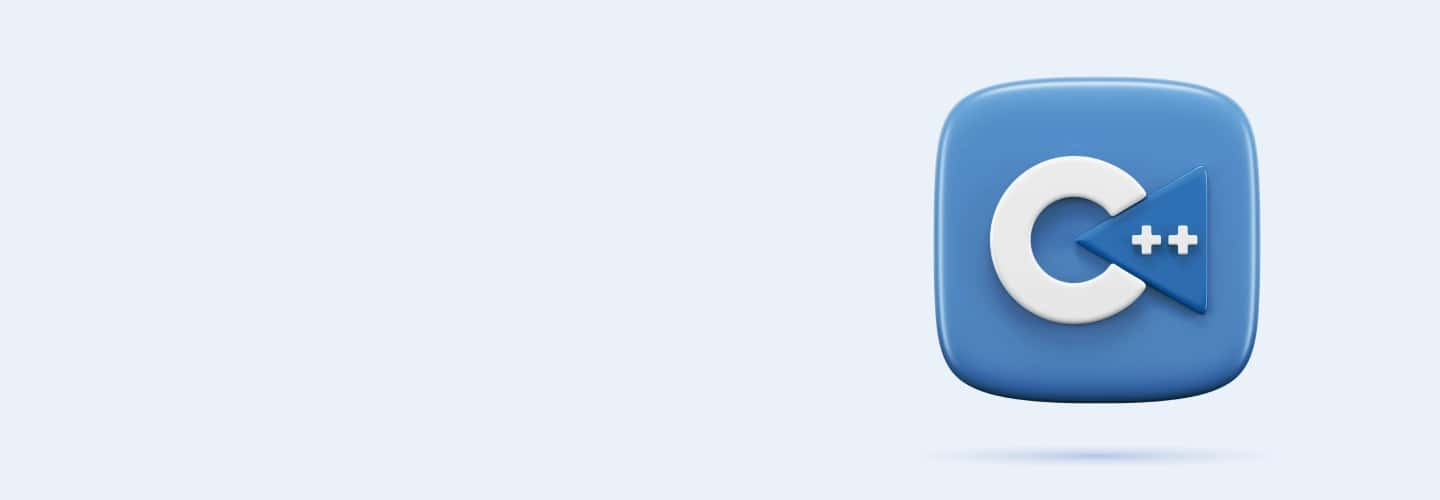
Q121
Q121 Spot the error:
int *ptr;
*ptr = new int;
*ptr = 5;
delete ptr;
Dereferencing an uninitialized pointer
Syntax error in memory allocation
No error
Incorrect use of delete
Q122
Q122 What is a function template in C++?
A predefined function in the C++ Standard Library
A special function used for debugging
A way to create functions with a generic type
A container in the STL
Q123
Q123 What is a class template in C++?
A class defined inside another class
A class that serves as a blueprint for creating other classes
A class that can handle multiple data types generically
A class used exclusively for STL operations
Q124
Q124 Which STL container is typically used for implementing a dynamic array?
std::map
std::vector
std::list
std::set
Q125
Q125 How does the std::map container in C++ STL store elements?
In a single list
In key-value pairs, with unique keys
As an array of elements
In a stack-like structure
Q126
Q126 What is the primary difference between std::list and std::vector in C++ STL?
std::list is for integer types only, while std::vector can hold any type
std::list provides sequential access, while std::vector provides random access
std::list is a dynamic array, while std::vector is a linked list
std::list is a doubly linked list, while std::vector is a dynamic array
Q127
Q127 In C++ STL, what is an iterator?
A loop that iterates over a container
A pointer-like object used to access elements in a container
A function to traverse arrays
A class template for container classes
Q128
Q128 What distinguishes std::set from other STL containers?
It allows for rapid insertion of elements
It automatically sorts the elements in ascending order
It stores each element in duplicate
It is the only STL container that uses templates
Q129
Q129 Why are templates used in C++?
