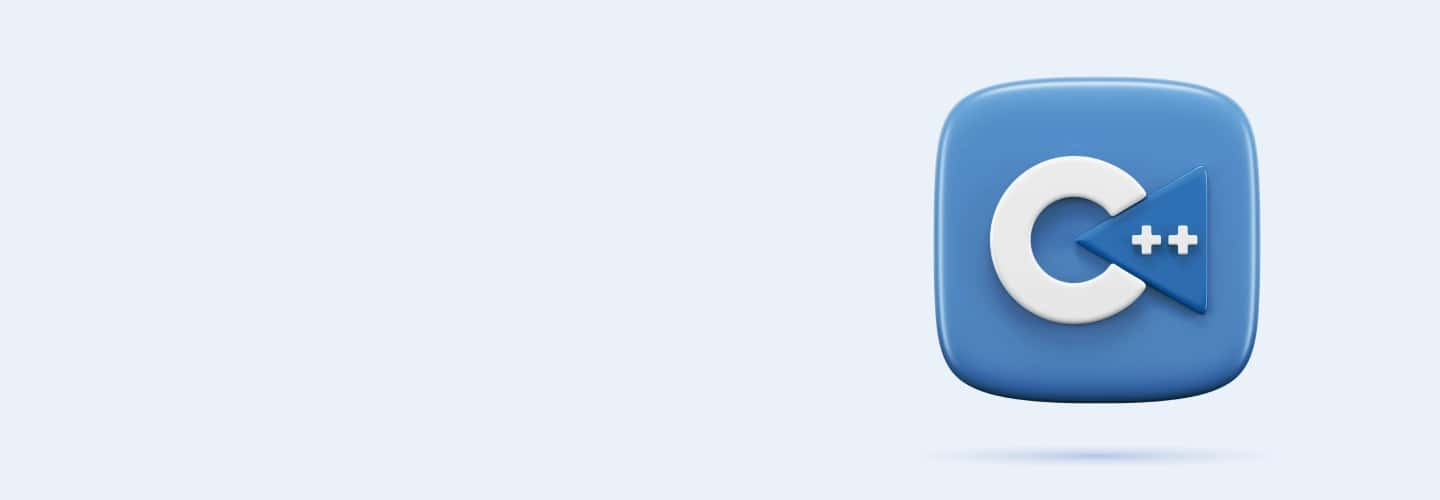
Q91
Q91 In C++, what is polymorphism?
The ability of different classes to have methods with the same name
The capability of a class to have multiple constructors
The ability of a function to perform different tasks based on the context
The concept of creating many objects from a single class
Q92
Q92 What is the purpose of a constructor in a C++ class?
To initialize the class's member variables
To delete objects of the class
To allocate memory for objects
To declare a new class
Q93
Q93 What is operator overloading in C++?
Giving additional meaning to C++ operators
Creating new operators in C++
Using existing operators in different contexts
Defining operators only in classes
Q94
Q94 How is abstraction different from encapsulation?
Abstraction hides complexity by showing only essential features, while encapsulation hides the internal state of an object
Abstraction and encapsulation are the same
Abstraction is used only in inheritance, while encapsulation is not
Encapsulation cannot be used in conjunction with polymorphism
Q95
Q95 What is the output of this C++ code?
class Test {
public:
int x;
}; Test obj;
obj.x = 5;
cout << obj.x;
5
0
Error
Undefined
Q96
Q96 Given the following C++ code, what is the output?
class MyClass { public: MyClass() {
cout << "Hello"; }
};
MyClass obj;
Hello
MyClass
Error
Nothing
Q97
Q97 In the code class Base {
};
class Derived : public Base {
};,
which OOP concept is illustrated?
Encapsulation
Polymorphism
Inheritance
Abstraction
Q98
Q98 Consider the code:
class Shape {
public:
virtual void draw() = 0;
}; class Circle : public Shape {
public: void draw() {
cout << "Circle"; }
};
What concept does this illustrate?
Encapsulation
Inheritance
Polymorphism
Abstraction
Q99
Q99 Pseudocode:
CLASS Animal FUNCTION makeSound() PRINT "Sound" What is this an example of?
