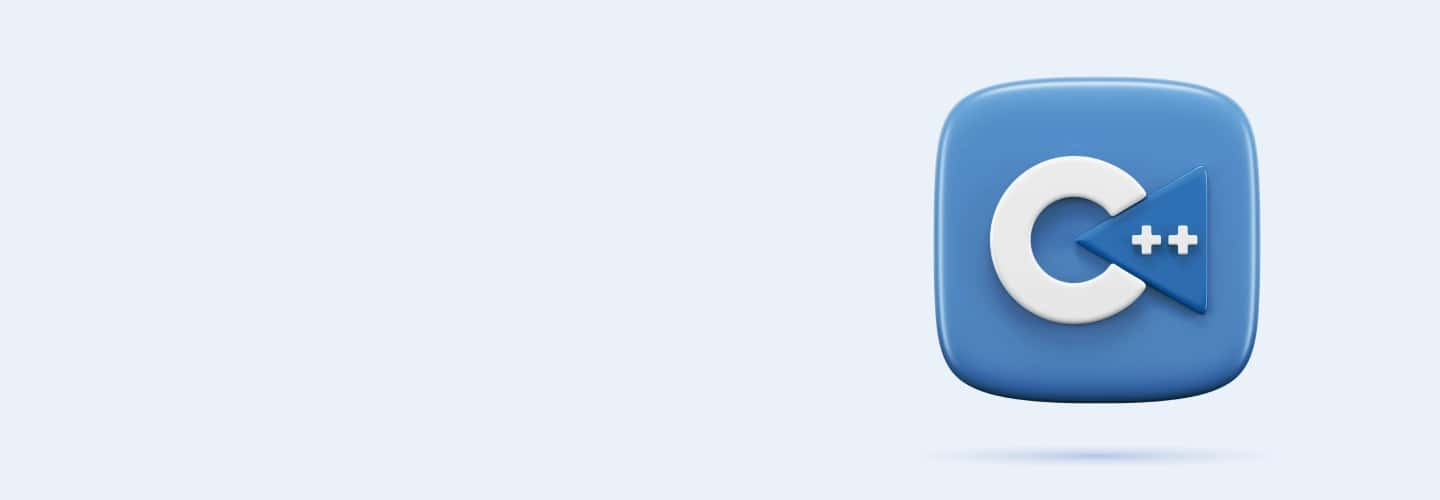
Q31
Q31 What is the purpose of the if statement in C++?
To execute a block of code multiple times
To stop the execution of the program
To make a decision
To declare a variable
Q32
Q32 Which control flow statement is used for executing a block of code repeatedly based on a condition?
if
else
for
switch
Q33
Q33 What is the function of the break statement in a loop?
To pause the loop
To skip an iteration of the loop
To terminate the loop
To continue to the next iteration of the loop
Q34
Q34 In C++, the do-while loop is unique because it...
executes its block of code at least once
is faster than other loops
does not check a condition
can only run a fixed number of times
Q35
Q35 What is the primary difference between a for loop and a while loop in C++?
Syntax only
While loop can run infinitely, for loop cannot
For loop is used for arrays, while loop is not
Initialization, condition checking, and increment/decrement are part of the for loop statement
Q36
Q36 What happens if the condition in an if statement is false?
The program terminates
The else part is executed
The program skips to the next loop
None of the above
Q37
Q37 What is the output of this code?
int i = 0;
while(i < 3) {
cout << i; i++;
}
012
123
Infinite loop
Syntax error
Q38
Q38 Consider the code:
for(int i = 0; i <= 2; i++) {
cout << i;
}.
What does it print?
012
123
Nothing
Error
Q39
Q39 What is the output of the following code?
int x = 10;
do {
cout << x;
x--; } while (x > 10);
Prints 10 once
Prints 10 and then 9
Prints numbers from 10 to 1
No output
Q40
Q40 Pseudocode:
IF x > 10 THEN PRINT "Greater" ELSE PRINT "Smaller or Equal" If x is 8, what is printed?
