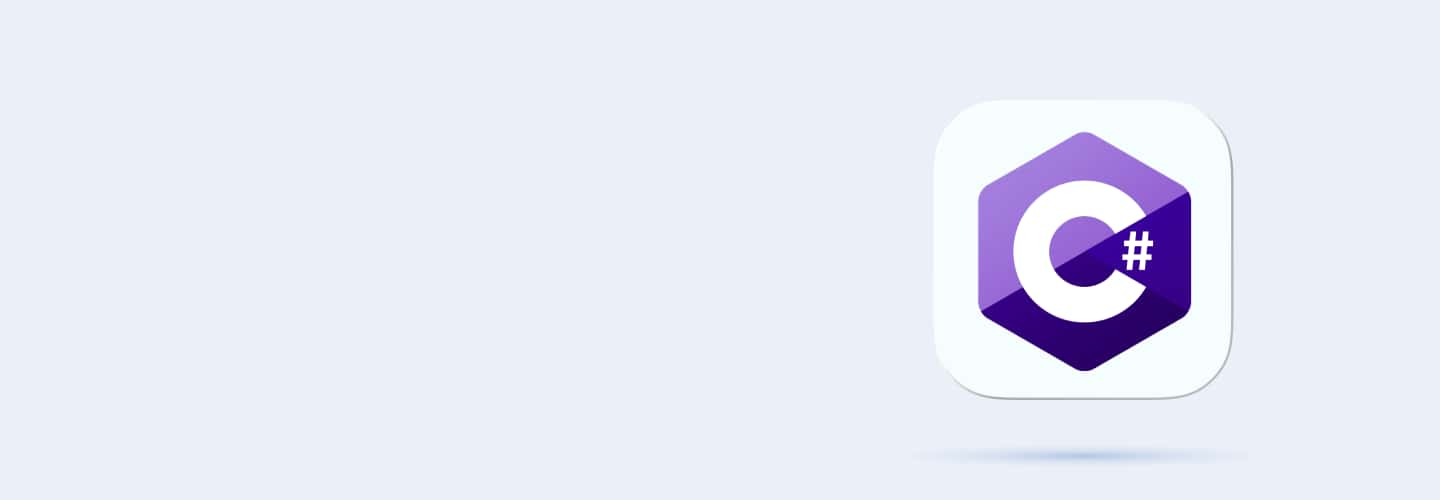
Q91
Q91 What is the purpose of a constructor in a class?
To initialize the object
To destroy the object
To clone the object
To overload methods
Q92
Q92 Which of the following is a correct way to define a parameterized constructor in C#?
public MyClass(int x) { }
public MyClass { int x; }
public void MyClass(int x) { }
public void MyClass { int x; }
Q93
Q93 Which keyword is used to refer to the current instance of the class?
self
this
base
current
Q94
Q94 What is the difference between a field and a property in C#?
Fields are used to store data, properties are used to encapsulate fields
Fields are methods, properties are variables
Fields are public, properties are private
There is no difference
Q95
Q95 What is the purpose of a destructor in a class?
To clean up resources used by the object
To initialize the object
To overload methods
To inherit from another class
Q96
Q96 How do you create an instance of a class named 'Car'?
Car myCar = new Car();
Car myCar();
Car myCar = Car();
new Car myCar;
Q97
Q97 How do you define a property in C#?
public int MyProperty { get; set; }
public int MyProperty { get set; }
public int MyProperty { set; }
public int MyProperty { get; }
Q98
Q98 How do you define a read-only property in C#?
public int MyProperty { get; }
public int MyProperty { set; }
public readonly int MyProperty { get; set; }
public int MyProperty { get; private set; }
Q99
Q99 You get an error 'Constructor not defined'.
What is the likely cause?
No constructor is defined in the class
The constructor is private
The constructor is static
The constructor is virtual
Q100
Q100 You encounter a 'null reference exception' when accessing a class member.
What could be the cause?
The object is not instantiated
The method is not defined
The field is private
The class is abstract
Q101
Q101 You get a 'field initializer cannot reference' error.
What is the likely cause?
Trying to use 'this' keyword in field initializer
Field initializer has syntax error
Using private field in public property
Incorrect use of static keyword
Q102
Q102 What is inheritance in C#?
A way to create new classes from existing classes
A way to encapsulate data
A way to override methods
A way to create interfaces
Q103
Q103 Which keyword is used to inherit a base class in C#?
base
inherit
extends
:
Q104
Q104 What is polymorphism in C#?
The ability to process objects differently based on their data type
The ability to inherit from multiple classes
The ability to encapsulate data
The ability to create new classes
Q105
Q105 What is the purpose of the 'abstract' keyword in C#?
To define methods that must be implemented in derived classes
To create instances of the class
To make the class immutable
To define read-only properties
Q106
Q106 Which of the following is true about method overriding in C#?
The method in the base class must be marked as virtual
The method in the derived class must have the same signature
The method in the derived class must be marked as override
All of the above
Q107
Q107 How do you define an abstract method in C#?
public abstract void MyMethod();
public virtual void MyMethod();
public override void MyMethod();
public void MyMethod();
Q108
Q108 How do you override a method in a derived class in C#?
public override void MyMethod() { // code }
public virtual void MyMethod() { // code }
public void MyMethod() { // code }
public new void MyMethod() { // code }
Q109
Q109 How do you call a base class method from a derived class in C#?
base.MyMethod();
this.MyMethod();
super.MyMethod();
parent.MyMethod();
Q110
Q110 You get an error 'cannot create an instance of the abstract class'.
What is the likely cause?
Trying to instantiate an abstract class
Calling an undefined method
Accessing private members
Incorrect inheritance
Q111
Q111 You encounter a 'method not overridden' warning.
What could be the issue?
The method in the base class is not marked as virtual
The method in the derived class is not marked as override
The method signatures do not match
All of the above
Q112
Q112 What is an interface in C#?
A class with implemented methods
A class with only private methods
A contract with no implementation
A method without a class
Q113
Q113 Which keyword is used to implement an interface in a class?
extends
implements
inherits
:
Q114
Q114 What is the main purpose of an abstract class in C#?
To provide a blueprint for derived classes
To allow multiple inheritance
To store constant values
To implement interfaces
Q115
Q115 Which of the following statements is true about abstract classes in C#?
Abstract classes can be instantiated
Abstract classes cannot contain implemented methods
Abstract classes can inherit from multiple classes
Abstract classes can have constructors
Q116
Q116 What is the difference between an interface and an abstract class in C#?
An interface can have fields, an abstract class cannot
An abstract class can have implementations, an interface cannot
An interface supports multiple inheritance, an abstract class does not
All of the above
Q117
Q117 How do you define an interface in C#?
interface IMyInterface { // code }
public interface IMyInterface { // code }
class IMyInterface { // code }
struct IMyInterface { // code }
Q118
Q118 How do you implement an interface method in a class in C#?
public void InterfaceMethod() { // code }
public void IMyInterface.InterfaceMethod() { // code }
public void InterfaceMethod() override { // code }
public void InterfaceMethod() implements { // code }
Q119
Q119 You get an error 'does not implement interface member'.
What is the likely cause?
The class does not provide implementations for all interface methods
The interface is not defined
The class is abstract
The class does not inherit the interface
Q120
Q120 You encounter an error 'cannot create an instance of an interface'.
What could be the cause?
Trying to instantiate an interface
Implementing the interface incorrectly
Using incorrect syntax
Interface name conflict