A function in C++
A class in C++
Polymorphism in C++
Encapsulation in C++
Q100
Q100 Pseudocode:
CLASS Rectangle PRIVATE width, height PUBLIC FUNCTION setDimensions(w, h) SET width TO w SET height TO h END What OOP concept is demonstrated here?
Encapsulation
Inheritance
Polymorphism
Abstraction
Q101
Q101 Pseudocode: CLASS Bird EXTENDS Animal FUNCTION makeSound() PRINT "Chirp" What concept is shown here?
Inheritance
Polymorphism
Encapsulation
Abstraction
Q102
Q102 Pseudocode:
CLASS Shape ABSTRACT FUNCTION draw() END CLASS Circle EXTENDS Shape FUNCTION draw() PRINT "Circle" What does this illustrate?
Encapsulation
Abstraction
Inheritance
Polymorphism
Q103
Q103 Find the error:
class MyClass { public: MyClass(int x) {
cout << x; }
};
MyClass obj;
Missing default constructor in class definition
Syntax error
No error
Incorrect constructor usage
Q104
Q104 Identify the mistake:
class Base {
public:
virtual void func() {} };
class Derived : public Base {
void func();
};
Missing function body in Derived
Incorrect use of virtual function
No error
Syntax error in class declaration
Q105
Q105 Spot the error:
class Test {
public:
int x;
void Test() {
x = 0; }
};
Incorrect constructor syntax
No error
Syntax error in variable declaration
Member initialization error
Q106
Q106 What is the primary purpose of the fstream library in C++?
To perform mathematical operations
To manage screen output
To handle file input and output
To process user input
Q107
Q107 In C++, which function is used to open a file?
file.open()
file.read()
file.write()
file.close()
Q108
Q108 What is a file pointer in C++?
A pointer to the contents of a file
A variable that stores the memory address of a file
A marker that keeps track of the file's current read/write position
A pointer to the file's metadata
Q109
Q109 How do you move the file pointer to a specific location for reading or writing in a file in C++?
Using the move() function
Using the relocate() function
Using the seekg() and seekp() functions
Using the transfer() function
Q110
Q110 Given the C++ code
ofstream file("example.txt");
file << "Hello, World!";
file.close();,
what does this code do?
Reads "Hello, World!" from example.txt
Writes "Hello, World!" to example.txt
Deletes "Hello, World!" from example.txt
Opens example.txt without modifying it
Q111
Q111 Pseudocode:
OPEN file.txt AS file WRITE "Data" TO file CLOSE file What is the primary action performed by this code?
Reading data from file.txt
Writing data to file.txt
Deleting data from file.txt
Creating a new file named file.txt
Q112
Q112 Identify the issue in this C++ code:
ifstream file;
file.open("data.txt");
Incorrect file mode
File not closed
Missing file path
No error
Q113
Q113 Spot the error:
ofstream file("example.txt");
file >> "Text";
file.close();
Wrong operator used for writing to file
File is not opened correctly
File is not closed properly
No error
Q114
Q114 What is the purpose of the new operator in C++?
To create a new class
To allocate memory on the stack
To allocate memory on the heap
To define a new data type
Q115
Q115 What does the delete operator do in C++?
Deletes a variable from the program
Frees memory allocated by new
Removes a file from the disk
Erases a class definition
Q116
Q116 Which of the following statements about dynamic memory allocation is true?
Memory allocated by new is automatically deallocated
new and delete can only be used with primitive data types
new returns null if memory allocation fails
Dynamic memory allocation is less efficient than static allocation
Q117
Q117 What is memory leak in the context of dynamic memory allocation?
An error that occurs when memory allocation fails
The process of deleting unused memory
Memory that is not properly released after use
A special technique to clean memory
Q118
Q118 How can you prevent a memory leak in a C++ program?
By using automatic variables instead of dynamic variables
By ensuring every new operator is matched with delete
By avoiding the use of pointers
By only using static memory allocation
Q119
Q119 Given the C++ code:
int *ptr = new int(5);
*ptr = 10;
delete ptr;,
what does this code do?
Allocates memory for an integer, sets it to 10, then releases the memory
Causes a memory leak
Generates a runtime error
Allocates memory for an integer without releasing it
Q120
Q120 Identify the issue in this code:
int *arr = new int[5];
delete arr;
Incorrect syntax for deleting an array
No error
Memory leak
Access violation