Greater
Smaller or Equal
Error
Nothing
Q41
Q41 Pseudocode:
SET i TO 0 WHILE i < 3 DO PRINT i INCREMENT i What does this print?
012
123
Infinite loop
Nothing
Q42
Q42 Pseudocode:
FOR i FROM 0 TO 2 DO PRINT i What is the output?
012
123
Error
Nothing
Q43
Q43 Pseudocode:
SET count TO 0 REPEAT PRINT count INCREMENT count UNTIL count EQUALS 5 What is the output?
01234
012345
Infinite loop
Error
Q44
Q44 Identify the error in the code:
int i = 1;
for(; i <= 5; i++) {
cout << i;
}
Missing initialization in for loop
No error
Syntax error in cout statement
Incorrect loop condition
Q45
Q45 Spot the mistake:
int num = 0;
do {
cout << num;
} while(num);
Infinite loop
Syntax error
Should use a for loop
No error
Q46
Q46 What is a function prototype in C++?
A function that always returns an integer
The first call to a function in a program
A declaration of a function before its definition
A function without arguments
Q47
Q47 What is function overloading in C++?
Calling a function multiple times
Defining multiple functions with the same name but different parameters
Defining a function inside another function
Using default arguments in a function
Q48
Q48 What is the purpose of default arguments in function definitions?
To specify what value a function returns
To give a default value to a parameter if no argument is passed
To make a parameter optional
To overload a function
Q49
Q49 In C++, what is recursion?
A function calling another function
A function that calls itself
Repeatedly executing a loop
A type of data structure
Q50
Q50 How does a recursive function in C++ stop calling itself?
By using a for loop
By reaching a return statement
Through a conditional statement that leads to a return
By calling another function
Q51
Q51 What does this function do?
void printHello() {
cout << "Hello, World!";
}
Prints "Hello, World!" to the console repeatedly
Prints "Hello, World!" once to the console
Does nothing
Causes a runtime error
Q52
Q52 What is the output of this function call?
int add(int x, int y = 5) {
return x + y;
} cout << add(3);
8
3
5
Syntax error
Q53
Q53 Consider the function:
int multiply(int x, int y) { return x * y; }
What is returned by multiply(5, 4);?
20
9
Syntax error
0
Q54
Q54 Pseudocode:
FUNCTION AddNumbers(x, y) RETURN x + y END What does this function do?
Adds two numbers and prints the result
Subtracts two numbers
Adds two numbers and returns the result
Multiplies two numbers
Q55
Q55 Pseudocode:
FUNCTION PrintMessage() PRINT "Hello" END What is the purpose of this function?
To return the string "Hello"
To print "Hello" to the console
To store the message "Hello"
To check if "Hello" is printed
Q56
Q56 Pseudocode:
FUNCTION FindMax(x, y) IF x > y THEN RETURN x ELSE RETURN y END What does this function return?
The sum of x and y
The smaller of x and y
The larger of x and y
Nothing if x equals y
Q57
Q57 Pseudocode:
FUNCTION CalculateFactorial(n) IF n <= 1 THEN RETURN 1 ELSE RETURN n * CalculateFactorial(n - 1) END What does this function calculate?
The sum of numbers up to n
The factorial of n
The product of n and n-1
The nth number in the Fibonacci sequence
Q58
Q58 Find the error in this function:
int square(int n) {
return n * n;
}
cout << square();
Missing argument in function call
Syntax error in return statement
No return type for function
No error
Q59
Q59 Identify the mistake in this code:
void printNum(int number = 5) {
cout << number;
}
printNum(10, 5);
Incorrect number of arguments
No error
Syntax error in function definition
Error in default argument
Q60
Q60 What is an array in C++?
A collection of variables of different types
A single variable that can store multiple values
A function that returns multiple values
A data structure that allows recursion