To create error-free code
To provide predefined functionality
To allow classes and functions to operate with generic types
To optimize code execution
Q130
Q130 Given the C++ code snippet:
template
return a + b;
}
What is this an example of?
A class template
A function template
An overloaded operator
An STL container
Q131
Q131 Identify the issue in this C++ code:
std::vector
for (int i = 0; i <= v.size(); ++i) {
cout << v[i]; }
Using a wrong loop condition
Accessing elements outside the vector's bounds
Incorrect use of the vector container
No error
Q132
Q132 Spot the error:
template
T value; void setValue(T val) { value = val; }
};
Incorrect template syntax
Missing constructor
No error
Error in setValue function
Q133
Q133 What is the purpose of exception handling in C++?
To handle syntax errors in the code
To increase the execution speed of programs
To manage runtime errors in a controlled way
To debug the program
Q134
Q134 What keyword is used in C++ to throw an exception?
throw
error
exception
exit
Q135
Q135 Which block of code is used to handle exceptions in C++?
try block
catch block
throw block
error block
Q136
Q136 What is the correct way to handle multiple exceptions in C++?
Using multiple throw statements
Using several try blocks
Using multiple catch blocks after a single try block
Using nested try-catch blocks
Q137
Q137 In C++, what happens if an exception is not caught?
The program will continue to execute
The exception gets rethrown automatically
The program terminates abnormally
The exception is ignored
Q138
Q138 What is the use of the std::exception class in C++?
To terminate a program
To catch syntax errors
As a base class for standard exception types
To declare new variables
Q139
Q139 What is the output of this code?
try { throw 20; } catch (int e) {
cout << "An exception occurred. Exception Nr. " << e;
}
An error message
An exception occurred. Exception Nr. 20
20
The program crashes
Q140
Q140 Given the C++ code snippet:
try { int* myarray = new int[1000]; } catch (std::bad_alloc& e) {
cout << "Allocation failed: " << e.what();
},
what does this code do?
It handles an out-of-memory error
It creates an array of 1000 integers
It throws a bad_alloc exception
It catches syntax errors
Q141
Q141 Identify the issue in this code:
try { throw "Error occurred"; } catch (...) {
cout << "An error occurred";
}
The catch block does not specify the exception type
The throw statement is incorrect
There is no error
The try block is empty
Q142
Q142 Spot the error:
try { int x = -1;
if (x < 0) { throw x; } } catch (unsigned int e) {
cout << "Caught an exception: " << e; }
The throw statement should not throw integers
The catch block is catching the wrong type of exception
There is no error
The if condition is incorrect
Q143
Q143 What is the purpose of namespaces in C++?
To optimize code execution speed
To handle exceptions
To avoid name conflicts in larger programs
To manage dynamic memory
Q144
Q144 What are smart pointers in C++?
Pointers that automatically manage memory allocation and deallocation
Pointers that increase execution speed
Arrays with advanced features
Iterators in the STL
Q145
Q145 What is a lambda expression in C++?
A function that cannot have its address taken
A compact way of defining anonymous functions
A template for creating multiple functions
A method in the STL
Q146
Q146 How is multithreading achieved in C++?
Using the std::thread library
By creating multiple processes
Through lambda expressions
By using dynamic memory allocation
Q147
Q147 What are regular expressions used for in C++?
To optimize algorithms
To create complex data structures
To search, edit, or manipulate text
To manage file input/output operations
Q148
Q148 In C++, which smart pointer type automatically deallocates the memory when it is no longer in use?
auto_ptr
shared_ptr
unique_ptr
weak_ptr
Q149
Q149 What is the significance of std::regex in C++?
It is used for creating regular expressions
It is a class template for sequence containers
It is a function for memory allocation
It is a method for string manipulation
Q150
Q150 Given the C++ code snippet:
auto lambda = [](int x, int y) { return x + y; }; cout << lambda(2, 3);,
what is the output?
5
Compilation error
2
3