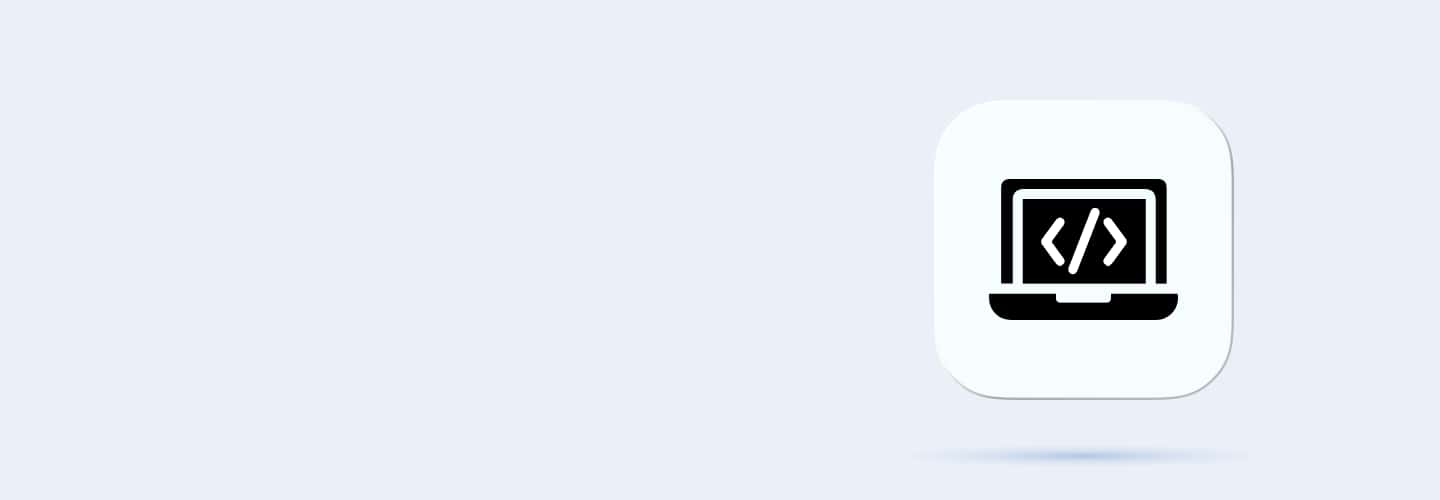
Q1
Q1 What is the main purpose of a compiler in programming?
To execute programs directly
To optimize memory
To translate source code to machine code
To interpret code line by line
Q2
Q2 Which programming phase is not a part of compiler design?
Lexical Analysis
Syntax Analysis
Intermediate Code Generation
Program Execution
Q3
Q3 What type of code does the compiler generate as output?
High-level code
Machine code
Binary code
Intermediate code
Q4
Q4 What is the difference between a compiler and an interpreter?
Compiler translates all at once, interpreter line by line
Interpreter translates all at once, compiler line by line
Both work line by line
Both translate all at once
Q5
Q5 Which of the following is NOT a phase of a compiler?
Lexical Analysis
Error Reporting
Semantic Analysis
Intermediate Code Generation
Q6
Q6 Why is it essential for a compiler to perform semantic analysis?
To check syntax rules
To translate code
To verify logical consistency
To detect runtime errors
Q7
Q7 What is the role of the front-end of a compiler?
Optimizes machine code
Manages runtime memory
Analyzes and validates source code
Executes compiled code
Q8
Q8 Which phase converts tokens into a syntax tree?
Lexical Analysis
Syntax Analysis
Code Generation
Semantic Analysis
Q9
Q9 A program fails with a 'missing semicolon' error during compilation. Which phase detects this?
Lexical Analysis
Syntax Analysis
Semantic Analysis
Code Optimization
Q10
Q10 Which phase of the compiler scans the source code as a stream of characters?
Lexical Analysis
Syntax Analysis
Semantic Analysis
Intermediate Code Generation
Q11
Q11 What is the output of the Syntax Analysis phase in a compiler?
Machine code
Syntax tree
Tokens
Optimized code
Q12
Q12 Which phase is responsible for type checking in a compiler?
Lexical Analysis
Semantic Analysis
Code Generation
Syntax Analysis
Q13
Q13 Which phase deals with improving the intermediate code without changing its functionality?
Code Optimization
Syntax Analysis
Lexical Analysis
Code Generation
Q14
Q14 In which phase are tokens grouped into grammatical phrases?
Lexical Analysis
Syntax Analysis
Code Optimization
Intermediate Code Generation
Q15
Q15 What is the primary purpose of the Code Generation phase in a compiler?
To detect errors
To generate tokens
To create machine code
To construct the syntax tree
Q16
Q16 Which phase performs allocation of registers for program variables?
Lexical Analysis
Syntax Analysis
Semantic Analysis
Code Optimization
Q17
Q17 Which phase of the compiler converts a source program into tokens?
Lexical Analysis
Syntax Analysis
Code Generation
Code Optimization
Q18
Q18 A compiler reports 'undefined variable' during the compilation process. Which phase is responsible for this?
Lexical Analysis
Syntax Analysis
Semantic Analysis
Code Generation
Q19
Q19 What is the main task of the lexical analysis phase in a compiler?
To parse the code
To group characters into tokens
To optimize the code
To generate machine code
Q20
Q20 Which of the following is an output of the lexical analysis phase?
Parse tree
Tokens
Intermediate code
Error messages
Q21
Q21 What is a token in the context of lexical analysis?
A syntax rule
A data structure
A logical unit of the program
A set of machine instructions
Q22
Q22 Which data structure is commonly used in lexical analysis to store tokens?
Linked list
Symbol table
Hash table
Stack
Q23
Q23 What kind of errors are typically detected during lexical analysis?
Syntax errors
Logical errors
Type errors
Invalid tokens
Q24
Q24 What is the role of finite automata in lexical analysis?
To validate syntax rules
To generate parse trees
To recognize patterns
To optimize intermediate code
Q25
Q25 Which regular expression matches valid identifiers in most programming languages?
[a-zA-Z_][a-zA-Z0-9_]
[0-9][a-zA-Z_]
[a-zA-Z][0-9]
[_][a-zA-Z0-9]
Q26
Q26 What is the output of the following regular expression for the input 'abc123': [a-z]+[0-9]+?
abc123
abc
123
abc1
Q27
Q27 Which regular expression matches a valid integer constant?
[0-9]+
[a-zA-Z]+
[0-9a-z]+
[0-9]*
Q28
Q28 Which regular expression will identify valid floating-point numbers?
[0-9]+\.[0-9]+
[a-z]+\.[a-z]+
[0-9][a-z]
[0-9]+[.][a-z]+
Q29
Q29 If the lexical analyzer encounters an unrecognized character, what kind of error is raised?
Syntax error
Logical error
Lexical error
Runtime error
Q30
Q30 What should be the output if the input contains an invalid token?
Compile with warnings
Ignore the token
Raise an error
Continue execution