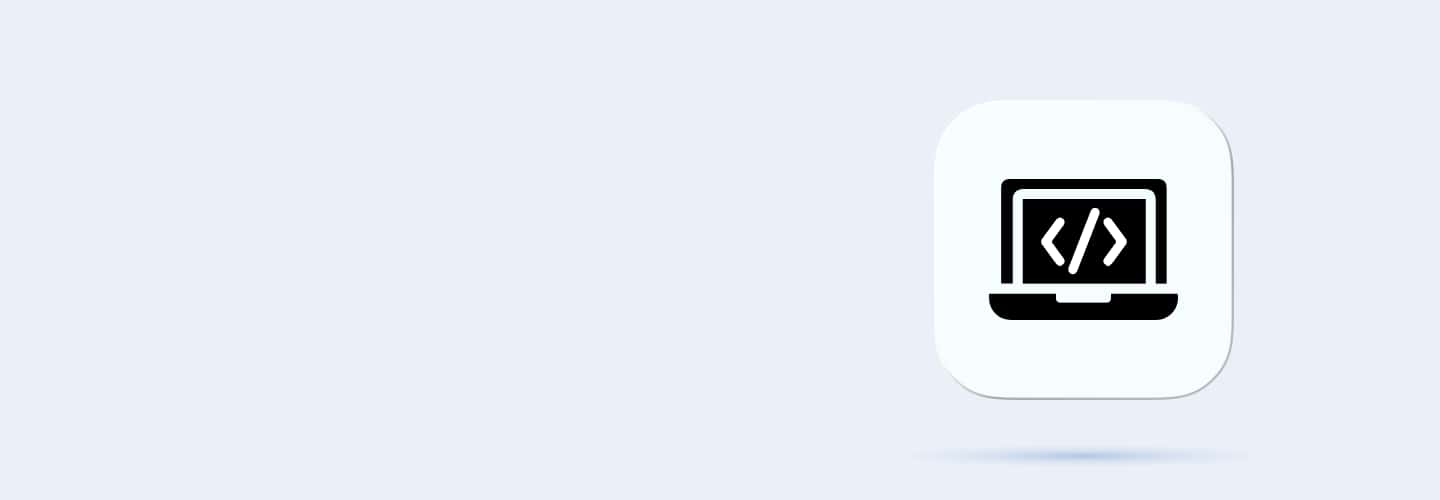
Q31
Q31 During tokenization, the lexical analyzer identifies an identifier starting with a digit. What type of error is this?
Logical error
Syntax error
Lexical error
Type error
Q32
Q32 What is the main role of syntax analysis in a compiler?
To check lexical rules
To validate grammatical structure
To optimize code
To generate machine code
Q33
Q33 Which data structure is used in top-down parsing to manage recursive calls?
Queue
Stack
Linked List
Binary Tree
Q34
Q34 What does an LR parser stand for in parsing techniques?
Left-to-right, rightmost derivation
Leftmost derivation, recursive
Right-to-left, leftmost derivation
Left-to-right, leftmost derivation
Q35
Q35 What is the primary difference between LL and LR parsers?
LL parsers use top-down parsing, LR parsers use bottom-up parsing
LL parsers handle left recursion, LR parsers cannot
LR parsers use recursive descent, LL parsers use shift-reduce
Both are identical
Q36
Q36 Which of the following is NOT a type of parsing algorithm?
Top-down parsing
Bottom-up parsing
Lexical parsing
Predictive parsing
Q37
Q37 Why is lookahead used in parsing?
To optimize parsing
To resolve ambiguities
To simplify grammar rules
To identify lexical errors
Q38
Q38 What is the FIRST step in constructing a parse tree?
Start with the root symbol
Expand all terminal nodes
Collapse all non-terminals
Evaluate leaf nodes
Q39
Q39 Given the grammar S → AA, A → aA | b, what is the language generated by the grammar?
All strings of 'a's followed by a single 'b'
All strings of 'a's and 'b's
All strings with even length
All strings with no 'a's or 'b's
Q40
Q40 What is the output of applying shift-reduce parsing to 'id + id' using the grammar E → E + E
A parse tree
A syntax error
Shift-reduce conflict
A leftmost derivation
Q41
Q41 Using recursive descent parsing, which grammar rule can cause an infinite loop?
Right-recursive grammar
Ambiguous grammar
Left-recursive grammar
Non-recursive grammar
Q42
Q42 A parser encounters an unexpected token '}' in the input. What type of error is this?
Lexical error
Syntax error
Semantic error
Logical error
Q43
Q43 How can shift-reduce conflicts in parsing be resolved?
By simplifying grammar
By using a larger lookahead
By using recursive parsing
By removing recursion
Q44
Q44 What is the primary challenge in implementing an LR parser for ambiguous grammars?
Shift-reduce conflicts
Excessive memory usage
Incorrect syntax trees
Infinite recursion
Q45
Q45 What is the main purpose of semantic analysis in a compiler?
To optimize the code
To check logical correctness
To generate machine code
To parse input
Q46
Q46 Which error is typically detected during semantic analysis?
Syntax error
Type mismatch
Undefined symbol
Memory overflow
Q47
Q47 What is the role of a symbol table in semantic analysis?
To store lexical tokens
To store grammar rules
To store information about program identifiers
To store intermediate code
Q48
Q48 How does semantic analysis handle function overloading?
By ignoring overloaded functions
By checking parameter types and counts
By generating syntax trees
By creating machine code
Q49
Q49 Which of the following is NOT a task of semantic analysis?
Type checking
Scope resolution
Error recovery
Code generation
Q50
Q50 Why is semantic analysis essential for detecting dynamic type errors?
To ensure optimized code
To ensure runtime safety
To ensure static correctness
To parse ambiguous grammar
Q51
Q51 Which type of error will occur if a variable is used before declaration?
Syntax error
Logical error
Semantic error
Lexical error
Q52
Q52 What will semantic analysis identify in the expression: int x = 5.5;?
Syntax error
Type mismatch error
Logical error
Lexical error
Q53
Q53 Which semantic rule ensures that variables are declared before use in a block?
Scope checking
Type checking
Binding rules
Declaration rules
Q54
Q54 What error will semantic analysis detect in the function call foo(5, 'a', true) if foo is defined as foo(int, char)?
Type mismatch
Logical error
Scope error
Syntax error
Q55
Q55 What is the likely error if a compiler reports 'undefined symbol' during semantic analysis?
Variable used before declaration
Invalid syntax
Memory allocation failure
Runtime error
Q56
Q56 What is the most probable cause of a 'duplicate declaration' error during semantic analysis?
Redeclaring a variable in the same scope
Using an undeclared variable
Assigning wrong types
Syntax mismatch
Q57
Q57 How does semantic analysis handle conflicting types in a single expression?
By ignoring conflicts
By type casting automatically
By reporting a type mismatch
By optimizing the expression
Q58
Q58 What is the primary purpose of intermediate code generation in a compiler?
To generate machine code
To check for logical errors
To create an intermediate representation
To parse the input
Q59
Q59 Which form of intermediate code uses a stack for its representation?
Three-address code
Postfix notation
Quadruples
Direct machine code
Q60
Q60 Which of the following is a commonly used intermediate representation?
Syntax tree
Abstract syntax tree (AST)
Control flow graph
Three-address code