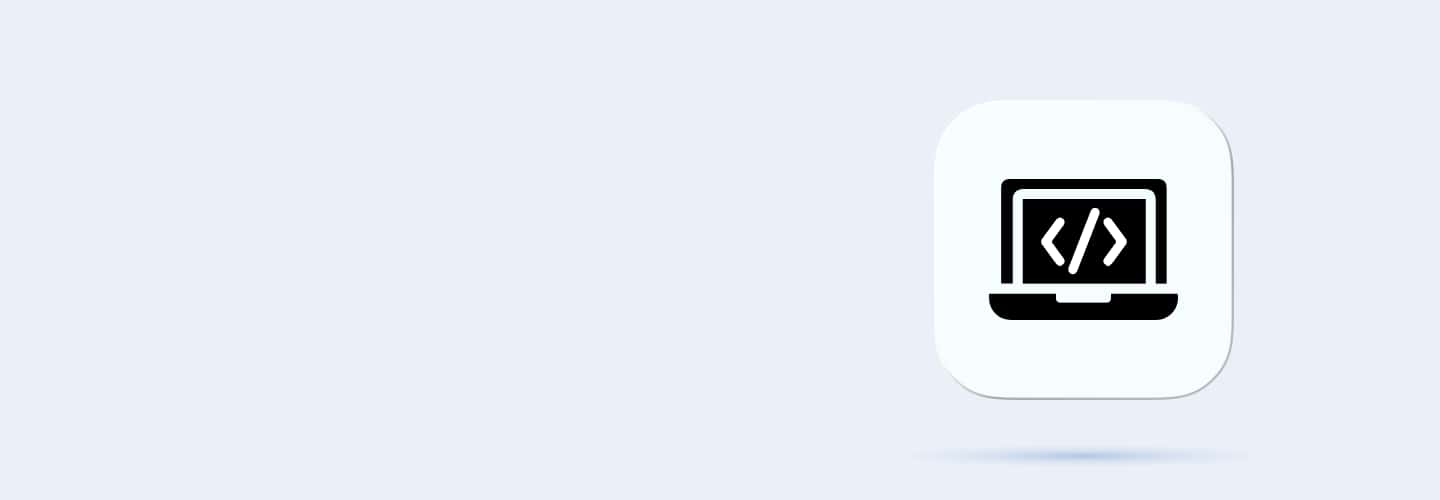
Q61
Q61 Why is intermediate code generation important in a compiler?
To simplify parsing
To optimize syntax trees
To achieve platform independence
To directly execute programs
Q62
Q62 What is the challenge of converting an abstract syntax tree into intermediate code?
Handling syntax errors
Resolving scope rules
Preserving the order of operations
Detecting type mismatches
Q63
Q63 Which intermediate representation would result from the expression 'a + b * c'?
a b c * +
a + b c *
b c + a *
a * b + c
Q64
Q64 Given the quadruple representation for x = a + b * c, what would be the last instruction?
x = a + b * c
t1 = b * c
t2 = a + t1
x = t2
Q65
Q65 What is the three-address code for the statement: z = (x + y) * w?
t1 = x + y; z = t1 * w
t1 = x + y; t2 = t1 * w; z = t2
z = (x + y) * w
t1 = x * w + y
Q66
Q66 What is the postfix representation of the expression: (a + b) * (c - d)?
a b + c d - *
a b c d + - *
a + b c - d *
a b c d * + -
Q67
Q67 What error is detected if a variable is used in intermediate code without initialization?
Syntax error
Semantic error
Initialization error
Lexical error
Q68
Q68 What might cause an 'undefined temporary variable' error during intermediate code generation?
A missing temporary variable declaration
Incorrect operator precedence
Improper grammar definition
Invalid target machine code
Q69
Q69 How can intermediate code generation handle expressions with multiple operators?
By using operator precedence rules
By creating new grammars
By generating random code
By skipping intermediate steps
Q70
Q70 What is the main goal of code optimization in a compiler?
To reduce code size
To improve execution speed
To ensure correctness
Both reduce code size and improve execution speed
Q71
Q71 Which of the following is a local optimization technique?
Dead code elimination
Loop unrolling
Function inlining
Common subexpression elimination
Q72
Q72 Which type of optimization requires control flow analysis?
Global optimization
Local optimization
Peephole optimization
Intermediate code generation
Q73
Q73 What is loop unrolling in the context of code optimization?
Reducing loop iterations
Combining multiple loops
Rewriting loops to execute fewer iterations
Removing loops altogether
Q74
Q74 Which optimization technique focuses on eliminating redundant code computations?
Constant folding
Loop unrolling
Common subexpression elimination
Dead code elimination
Q75
Q75 What is the optimized form of the expression: a = b + c; d = b + c;?
a = b + c; d = a;
a = b + c; d = b + c;
a = d = b + c;
No optimization possible
Q76
Q76 Which loop optimization is applied to: for(i=0; i<10; i++) sum += i;?
Constant folding
Loop unrolling
Loop fusion
Dead code elimination
Q77
Q77 What is the result of applying constant folding to the code: int x = 5 * 2;?
int x = 5 * 2;
int x = 10;
No change
Compilation error
Q78
Q78 What is the possible issue if loop unrolling results in increased code size?
Poor register allocation
Memory allocation failure
Code bloat
Unoptimized machine code
Q79
Q79 What type of error occurs if dead code is incorrectly eliminated?
Semantic error
Logical error
Runtime error
Syntax error
Q80
Q80 How can global optimization cause unintended side effects?
By modifying local variables
By ignoring variable scopes
By reordering instructions
By skipping error checks
Q81
Q81 What is the primary goal of code generation in a compiler?
To translate intermediate code to machine code
To optimize syntax trees
To validate program logic
To check for runtime errors
Q82
Q82 Which of the following is a challenge in code generation?
Parsing the input
Optimizing intermediate code
Efficient register allocation
Ensuring lexical correctness
Q83
Q83 What is instruction selection in the context of code generation?
Choosing instructions based on syntax
Choosing efficient machine instructions
Selecting intermediate code
Selecting error-handling code
Q84
Q84 Which of the following issues can occur during register allocation?
Code redundancy
Register spill
Incorrect parsing
Invalid syntax
Q85
Q85 Why is target machine architecture important in code generation?
To optimize source code
To ensure platform independence
To map intermediate code to machine code
To validate grammar rules
Q86
Q86 What is the generated machine code for the intermediate instruction: t1 = a + b?
ADD a, b, t1
ADD t1, a, b
LOAD t1, a
STORE t1, b
Q87
Q87 How is the instruction 'x = y + z' translated to machine code using two-address instructions?
ADD y, z, x
LOAD x, y; ADD x, z
LOAD y; ADD y, z; STORE x
None of the above
Q88
Q88 What is the output of translating the expression (a + b) * c into three-address machine code?
t1 = a + b; t2 = t1 * c
t1 = a * c; t2 = t1 + b
t1 = a + c; t2 = t1 * b
t1 = a * b; t2 = t1 + c
Q89
Q89 What is the most likely cause of 'unmapped variable' error during code generation?
Undefined variable in source code
Syntax error in intermediate code
Unallocated register for a variable
Machine code overflow
Q90
Q90 Why might a 'segmentation fault' occur in generated machine code?
Incorrect syntax in source code
Accessing invalid memory locations
Loop unrolling
Register spill