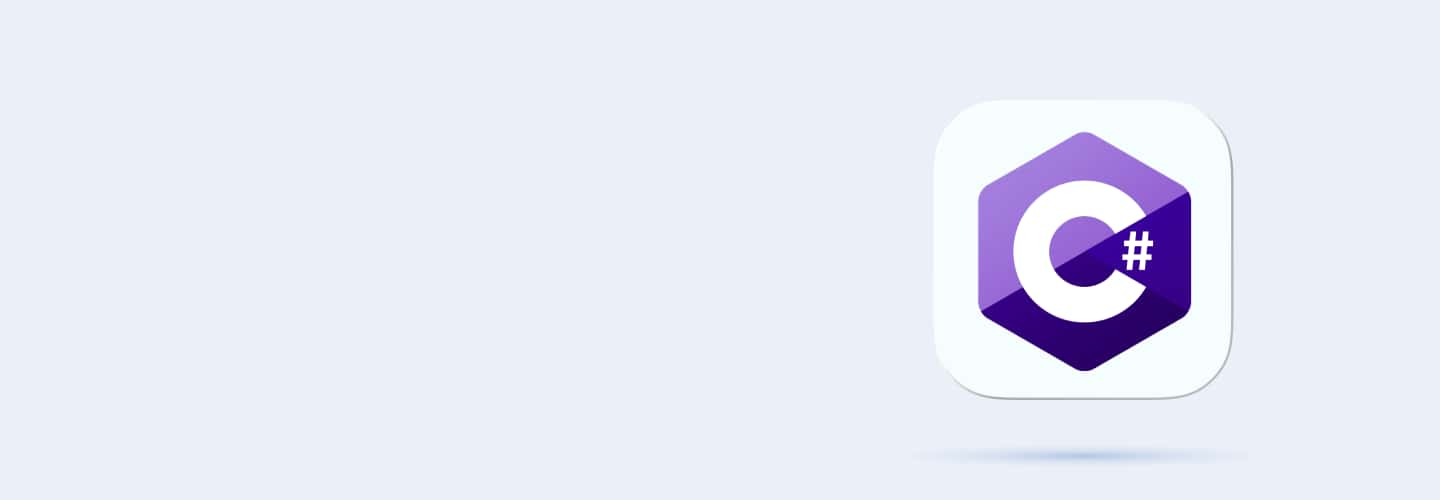
Q121
Q121 What is the purpose of exception handling in C#?
To handle errors and exceptional conditions
To define variables
To create classes
To manage memory
Q122
Q122 Which keyword is used to catch exceptions in C#?
try
catch
throw
finally
Q123
Q123 What does the 'finally' block do in exception handling?
Executes only if an exception is thrown
Executes regardless of an exception
Executes before the catch block
Executes only if no exception is thrown
Q124
Q124 What is the difference between 'throw' and 'throw ex' in C#?
'throw' rethrows the original exception, 'throw ex' throws a new exception
There is no difference
'throw' is used for system exceptions, 'throw ex' is used for application exceptions
'throw' stops the program, 'throw ex' continues the program
Q125
Q125 How do you write a try-catch block in C#?
try { // code } catch (Exception e) { // code }
try { // code } catch { // code }
try catch { // code }
try { // code } finally { // code }
Q126
Q126 How do you throw an exception in C#?
throw new Exception("Error message");
throw Exception("Error message");
throw "Error message";
throw Exception;
Q127
Q127 You get an 'Unhandled Exception' error.
What is the likely cause?
The exception is not caught by any catch block
The exception is caught by the catch block
The finally block is missing
The try block is empty
Q128
Q128 You encounter a 'stack overflow' exception.
What could be the cause?
Infinite recursion
Division by zero
Accessing null object
Array index out of bounds
Q129
Q129 You get a 'NullReferenceException'.
What is the likely cause?
Accessing a method on a null object
Array index out of bounds
Division by zero
Infinite loop
Q130
Q130 What is a delegate in C#?
A type-safe function pointer
A class
An interface
A struct
Q131
Q131 What is the main use of delegates in C#?
To handle exceptions
To allow methods to be passed as parameters
To define classes
To manage memory
Q132
Q132 What is an event in C#?
A method
A delegate that is associated with a specific action
A class
A type of loop
Q133
Q133 How do you declare a delegate in C#?
delegate void MyDelegate(int x);
void delegate MyDelegate(int x);
delegate MyDelegate void(int x);
void MyDelegate delegate(int x);
Q134
Q134 How do you invoke a delegate in C#?
myDelegate.Invoke(10);
Invoke(myDelegate, 10);
call myDelegate(10);
execute myDelegate(10);
Q135
Q135 You get an 'object not set to an instance of an object' error when using a delegate.
What is the likely cause?
Delegate is null
Delegate is already invoked
Delegate is not declared
Delegate is not assigned to an event
Q136
Q136 You encounter an 'event handler not found' error.
What could be the cause?
The event is not defined
The event handler method signature does not match the delegate
The delegate is not instantiated
The event is private
Q137
Q137 What is LINQ in C#?
A method for asynchronous programming
A type-safe function pointer
A query syntax to retrieve data from different sources
A way to handle exceptions
Q138
Q138 What does the 'Select' clause in LINQ do?
Filters elements based on a condition
Projects each element into a new form
Sorts the elements
Groups the elements
Q139
Q139 What is a lambda expression in C#?
A way to define a delegate inline
A type of loop
A method to handle exceptions
A way to declare a class
Q140
Q140 How do you write a lambda expression to add two numbers in C#?
(a, b) => a + b
(a, b) -> a + b
(a, b) { a + b; }
(a, b) +> a + b
Q141
Q141 How do you use LINQ to find the sum of all elements in a list of integers in C#?
list.Sum();
list.Aggregate();
list.Count();
list.Sum((x) => x);
Q142
Q142 You get an 'InvalidOperationException' when using LINQ.
What is the likely cause?
The query is empty
The query has syntax errors
The data source is null
LINQ is not supported
Q143
Q143 You encounter a 'null reference' exception when using a lambda expression.
What could be the cause?
The lambda expression is not assigned
The data source is null
The lambda expression is incorrect
The lambda expression is not defined
Q144
Q144 What is the purpose of the 'FileStream' class in C#?
To handle memory management
To manage database connections
To read from and write to files
To handle network communications
Q145
Q145 What is the difference between 'StreamReader' and 'StreamWriter' in C#?
'StreamReader' is for reading files, 'StreamWriter' is for writing files
'StreamReader' is for binary files, 'StreamWriter' is for text files
'StreamReader' is for small files, 'StreamWriter' is for large files
There is no difference
Q146
Q146 What is the function of the 'File' class in C#?
Provides static methods for file operations
Manages database connections
Handles network communications
Manages memory allocation
Q147
Q147 How do you create a new file in C# using the 'File' class?
File.Create("path");
File.New("path");
File.Make("path");
File.Build("path");
Q148
Q148 How do you read all lines of a text file into a string array in C#?
File.ReadAllLines("path");
File.ReadAllText("path");
File.LoadLines("path");
File.LoadText("path");
Q149
Q149 You get an 'UnauthorizedAccessException' when trying to access a file.
What is the likely cause?
The file is read-only
The file does not exist
The file is open in another application
The file path is incorrect
Q150
Q150 You encounter a 'FileNotFoundException'.
What could be the cause?
The file path is incorrect
The file is corrupted
The file is locked
The file is read-only