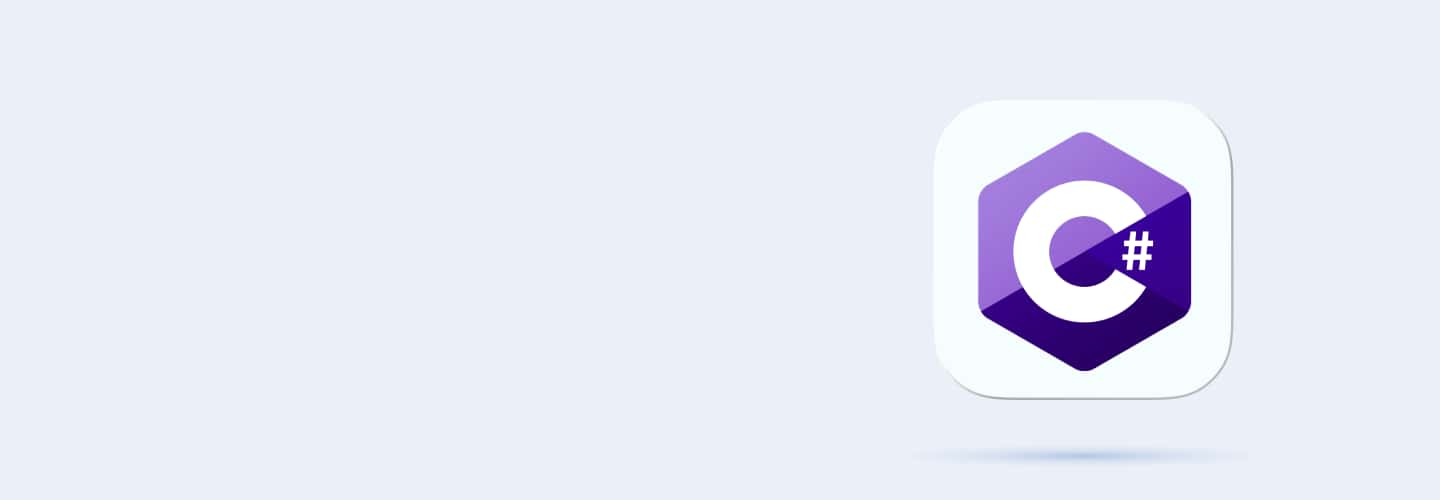
Q31
Q31 How do you define an array of integers in C#?
int[] numbers = new int[5];
int numbers = new int[5];
int[5] numbers;
int array[5];
Q32
Q32 You get an 'unassigned local variable' error.
What is the likely cause?
Variable declared but not initialized
Incorrect data type
Using a reserved keyword
Incorrect syntax
Q33
Q33 You get a 'cannot implicitly convert type' error.
What might be the cause?
Trying to assign a float to an int
Missing semicolon
Incorrect method definition
Using a reserved keyword
Q34
Q34 What does the '==' operator do in C#?
Compares values for equality
Assigns value
Compares references
None of the above
Q35
Q35 Which operator is used to concatenate strings in C#?
=+
&
*
&&
Q36
Q36 What is the result of '10 / 3' in C#?
3.33
3
4
3.0
Q37
Q37 What does the '&&' operator do in C#?
Logical AND
Logical OR
Bitwise AND
Bitwise OR
Q38
Q38 What is the output of '5 + 2 * 3' in C#?
21
11
10
7
Q39
Q39 How do you increment a variable 'x' by 1 in C#?
x = x + 1;
x += 1;
x++;
All of the above
Q40
Q40 What is the result of '15 % 4' in C#?
3
4
1
2
Q41
Q41 How do you write a conditional expression that checks if 'x' is greater than 'y' in C#?
if (x > y) { }
if x > y then { }
if (x > y) then { }
if x > y { }
Q42
Q42 You get a 'Division by zero' runtime error.
What is the likely cause?
Dividing an integer by zero
Multiplying two large integers
Using uninitialized variables
Accessing array elements out of bounds
Q43
Q43 You get an 'invalid expression' error when using operators.
What could be the issue?
Missing operand
Using reserved keywords
Incorrect syntax
All of the above
Q44
Q44 What might cause a 'type mismatch' error in an expression?
Mixing data types in an expression
Using incorrect variable names
Incorrect function call
Syntax errors
Q45
Q45 Which keyword is used to exit a loop prematurely in C#?
exit
stop
break
return
Q46
Q46 Which of the following is a conditional statement in C#?
for
while
if
do
Q47
Q47 What is the purpose of the 'continue' keyword in loops?
Exit the loop
Skip the current iteration and continue with the next
End the program
None of the above
Q48
Q48 Which loop is guaranteed to execute at least once?
for
while
do-while
foreach
Q49
Q49 How do you define a switch statement in C#?
switch (variable) { case value: break; }
switch (variable) { case value; break; }
switch variable { case value: break; }
switch variable { case value; break; }
Q50
Q50 How do you write an 'if-else' statement in C#?
if (condition) { // code } else { // code }
if condition: { // code } else: { // code }
if condition then { // code } else then { // code }
if (condition) { // code } otherwise { // code }
Q51
Q51 How do you write a 'for' loop in C#?
for (int i = 0; i < 10; i++) { }
for (int i = 0; i < 10) { }
for i in range(10): { }
for i = 0 to 9 { }
Q52
Q52 How do you write a 'while' loop in C#?
while (condition) { // code }
while condition: { // code }
while (condition) then { // code }
while condition { // code }
Q53
Q53 Your 'if' statement always executes the 'else' part.
What could be the issue?
Incorrect condition
Missing braces
Variable scope issue
Using 'else if' instead of 'else'
Q54
Q54 You encounter an infinite loop in your code.
What is the most likely cause?
Incorrect loop condition
Missing return statement
Variable scope issue
Syntax error
Q55
Q55 You get a 'case label not within a switch statement' error.
What might be the cause?
Case label used outside switch statement
Incorrect case value
Missing break statement
Incorrect syntax
Q56
Q56 What is the correct way to declare a single-dimensional array in C#?
int[] arr;
int arr[];
int[10] arr;
int arr;
Q57
Q57 What is the index of the first element in a C# array?
0
1
-1
It depends on the array size
Q58
Q58 Which collection type is ordered and allows duplicate elements?
Dictionary
HashSet
List
Queue
Q59
Q59 What method is used to add an element to a List in C#?
Add()
Insert()
Append()
Push()
Q60
Q60 Which of the following is true about arrays and collections in C#?
Arrays are dynamic, collections are static
Arrays can store elements of different types, collections cannot
Collections provide more flexibility and functionality than arrays
Arrays are always passed by reference