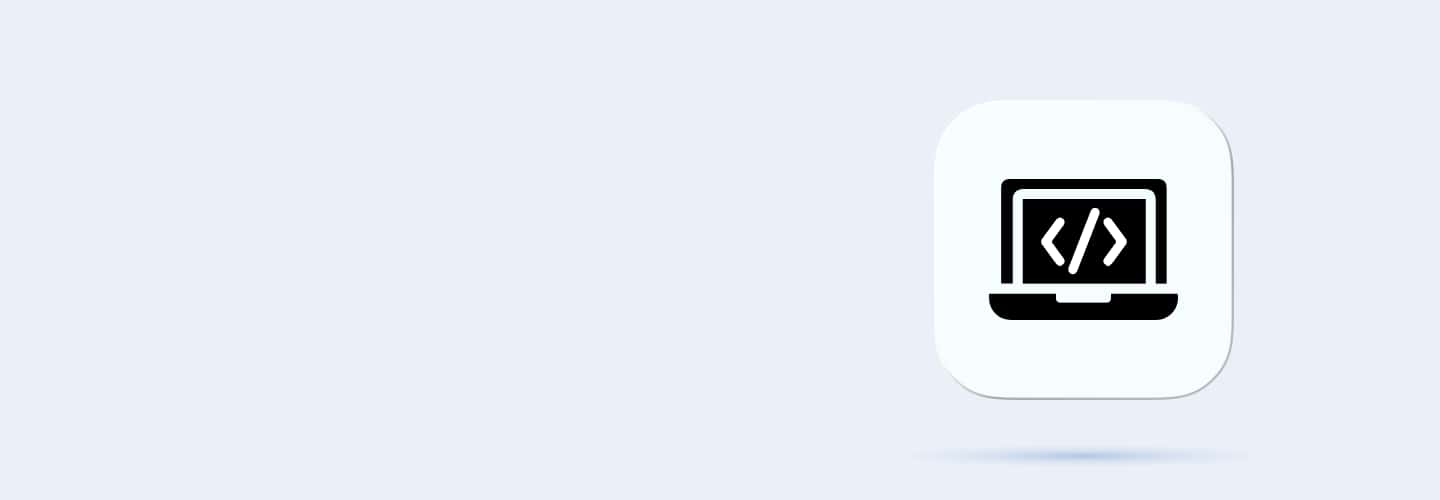
Q91
Q91 What is the likely cause of inefficient machine code generation?
Suboptimal instruction selection
Errors in parsing
Type mismatches
Incorrect syntax trees
Q92
Q92 What is the primary purpose of a symbol table in a compiler?
To store syntax rules
To store identifiers and their attributes
To generate intermediate code
To check for logical errors
Q93
Q93 Which data structure is commonly used to implement a symbol table?
Array
Stack
Hash table
Linked list
Q94
Q94 What information is typically stored in a symbol table for variables?
Only variable names
Names and values
Names, types, and scope
Syntax and rules
Q95
Q95 What role does the runtime stack play in a runtime environment?
Stores variables
Manages function calls
Holds intermediate code
Optimizes machine code
Q96
Q96 Why is dynamic memory allocation handled by the runtime environment?
To reduce compile-time errors
To improve code readability
To handle memory requirements at runtime
To optimize machine code
Q97
Q97 How is a new variable added to the symbol table during compilation?
It is added by the lexical analyzer
It is added by the semantic analyzer
It is added by the syntax analyzer
It is added by the runtime environment
Q98
Q98 What entry would a function declaration add to a symbol table?
Function name only
Function name and parameters
Function name, parameters, and return type
None of the above
Q99
Q99 How are nested scopes managed in a symbol table?
Using a single global table
Using a stack of symbol tables
Using separate files
Using intermediate code
Q100
Q100 What error occurs if a variable is not found in the symbol table during compilation?
Syntax error
Logical error
Undefined variable error
Runtime error
Q101
Q101 What is the likely issue if a function's return type conflicts with its usage?
Semantic error
Runtime error
Syntax error
Memory allocation error
Q102
Q102 How does a runtime environment handle dangling pointers?
By automatically freeing memory
By detecting and nullifying the pointer
By converting pointers to references
By ignoring them
Q103
Q103 What is the primary purpose of error detection in a compiler?
To ensure code optimization
To validate code correctness
To parse syntax trees
To generate machine code
Q104
Q104 Which phase of the compiler is responsible for detecting syntax errors?
Lexical Analysis
Syntax Analysis
Semantic Analysis
Code Generation
Q105
Q105 What is a common method for recovering from errors in a compiler?
Backtracking
Error messages
Panic mode
Intermediate code generation
Q106
Q106 Which type of errors are typically detected during semantic analysis?
Syntax errors
Logical errors
Type mismatch errors
Memory errors
Q107
Q107 What is the difference between lexical and syntax errors?
Lexical errors occur at runtime, syntax errors at compile time
Lexical errors involve tokens, syntax errors involve structure
Syntax errors involve semantics, lexical errors involve parsing
There is no difference
Q108
Q108 What is the error in the following code: if (x > y { z = x; }
Lexical error
Syntax error
Semantic error
Runtime error
Q109
Q109 Identify the error in this statement: int x = 5; float y = x + 'a';
Lexical error
Syntax error
Type mismatch
Logical error
Q110
Q110 What type of recovery is used if a parser corrects the code to continue?
Panic mode recovery
Phrase-level recovery
Error production recovery
Automatic recovery
Q111
Q111 What error will occur if a variable is used without initialization?
Syntax error
Semantic error
Logical error
Runtime error
Q112
Q112 What is the likely issue if a compiler reports 'missing semicolon' at an incorrect location?
Lexical error
Syntax error
Semantic error
Error propagation
Q113
Q113 What is the primary purpose of a compiler toolchain?
To optimize machine code
To link libraries
To convert source code into executable programs
To debug runtime errors
Q114
Q114 Which of the following is an open-source compiler infrastructure?
GCC
LLVM
Clang
All of the above
Q115
Q115 What is the role of an assembler in a compiler toolchain?
To optimize code
To convert assembly code into machine code
To link libraries
To generate intermediate code
Q116
Q116 Which component of the compiler toolchain is responsible for resolving external references in a program?
Compiler
Linker
Loader
Assembler
Q117
Q117 What output is generated by a lexical analyzer generator such as Lex?
Intermediate code
Token stream
Machine code
Abstract syntax tree
Q118
Q118 How does Yacc assist in compiler design?
It generates lexical analyzers
It generates parsers
It generates intermediate code
It generates machine code
Q119
Q119 What is the most likely issue if a linker reports 'undefined reference' errors?
Syntax error
Missing library
Runtime error
Semantic error
Q120
Q120 How can a debugging tool like GDB assist in compiler design?
By optimizing machine code
By identifying runtime errors
By parsing grammar rules
By resolving lexical errors