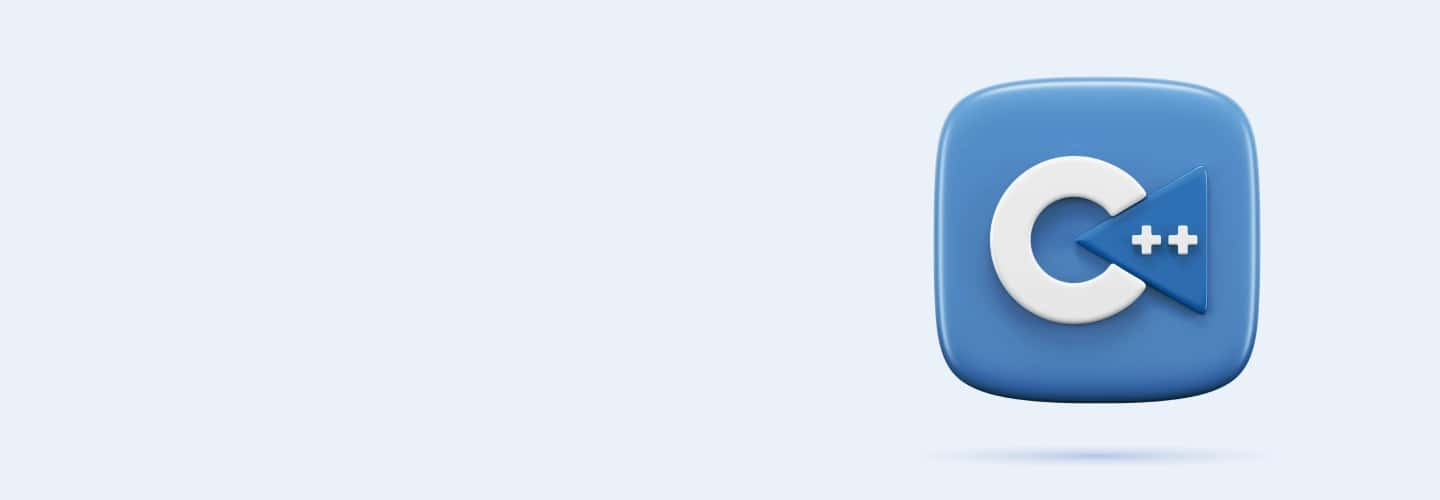
Q61
Q61 How do you access the third element in an array named 'arr' in C++?
arr[2]
arr[3]
arr(2)
arr(3)
Q62
Q62 What are multidimensional arrays in C++?
Arrays with only one row or column
Arrays with more than one dimension, like 2D or 3D arrays
Arrays containing elements of different data types
Dynamic arrays
Q63
Q63 In C++, how can you initialize an array of integers named 'num' with the first four natural numbers?
int num[] = {1, 2, 3, 4};
int num[4] = {1, 2, 3, 4};
int num[4] = {1, 2, 3};
int num[] = {1, 2, 3, 4, 5};
Q64
Q64 What is the size of a character array initialized as char arr[] = "Hello";?
4
5
6
7
Q65
Q65 Which statement is true about passing an array to a function in C++?
The array is always passed by value
The array is always passed by reference
A copy of the array is created
The size of the array must be known to the function
Q66
Q66 What is the output of this code?
int arr[] = {1, 2, 3};
cout << arr[1];
1
2
3
Error
Q67
Q67 Consider the code:
int matrix[2][3] = {{1, 2, 3}, {4, 5, 6}};
cout << matrix[1][1];
What does it print?
1
2
5
6
Q68
Q68 What is the output of the following code?
char chars[] = {'A', 'B', 'C', '\0'};
cout << chars;
A
AB
ABC
ABCD
Q69
Q69 Pseudocode:
SET arr[3] TO [10, 20, 30] PRINT arr[0] What does this print?
10
20
30
0
Q70
Q70 Pseudocode:
SET matrix[2][2] TO [[1, 2], [3, 4]] PRINT matrix[0][1] What is the output?
1
2
3
4
Q71
Q71 Pseudocode:
SET nums[5] TO [1, 2, 3, 4, 5] FOR EACH num IN nums DO PRINT num What does this print?
12345
54321
15
Error
Q72
Q72 Pseudocode:
SET charArray TO ['H', 'e', 'l', 'l', 'o', '\0'] PRINT charArray What is printed?
H
Hello
ello
Helloworld
Q73
Q73 Pseudocode:
SET arr[3] TO [0] FOR i FROM 0 TO 2 DO SET arr[i] TO i + 1 END PRINT arr[2] What is the output?
1
2
3
0
Q74
Q74 Identify the error in this code:
int arr[3];
arr[3] = 10;
cout << arr[3];
Out of bounds array access
Syntax error
Type mismatch
No error
Q75
Q75 Spot the mistake:
int nums[] = {1, 2, 3};
for(int i = 0; i <= 3; i++) {
cout << nums[i];
}
Incorrect loop condition
Missing array initialization
No error
Syntax error in cout statement
Q76
Q76 Find the error in this code:
char str[5]; str = "Hello";
cout << str;
Invalid assignment to char array
No error
Syntax error in cout statement
Array size mismatch
Q77
Q77 What is a pointer in C++?
A variable that stores the address of another variable
A reference to another variable
A type of array
A function parameter
Q78
Q78 How do you declare a pointer to an integer in C++?
int *ptr;
int &ptr;
int ptr[];
int ptr;
Q79
Q79 What is the purpose of the reference operator (&) in C++?
To create a reference variable
To access the address of a variable
To dereference a pointer
To perform bitwise AND
Q80
Q80 What does the dereference operator (*) do in C++?
It multiplies two values
It returns the address of a pointer
It accesses the value at the address a pointer is pointing to
It creates a pointer
Q81
Q81 How are arrays and pointers related in C++?
Pointers can only point to the first element of an array
Arrays and pointers are fundamentally different
An array name can be used as a pointer to its first element
Pointers cannot be used to access array elements
Q82
Q82 What is the output of the following code?
int x = 10;
int *ptr = &x;
cout << *ptr;
10
&x
ptr
*ptr
Q83
Q83 Given the code
int arr[] = {1, 2, 3};
int *ptr = arr;
cout << ptr[1];,
what is the output?
1
2
3
&arr[1]
Q84
Q84 Pseudocode:
SET x TO 20 SET ptr TO ADDRESS OF x PRINT VALUE AT ptr What does this print?
20
ADDRESS OF x
ptr
VALUE AT ptr
Q85
Q85 Pseudocode:
SET arr TO [10, 20, 30] SET ptr TO ADDRESS OF arr[0] PRINT ptr[2] What is the output?
10
20
30
ADDRESS OF arr[2]
Q86
Q86 Identify the error in this code:
int x = 5;
int *ptr;
*ptr = &x;
cout << *ptr;
Incorrect assignment to pointer
Syntax error
No error
Dereferencing an uninitialized pointer
Q87
Q87 Find the mistake:
int num = 10;
int &ref = num;
ref = nullptr;
cout << num;
Assigning nullptr to a reference
Syntax error
No error
Incorrect initialization of the reference
Q88
Q88 What is a class in C++?
A blueprint for creating objects
A function in OOP
A variable type
An operator
Q89
Q89 What is inheritance in OOP?
The process of acquiring properties from one class to another
Copying of methods between classes
Linking of two classes
Creating new classes
Q90
Q90 What does encapsulation in C++ refer to?
The process of combining data and functions into a single unit
Creating multiple copies of the same function
Protecting data by making it private
Linking different classes together