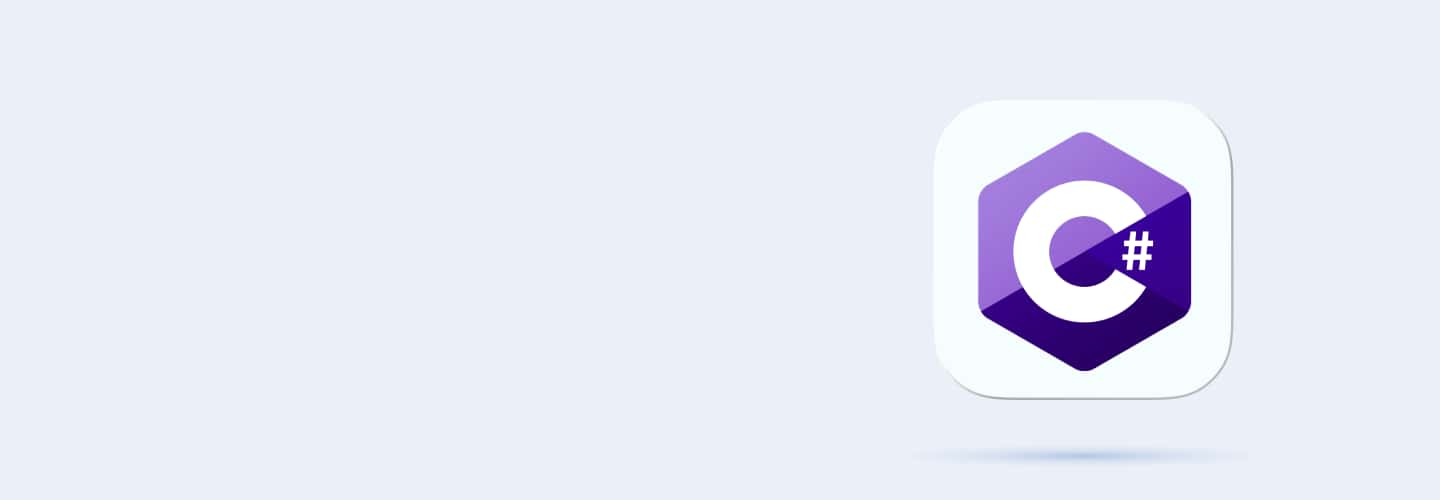
Q61
Q61 How do you initialize an array with values in C#?
int[] arr = {1, 2, 3};
int arr = {1, 2, 3};
int[] arr = new int[] {1, 2, 3};
int arr[] = new int[] {1, 2, 3};
Q62
Q62 How do you declare and initialize a List in C#?
List
List
List
List
Q63
Q63 How do you remove an element from a List by index in C#?
list.RemoveAt(index);
list.Delete(index);
list.Remove(index);
list.Clear(index);
Q64
Q64 How do you sort a List in C#?
list.Sort();
list.Order();
list.Arrange();
list.Align();
Q65
Q65 You get an 'Index out of range' exception.
What is the likely cause?
Accessing array element beyond its bounds
Incorrect data type
Using null value
Syntax error
Q66
Q66 You encounter a 'null reference' exception when accessing a collection.
What could be the cause?
The collection is not initialized
The collection is empty
The collection has invalid elements
The collection is of incorrect type
Q67
Q67 You get an 'ArgumentOutOfRangeException' when trying to access an element in a List.
What could be the issue?
Invalid index value
List is not initialized
List has no elements
Incorrect data type
Q68
Q68 What is a method in C#?
A block of code that performs a task
A variable
A data type
An operator
Q69
Q69 Which keyword is used to return a value from a method in C#?
break
exit
return
end
Q70
Q70 What is the purpose of the 'static' keyword in a method declaration?
Makes the method accessible without an instance
Makes the method private
Makes the method overrideable
None of the above
Q71
Q71 What is an overloaded method in C#?
A method with the same name but different parameters
A method that returns different types
A method that is static
A method that is virtual
Q72
Q72 Which of the following is true about method overriding in C#?
Overriding requires the 'override' keyword
Overriding is the same as overloading
Overriding methods must have different names
None of the above
Q73
Q73 How do you define a method in C#?
void MethodName() { // code }
method MethodName() { // code }
void MethodName[] { // code }
function MethodName() { // code }
Q74
Q74 How do you call a method named 'Display' in C#?
Display();
call Display();
invoke Display();
execute Display();
Q75
Q75 How do you define a method that takes an integer parameter in C#?
void MethodName(int x) { // code }
void MethodName(x int) { // code }
void MethodName(int: x) { // code }
void MethodName(int) { // code }
Q76
Q76 How do you define a method that returns an integer value in C#?
int MethodName() { return 0; }
integer MethodName() { return 0; }
void MethodName() { return 0; }
int MethodName() { }
Q77
Q77 You get a 'missing return statement' error.
What is the likely cause?
The method does not return a value
The method has no parameters
The method is static
The method is private
Q78
Q78 You encounter a 'method not found' error.
What could be the cause?
Misspelled method name
Incorrect method parameters
Method is private
All of the above
Q79
Q79 What is a class in C#?
A blueprint for creating objects
A variable
A data type
A method
Q80
Q80 Which keyword is used to inherit a class in C#?
inherit
base
extends
:
Q81
Q81 What is encapsulation in C#?
Hiding the implementation details
Ability to inherit from another class
Writing reusable code
All of the above
Q82
Q82 Which of the following supports method overloading in C#?
Polymorphism
Encapsulation
Abstraction
Inheritance
Q83
Q83 What is the purpose of the 'virtual' keyword in a method declaration in C#?
To allow the method to be overridden in a derived class
To make the method static
To prevent the method from being overridden
None of the above
Q84
Q84 How do you define a class in C#?
class MyClass { // code }
MyClass class { // code }
define class MyClass { // code }
class: MyClass { // code }
Q85
Q85 How do you create an object of a class in C#?
MyClass obj = new MyClass();
MyClass obj();
MyClass obj = MyClass();
new MyClass obj;
Q86
Q86 How do you define a method in a class that can be overridden in C#?
public virtual void MyMethod() { // code }
public override void MyMethod() { // code }
public void MyMethod() { // code }
public static void MyMethod() { // code }
Q87
Q87 You get a 'cannot create an instance of the abstract class' error.
What is the likely cause?
Trying to instantiate an abstract class
Calling an undefined method
Accessing private members
Incorrect inheritance
Q88
Q88 You encounter a 'method not overridden' warning.
What could be the issue?
The method in the base class is not marked as virtual
The method in the derived class is not marked as override
The method signatures do not match
All of the above
Q89
Q89 You get a 'object reference not set to an instance of an object' error.
What is the most likely cause?
Accessing a member of a null object
Incorrect method parameters
Invalid cast
Syntax error
Q90
Q90 What is an object in C#?
An instance of a class
A blueprint of a class
A variable
A method