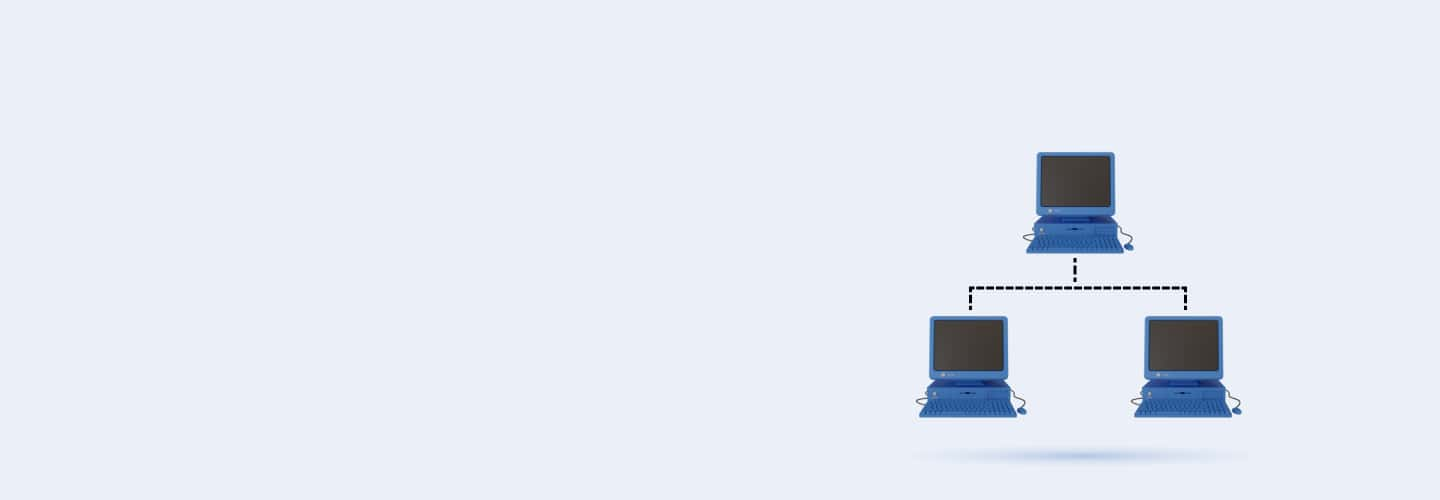
Q61
Q61 How does memory-mapped I/O differ from isolated I/O?
Memory-mapped I/O shares address space with memory
Memory-mapped I/O uses separate address spaces
Both use interrupts
Isolated I/O uses separate address spaces
Q62
Q62 Write an assembly language instruction to read data from an I/O port using IN instruction.
MOV AX, 30H
XOR AX, AX
OUT AX, 30H
IN AX, 30H
Q63
Q63 How would you implement an interrupt handler in assembly language?
By writing a subroutine
By sending data to the device
By polling the device
By using a jump instruction
Q64
Q64 Write an assembly language command to transfer data to a peripheral device using the OUT instruction.
OUT 30H, AX
IN AX, 30H
MOV AX, 30H
AND AX, AX
Q65
Q65 A device sends data in 16-bit format. How would you configure a CPU register to handle this data in assembly?
Set the AL register
Set the AX register
Set the AH register
Set the BX register
Q66
Q66 The CPU is unable to detect when an I/O device is ready for data transfer. What could be the issue?
ALU failure
Faulty interrupt system
DMA failure
Incorrect polling
Q67
Q67 A program is not correctly sending data to an I/O device. What could be the issue?
ALU failure
Cache memory error
Incorrect OUT instruction
Faulty Program Counter
Q68
Q68 The CPU is experiencing slow data transfer with an I/O device. What could be a potential cause?
Improper polling
DMA malfunction
Faulty ALU
Interrupt-driven I/O failure
Q69
Q69 What is the primary benefit of pipelining in CPU architecture?
By reducing instruction length
By storing more data
Increases CPU clock speed
Allows multiple instructions to be executed simultaneously
Q70
Q70 Which of the following types of hazards occurs when two instructions need the same data simultaneously?
Structural hazard
Memory hazard
Control hazard
Data hazard
Q71
Q71 How do control hazards affect pipelining performance?
They reduce cache hit rates
They reduce instruction throughput
They increase memory access time
They increase instruction length
Q72
Q72 What is instruction-level parallelism (ILP)?
Executing instructions in sequence
Executing multiple instructions from the same thread
Executing instructions sequentially
Executing multiple instructions from different threads
Q73
Q73 Which technique can help mitigate the impact of branch hazards in pipelining?
Reducing instruction size
Branch prediction
Increasing clock speed
Increasing pipeline stages
Q74
Q74 How would you handle a data hazard in a pipelined processor?
Increase clock speed
Reorder instructions to avoid conflicts
Use more cache memory
Reduce pipeline stages
Q75
Q75 How would you implement instruction pipelining in an assembly language program?
By using more memory
By executing instructions sequentially
By overlapping the execution of multiple instructions
By increasing clock speed
Q76
Q76 How can data hazards be avoided in a pipelined processor?
By using direct memory access
By reordering instructions
By increasing memory size
By using sequential execution
Q77
Q77 A pipelined CPU is experiencing frequent stalls. What is the most likely cause?
Control hazard
Memory overflow
ALU failure
Data hazard
Q78
Q78 A CPU pipeline is stalling on every branch instruction. What technique could solve this issue?
Increasing cache size
Sequential execution
Branch prediction
Memory mapping
Q79
Q79 A processor with instruction pipelining is experiencing performance issues during parallel execution. Why?
ALU failure
Incorrect instruction set
Insufficient registers
Poor pipeline design
Q80
Q80 What is the main function of cache memory in a computer system?
To execute instructions
To store unused data
To store frequently used data
To increase CPU speed
Q81
Q81 How does cache memory improve CPU performance?
By storing instructions
By increasing clock speed
By reducing memory access time
By storing programs
Q82
Q82 Which type of cache memory is typically located closest to the CPU?
L1 cache
L2 cache
L3 cache
Main memory
Q83
Q83 What happens when a cache miss occurs?
Data is retrieved from the cache
CPU executes the instruction again
Data is fetched from the main memory
Data is lost
Q84
Q84 How does set-associative mapping differ from direct mapping in cache memory?
Set-associative mapping uses fewer blocks
Direct mapping is slower
Set-associative mapping uses more blocks per set
Direct mapping uses random blocks
Q85
Q85 Write an assembly instruction to load data from cache into a register.
MOV AX, [CACHE]
MOV AX, [MEM]
LOAD AX, [MEM]
LOAD AX, [CACHE]
Q86
Q86 A CPU accesses cache memory with the address 1010. What is the corresponding set index for 4-set cache?
2
0
3
1
Q87
Q87 Given a cache size of 32 KB with 8 bytes per block, how many blocks are available in the cache?
512
256
1024
4096
Q88
Q88 A CPU is experiencing frequent cache misses. What could be the reason?
ALU failure
Program counter issue
Incorrect clock speed
Too small cache size
Q89
Q89 A cache is underperforming, leading to frequent memory accesses. What could be the issue?
Too many registers
Poor cache replacement policy
ALU malfunction
Overloaded CPU
Q90
Q90 A system with a well-sized cache is experiencing unexpected slowdowns. What could be the problem?
Too many branches
Cache memory failure
Incorrect cache mapping
Insufficient RAM